Testing is a critical aspect of software unit testing, ensuring applications function as expected and meet user needs.
So why leave testing as an afterthought when it can drive your entire development process?
Among the many software testing tools, NUnit stands out as one of the most trusted unit testing frameworks for .NET applications. With its intuitive syntax, powerful features, and seamless integration with modern development environments, NUnit is a favorite for developers working on agile methodologies or extreme programming practices.
On the other hand, Test-Driven Development (TDD) is a software development technique that places testing at the forefront of the code development cycle. But what does TDD mean, practically? It’s an approach where tests are written before production code, helping developers align with business requirements, reduce technical debt, and produce reliable code.
In this blog, we’ll explore how the NUnit framework complements the principles of test-driven development, helping agile development teams deliver high-quality software through better design, cleaner tests, and reduced debugging efforts.
Why this blog is a must-read: 📘
- Understand TDD Basics: Learn what TDD means, its principles, and how it ensures better software unit testing.
- Explore NUnit Framework: Discover why NUnit is a preferred choice among unit testing tools for .NET applications.
- Master Writing Tests: Gain insights into writing effective and maintainable NUnit testing test cases.
- Unlock Advanced NUnit Features: Dive into data-driven testing, parameterized tests, and mocking dependencies.
- Adopt TDD Best Practices: Avoid common pitfalls, refactor using feedback, and achieve comprehensive test coverage.
Key Principles of Test-Driven Development (TDD)
The foundation of what is TDD lies in its simple yet effective principles that enforce disciplined coding and testing practices. By adhering to these principles, developers create maintainable, testable code that evolves gracefully alongside user requirements.
This approach is particularly beneficial in types of software testing, such as unit and integration testing, ensuring clarity and quality in every iteration.

Red-Green-Refactor Cycle
At the heart of TDD frameworks like NUnit lies the Red-Green-Refactor cycle, a systematic process that ensures every new feature or bug fix is implemented with clarity and precision. Here’s how the cycle works:
- Red Phase: Write a test for the functionality you’re about to implement. Due to the lack of the feature, this test will initially fail. For example, in a calculator application, you might write a test for the Add method, expecting it to return the sum of two numbers.
- Green Phase: Write the minimum code required to make the test pass. The goal here is to implement the piece of functionality without worrying about perfection.
- Refactor Phase: Once the test passes, revisit the code and improve its structure. This includes eliminating code duplication, adhering to design patterns, and ensuring testable code.
The Red-Green-Refactor cycle ensures every piece of functionality in your software testing is implemented with purpose and precision, promoting better unit testing types and practices.
Writing Tests Before Code for Better Design
Writing tests before production code is a hallmark of test-driven development. This approach forces developers to focus on core functionality, defining the desired behavior of the code in advance.
For instance, before implementing a string version of the GetText method, you would first define tests for its expected behavior. These tests act as a guide, ensuring the final implementation meets the intended design. This approach, often referred to as the blue phase, creates clarity in the development process and aligns with software testing tools like NUnit.

For example, before implementing a string version of a method like GetText, you might first define tests for its expected behavior. By doing this, you create a blue phase of clarity where the design requirements are explicitly defined.
Benefits of TDD in Agile Development
TDD fits perfectly within the agile framework, supporting agile teams in building better software. Here’s how:
- Better Collaboration: TDD fosters collaboration among team members, including business analysts, testers, and developers. By writing tests upfront, everyone gains a shared understanding of the expected outcomes.
- Cleaner Code Over Time: Following TDD principles results in code over time that is easier to maintain and extend.
- Faster Debugging: Since tests are written first, bugs in the actual code are detected earlier, reducing debugging time significantly.
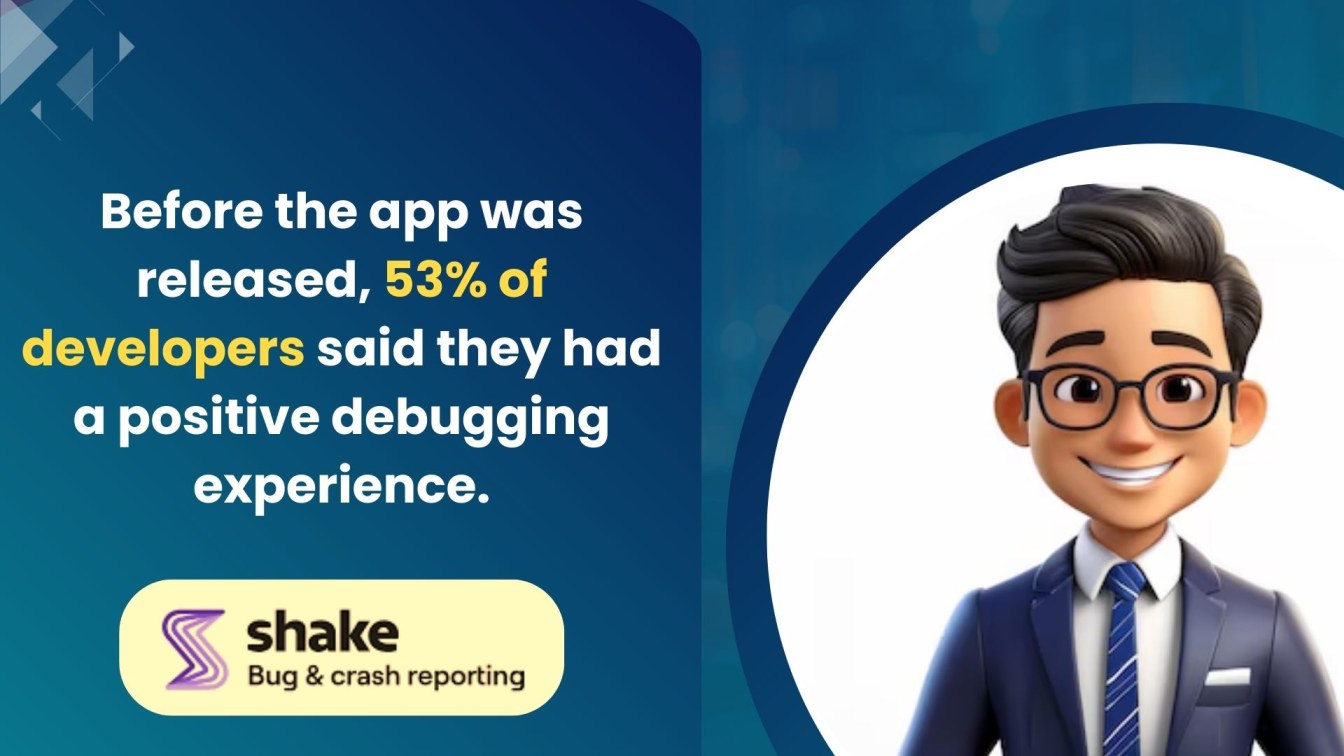
- Reduced Technical Debt: TDD enforces an iterative process where unnecessary or boilerplate code is minimized, keeping the code base clean and efficient.
