Code coverage in software testing measures the percentage of your source code executed during automated tests. It helps ensure that all critical code paths are tested, improving software quality by identifying untested areas. Common code coverage tools like JaCoCo for Java, Istanbul (nyc) for JavaScript/Node.js, and Coverage.py for Python generate detailed code coverage reports, highlighting gaps in tests. Achieving high code coverage doesn't guarantee bug-free software, but it contributes significantly to better unit testing, quality software, and efficient CI/CD processes.
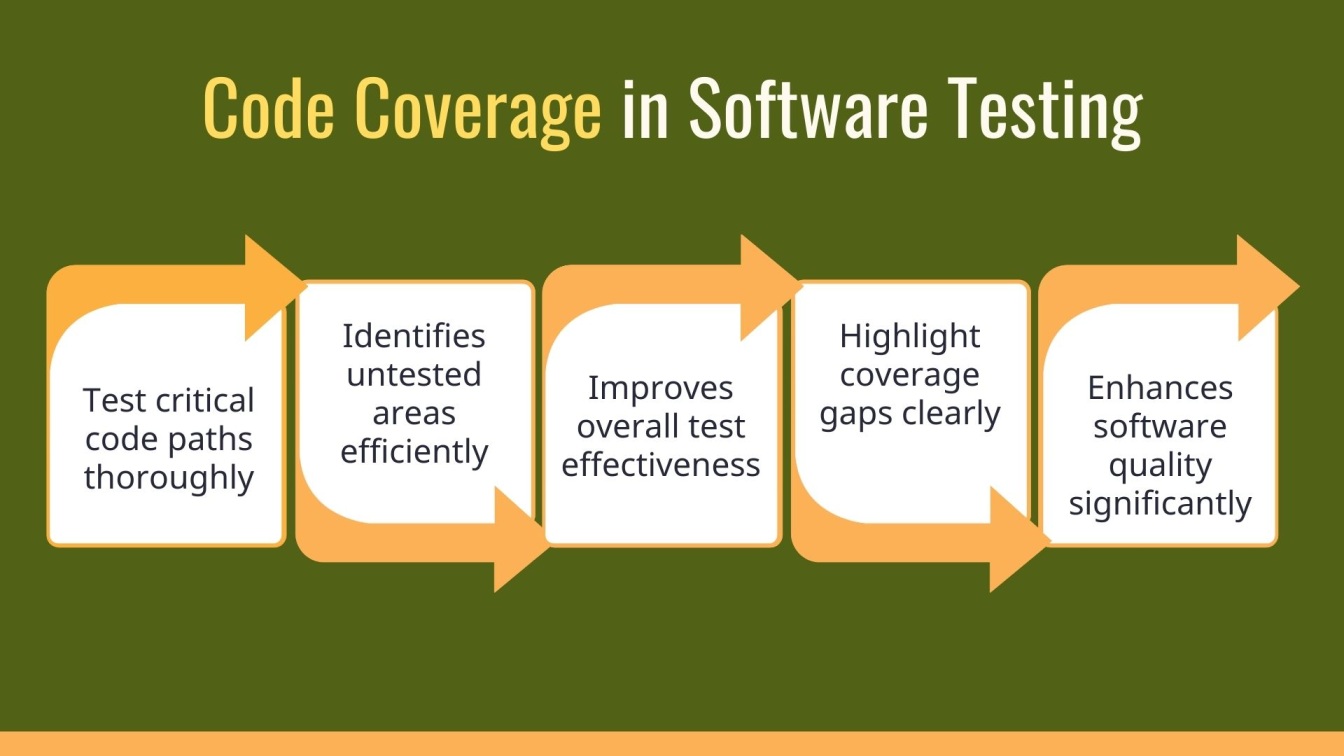
What’s next? Keep reading to discover:
🚀 Why It Matters – Enhancing software quality and testing effectiveness.
🚀 Top Tools – Best tools like JaCoCo, Istanbul, and Coverage.py for accurate measurement.
🚀 Code Coverage Metrics – Key metrics: line, branch, and function coverage.
🚀 Common Challenges – Issues like false security and test maintenance concerns.