Agile development thrives on continuous improvement, and TDD aligns perfectly with this philosophy.
Writing Effective NUnit Test Cases
Crafting effective NUnit test cases is essential to ensure a cleaner code base, maintain quality, and detect issues early in the software development process. With its simple syntax and versatile capabilities, NUnit helps developers create well-structured tests that validate core functionality, detect code duplication, and ensure alignment with business requirements.
In this section, we'll discuss best practices for structuring tests, using assertions, and testing edge cases to enhance the development process.
Structuring Test Cases for Clarity and Maintainability
A well-organized test structure is the foundation of maintainable and scalable testing. Agile development teams benefit from writing tests that are easy to read, understand, and update as the software design evolves. Here’s how you can structure NUnit test cases effectively:
- Use Descriptive Names:
Clear descriptions of the scenario being examined should be included in test names. Avoid generic names like Test1 or UnitTest—opt for descriptive ones like Test_Addition_With_Valid_Inputs to indicate the piece of functionality being verified. - Group Tests by Functionality:
Organize tests within public classes. For instance, tests for a calculator application can be grouped under CalculatorTests to provide clarity and context. - Follow the AAA Pattern:
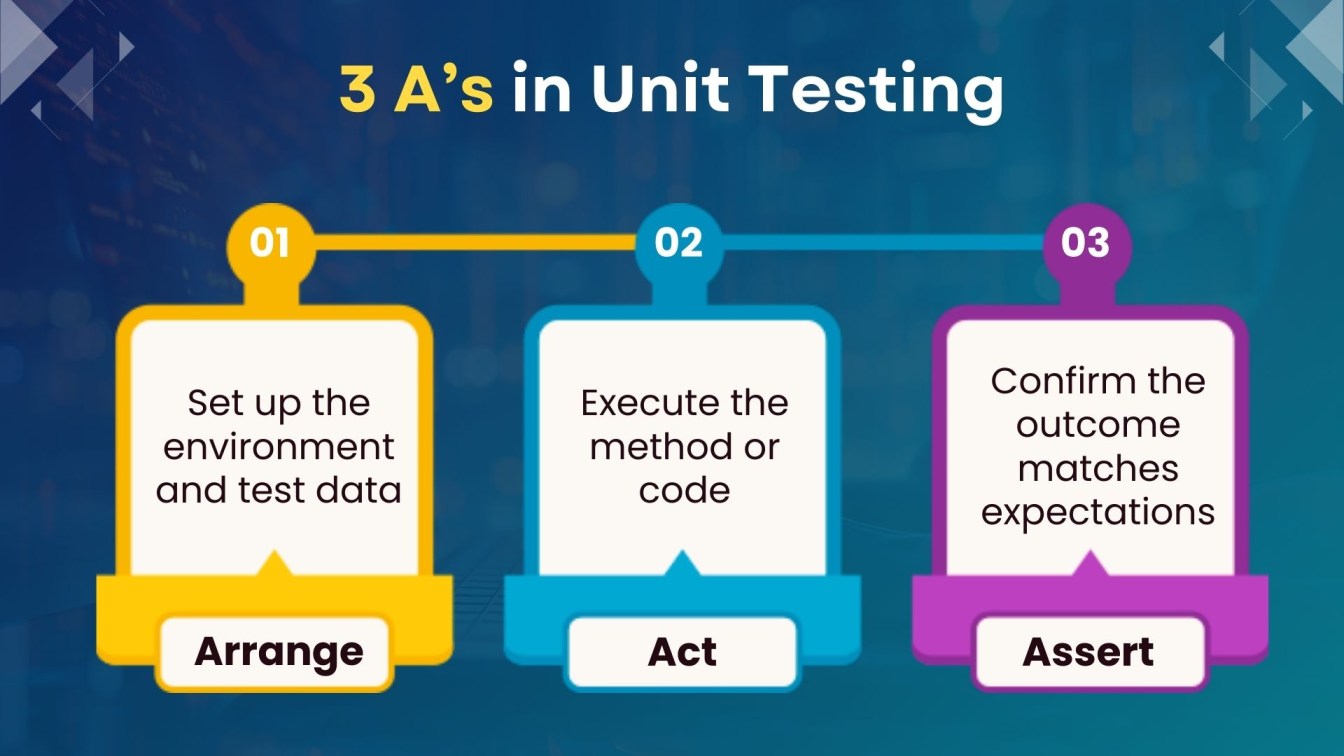
Structure test methods in three distinct phases:
- Arrange: Configure the environment and test data as needed.
- Act: Call the method or unit of code being tested.
- Assert: Verify the outcome matches expectations

Structuring test cases this way ensures they remain easy to maintain, even in complex projects.
Using Assertions for Accurate Validations
Assertions are the core of any test case, providing the mechanism to verify that the actual code behaves as expected. NUnit offers a variety of assertion methods to cover diverse scenarios.
- Common Assertions:
- Assert.AreEqual(expected, actual): Verifies equality.
- Assert.IsTrue(condition): Ensures a condition is true.
- Assert.Throws<ExceptionType>(method): Confirms that an exception is thrown as expected.
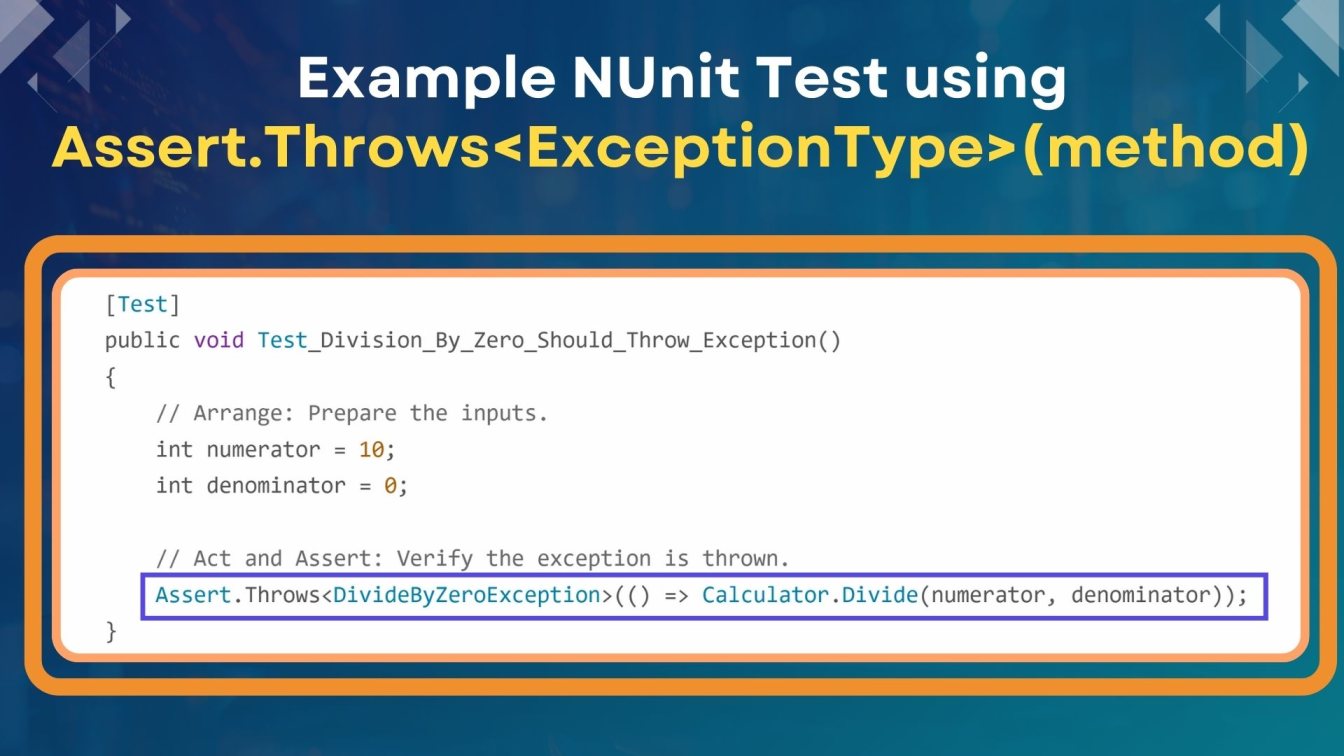
Comment: This test ensures that dividing by zero throws the appropriate exception, validating the application's handling of errors.
Assertions help confirm that every code unit adheres to its expected behavior, enabling agile processes to maintain high standards of code quality.
Testing Edge Cases and Boundary Conditions
While testing common scenarios is essential, agile methodologies emphasize the importance of covering edge cases and boundary conditions. These tests ensure the current functionality performs correctly in unusual or extreme situations, minimizing unexpected failures in production.
Examples of Edge Cases:
- Handling empty or null inputs.
- Verifying behavior with maximum or minimum values.
- Testing for unexpected or invalid inputs.