🚀 Best Practices – Proven strategies to optimize coverage and improve testing.
Why Code Coverage Is Crucial for Software Quality
Code coverage plays a vital role in maintaining and improving software quality by ensuring thorough testing of the codebase. It helps in identifying areas of code that have not been executed during testing, ensuring that the tests are thorough and effective. Here's why it’s crucial:
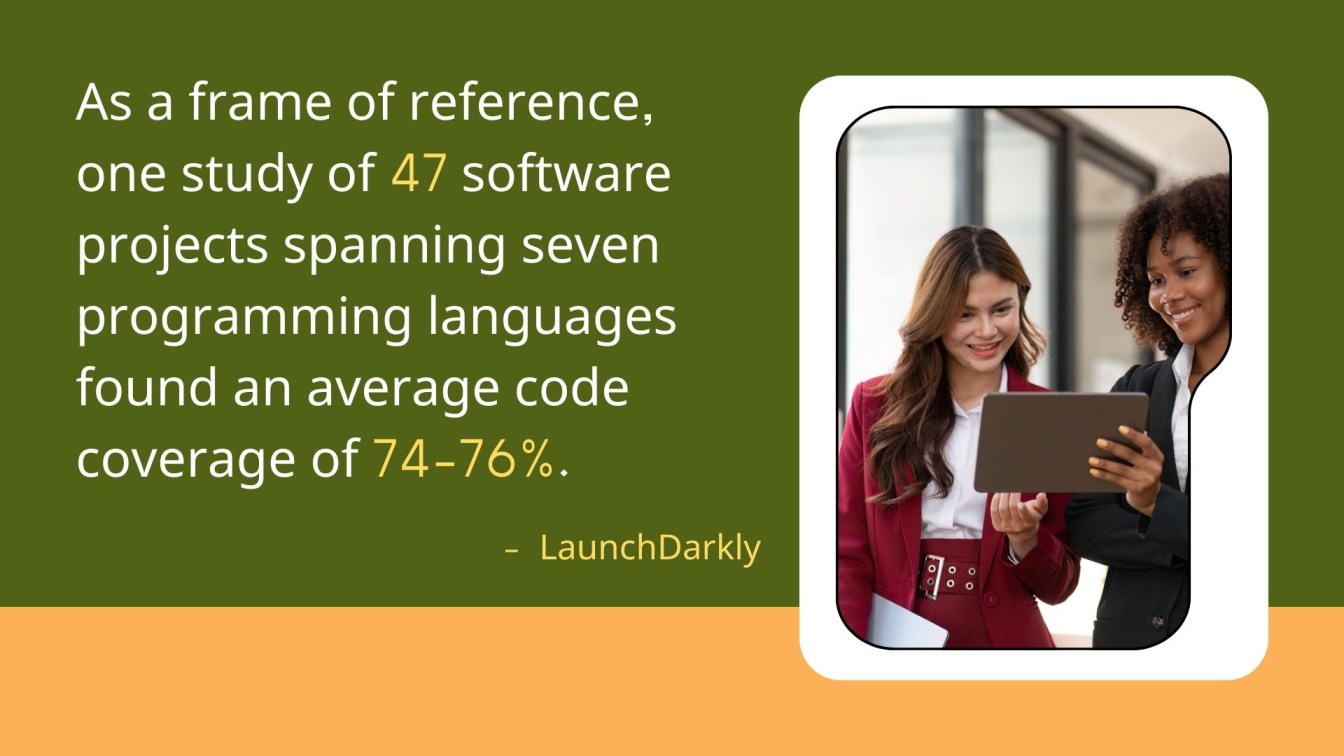
- Identifies Untested Areas: It helps pinpoint untested code paths, ensuring every part of the application is validated.
- Reduces Hidden Bugs: By improving test coverage, the likelihood of undetected defects reaching production is minimized.
- Increases Confidence: High code coverage often leads to greater confidence in the stability and performance of the application.
- Helps in Focused Testing: Developers can prioritize testing of critical and complex code sections that may have been previously overlooked.