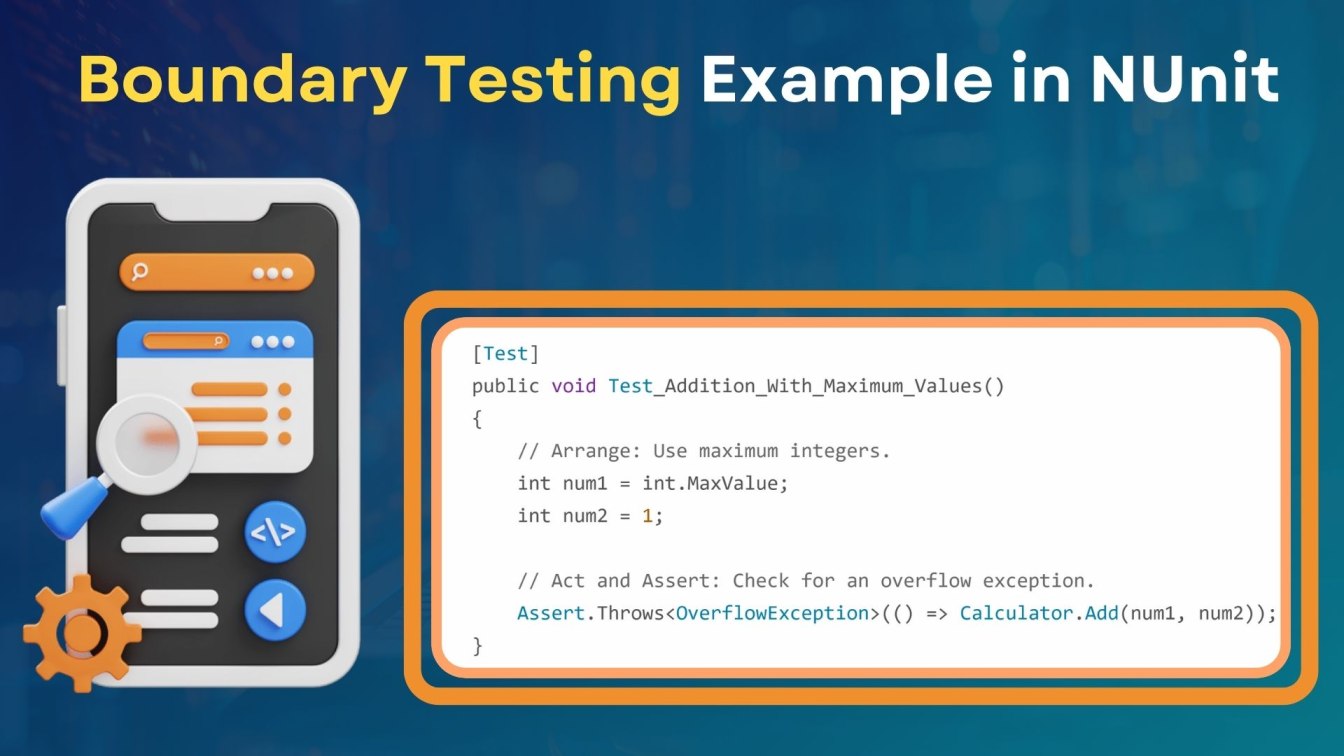
This test ensures the application gracefully handles scenarios where input values exceed acceptable limits.
Testing edge cases helps ensure correct behavior across a wide range of conditions, reducing debugging time and improving the software design.

Advanced Features of NUnit
NUnit’s versatility extends beyond basic testing. Its advanced features simplify testing in complex projects by reducing code duplication, improving efficiency, and enabling developers to test a variety of scenarios effortlessly.
Below, we explore parameterized tests, data-driven testing, and mocking dependencies, all of which play a crucial role in modern development environments.
Parameterized Tests for Flexibility
Parameterized tests allow developers to execute the same test logic with multiple inputs, reducing redundancy and ensuring comprehensive validation.
This is especially beneficial in applications requiring testing across combinations, such as cross-browser testing or multiple operating systems.
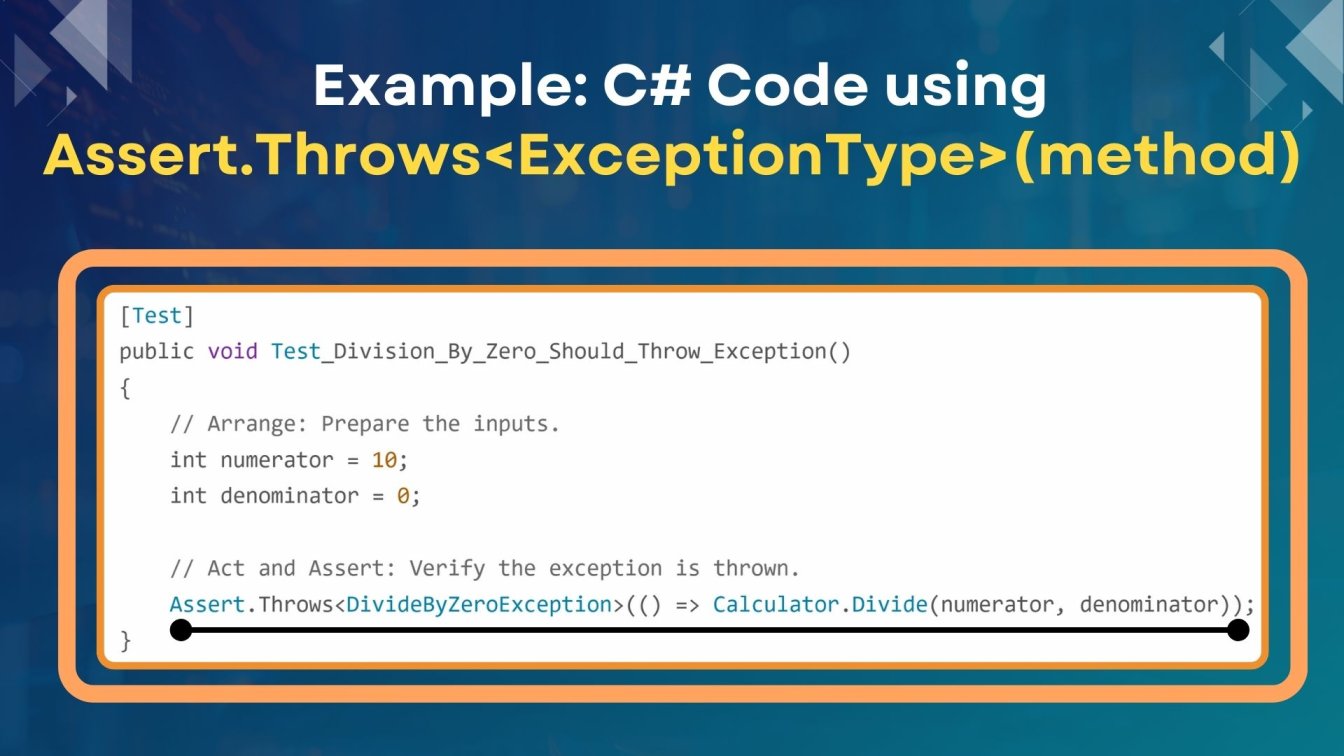
Comment: Each [TestCase] defines a unique input-output combination, enabling a reliable code-testing process without duplicating logic.
Parameterized tests improve the efficiency of testing across scenarios, ensuring minimum code is written for maximum coverage.
Data-Driven Testing with Test Fixtures
When testing requires a larger set of data or diverse configurations, test fixtures provide a structured way to manage them. Fixtures group-related tests under a common setup, reducing repetitive code and simplifying test management.
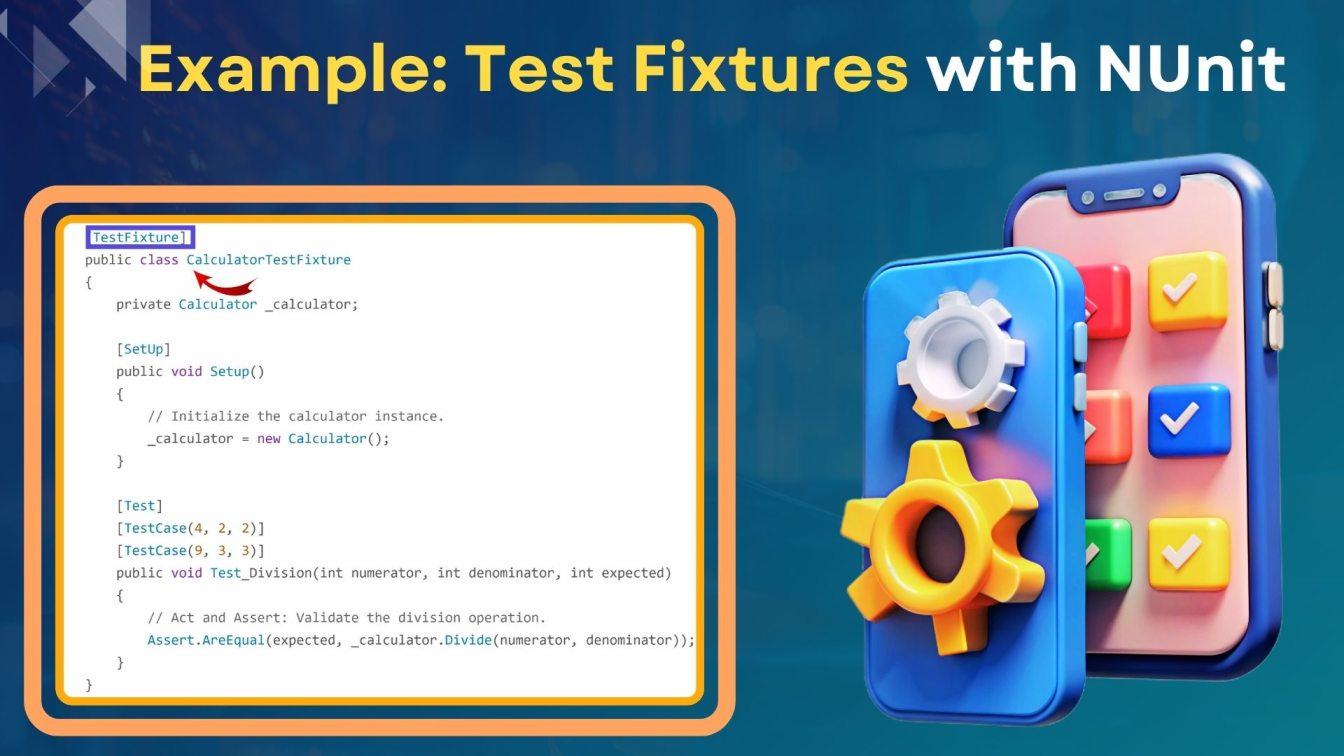
Comment: The [SetUp] attribute initializes resources before every test, ensuring consistency and avoiding repeated code.
Data-driven testing is invaluable for agile teams working on cross-functional projects or applications with diverse input scenarios.
Mocking Dependencies for Effective Unit Testing
In complex projects, testing individual components often requires isolating them from external dependencies such as databases, APIs, or third-party services. Mocking enables developers to simulate these dependencies, focusing solely on the core functionality of the application code.
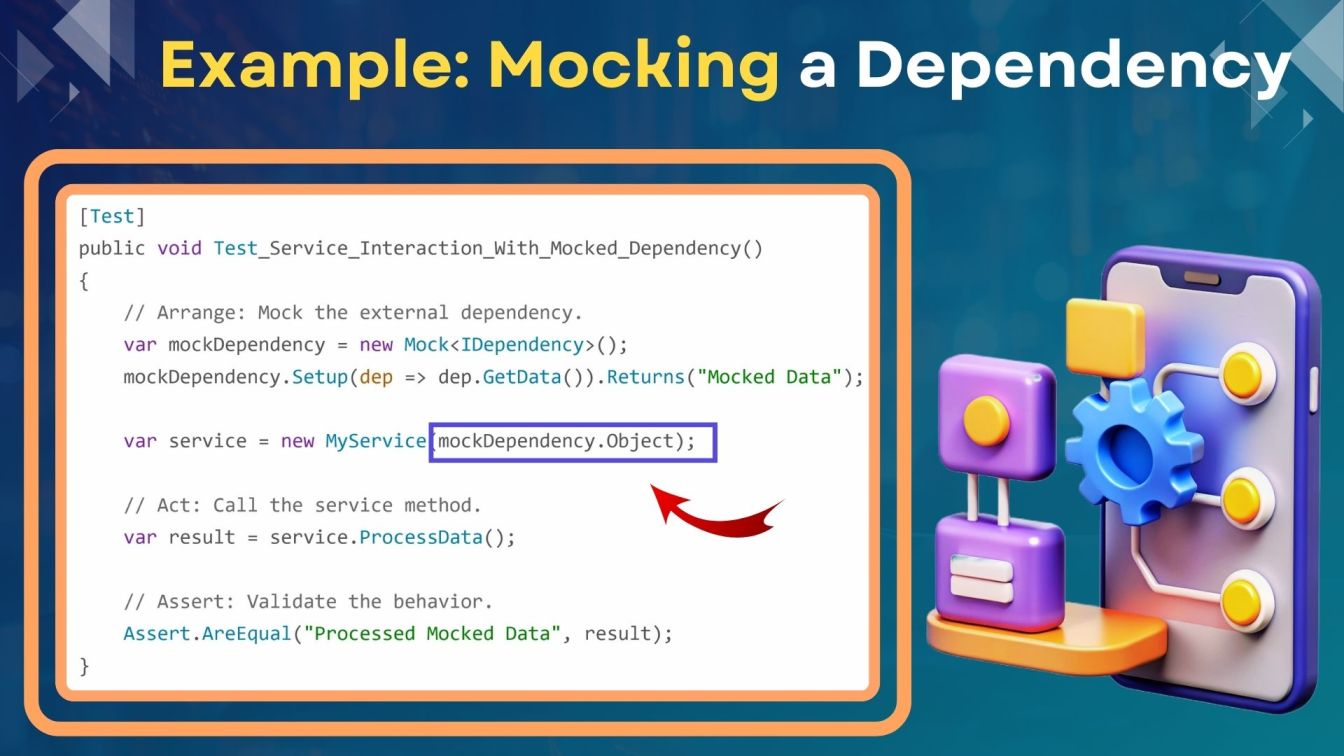
Comment: This test isolates the service by mocking the dependency, ensuring that the test focuses on the service’s logic.
Mocking is an essential practice in unit testing frameworks, especially when dealing with external behavior or dependencies. It ensures tests remain reliable and maintainable, even in complex projects.

Best Practices for TDD with NUnit
Test-Driven Development (TDD) is a development technique that helps developers write cleaner code, maintain high code quality, and reduce technical debt over time. When paired with NUnit, one of the most popular unit testing frameworks, TDD becomes an effective tool to ensure that the software development process is reliable and adaptable to evolving project requirements.
By following certain best practices, development teams can avoid common pitfalls and achieve a balance between testing and implementation. In this section, we’ll explore ways to refine your TDD approach using NUnit, focusing on avoiding mistakes, utilizing feedback from test results, and ensuring comprehensive test coverage.
Avoiding Common Mistakes in TDD
TDD can be transformative for agile development teams, but it requires discipline and the avoidance of certain common mistakes that can derail its benefits. Below are some frequent issues and strategies to overcome them:
- Writing Tests Without Clear Requirements:
TDD is rooted in understanding business requirements and translating them into code units. Writing tests without fully grasping user requirements or the intended behavior leads to unnecessary or irrelevant tests. Collaborate with business analysts to define acceptance tests for each feature. - Overlooking Edge Cases and Negative Scenarios:
Many teams focus solely on happy path tests, ignoring boundary conditions and invalid inputs. A comprehensive suite of tests should include these scenarios to prevent bugs in real code. - Skipping Refactoring After Passing Tests:
The red-green-refactor cycle encourages iterative improvements, but some developers stop at the "green" phase. Always refactor production code to eliminate code duplication and improve clarity without altering behavior. - Relying Solely on Unit Tests:
TDD prioritizes unit tests, but these should be complemented with integration and acceptance testing to ensure the entire system functions correctly. Tools like Selenium can assist in broader tests like cross-browser testing.
By avoiding these mistakes, developers can fully embrace TDD and make their testing practices more effective.
Refactoring Code Using NUnit Feedback
Refactoring is an integral part of TDD that ensures the code remains maintainable and free of basic code smells. Because it continuously provides input on how changes affect the system, NUnit plays a vital function. Here’s how to use NUnit feedback effectively during refactoring:
- Start with Passing Tests:
Before refactoring, ensure all existing tests are passing. This establishes a baseline for current functionality and ensures that future changes don’t break the system. - Refactor in Small Steps:
Break down refactoring into manageable steps. For example:- Simplify complex methods into smaller helper functions.
- Replace repetitive code with reusable methods.
- Use dependency injection to reduce coupling.
- Use Tests as a Safety Net:
As you refactor, run your NUnit tests frequently. Any failing test indicates a deviation from the expected behavior, allowing you to address issues before they escalate. - Eliminate Boilerplate Code:
Refactor to remove unnecessary boilerplate code and focus on the minimum code required for functionality.
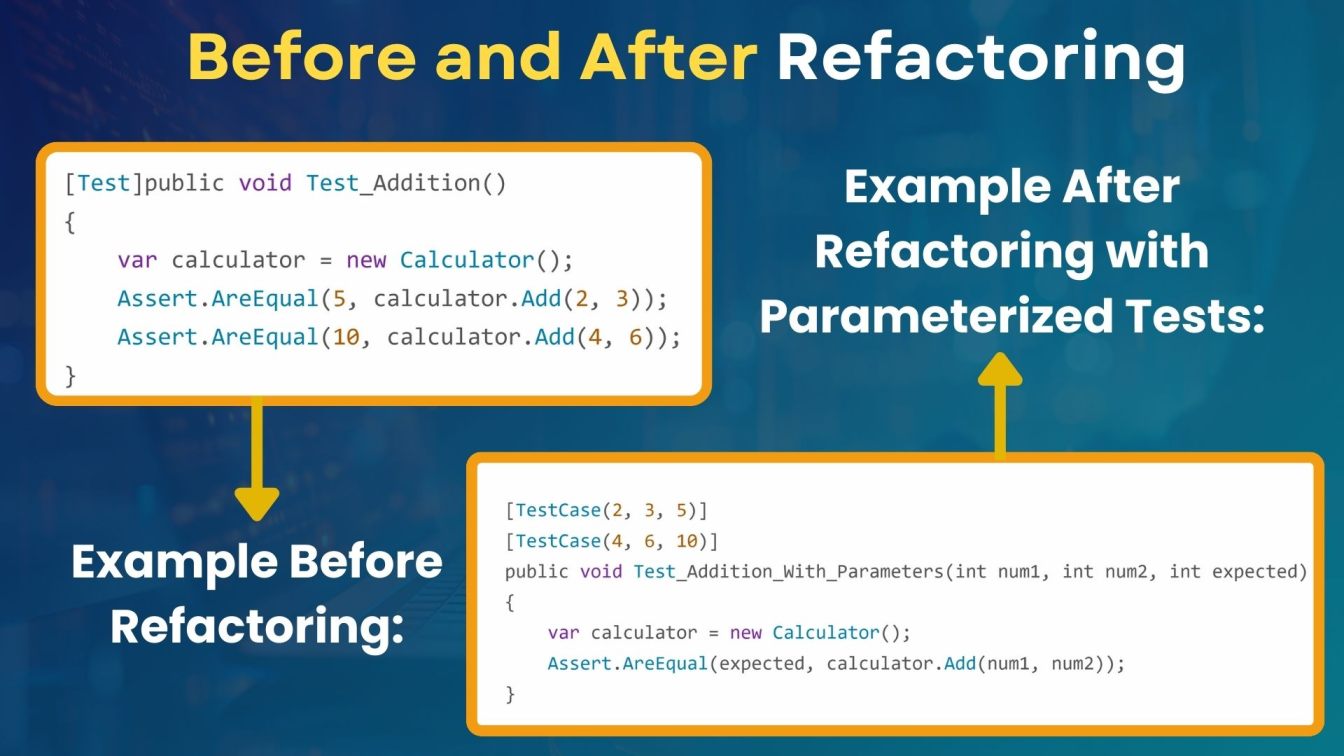
- The refactored version reduces code duplication and enhances readability while maintaining the same test coverage.
- Address Code Smells:
Refactor to remove basic code smells like long methods, large classes, and unclear naming conventions. This ensures the code structure remains clean and easy to understand.
Refactoring with NUnit feedback ensures that your application code evolves without compromising its integrity or performance.
Ensuring Comprehensive Test Coverage
Comprehensive test coverage is critical to ensuring that every piece of functionality in the application is tested. Without it, you risk leaving bugs undetected in core functionality or external dependencies. Below are strategies to maximize test coverage with NUnit:
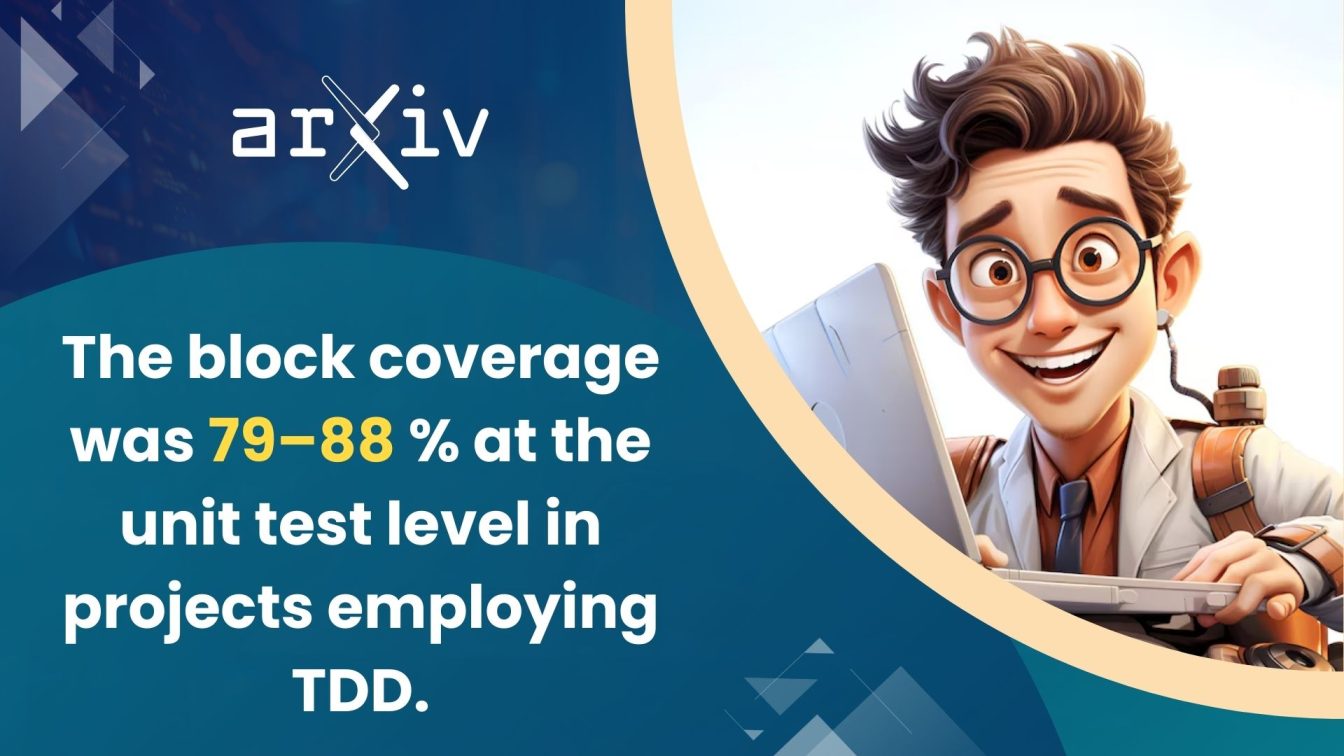
- Cover All Functional Requirements:
Start by ensuring that every business requirement is translated into one or more test cases. Collaborate with agile teams to define the scope of testing for each feature. - Test Across Different Scenarios:
Ensure that your tests cover a wide range of inputs and outputs, including:- Valid inputs (normal cases).
- Invalid inputs (error handling).
- Edge cases (e.g., maximum or minimum values).
- Boundary conditions.
- Incorporate Data-Driven Testing:
Use NUnit’s data-driven testing features, such as [TestCase] and [TestFixture], to efficiently test multiple scenarios without duplicating code
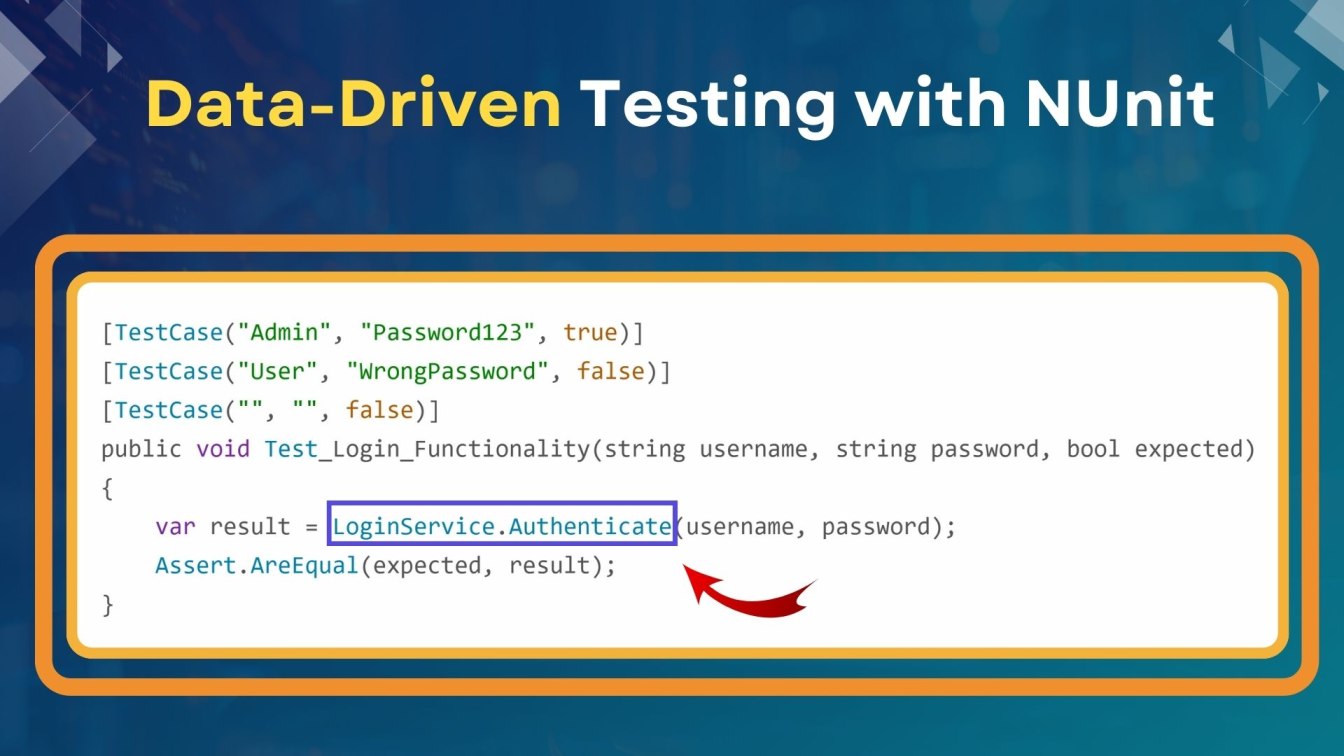
- Measure Code Coverage:
Use tools like Visual Studio’s built-in code coverage tools to identify untested areas in the code. These tools provide a visual representation of which lines of code are covered by your tests, helping to improve coverage. - Include Edge Cases in Coverage Metrics:
Edge cases and boundary conditions often fall outside typical scenarios but are critical for preventing unexpected behavior in production. - Validate Against Acceptance Tests:
Acceptance tests ensure that the application meets user requirements and behaves as expected in real-world scenarios. NUnit can be integrated with continuous integration pipelines to run acceptance tests automatically after every change.
Example Integration:- Set up NUnit tests in a unit test project.
- Configure the project in a continuous integration and deployment (CI/CD) pipeline using tools like Jenkins or Azure DevOps.
Comprehensive test coverage ensures that every code unit is validated, giving development teams confidence in the reliability of their application code.
Conclusion
TDD, when combined with NUnit, offers a powerful approach to building maintainable, high-quality software. By avoiding common mistakes, leveraging feedback for refactoring, and focusing on comprehensive test coverage, developers can achieve cleaner tests, minimize technical debt, and meet evolving business requirements effectively.
NUnit’s features and flexibility make it an invaluable tool for modern development teams, enabling them to create testable code that adapts to agile methodologies and ensures a seamless development process. Following these best practices will not only enhance your testing capabilities but also lead to better collaboration among cross-functional teams, ultimately improving the overall software development methodology. 🚀
People Also Ask
Is TDD basically Unit Testing?
No, TDD is a development approach where tests drive code creation, while unit testing is a technique to validate individual pieces of code.
Which is better, NUnit or XUnit?
Both are excellent unit testing frameworks, but NUnit offers more legacy support, while XUnit is modern and better suited for dependency injection.
What is the difference between TDD and BDD?
TDD focuses on testing code behavior from a developer's perspective, whereas BDD emphasizes collaboration and tests based on user behavior and requirements.
What do we use TestNG and what is it?
TestNG is a testing framework for Java, used for its powerful features like annotations, data-driven testing, and better test configuration.
What comes after TDD?
After TDD, teams often perform integration testing, acceptance testing, and continuous integration to ensure the system works as a whole.