While 100% code coverage isn't always necessary, a solid code coverage strategy ensures that the software is thoroughly tested. Tools like JaCoCo, Istanbul, and Coverage.py help generate detailed code coverage reports, enabling teams to optimize their testing efforts and improve overall software quality.
Exploring Different Types of Code Coverage Metrics
When it comes to code coverage, there are various metrics used to measure how thoroughly the code has been tested. Each metric focuses on different aspects of the codebase, providing insights into the effectiveness of testing.
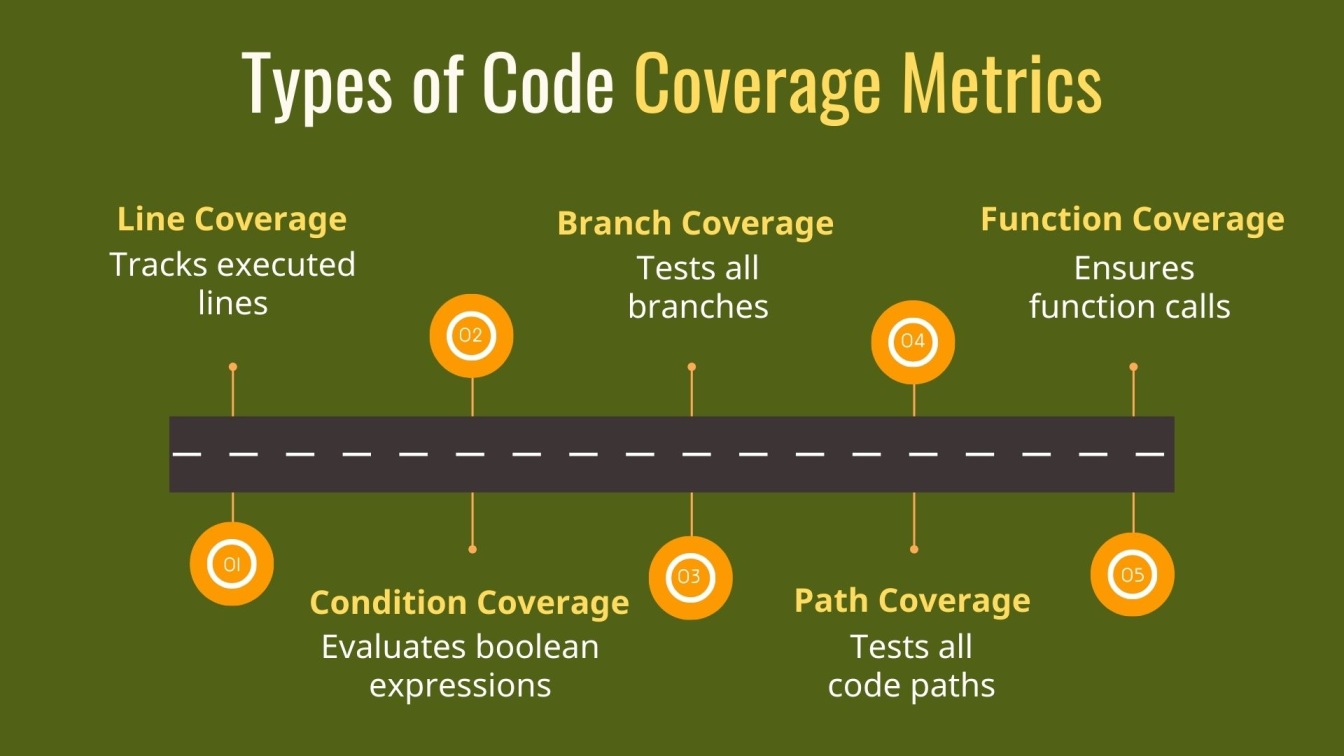
The main types of code coverage metrics are:
- Line Coverage: This measures the percentage of lines of code that have been executed during testing. It’s the most basic form of coverage, but it can be misleading since it doesn’t account for whether all logical branches of the code are tested.
- Branch Coverage: This metric focuses on testing every possible branch in the code (e.g., if-else statements). It ensures that all conditional paths are executed, giving a better view of the code's behavior.
- Function Coverage: This metric tracks whether each function or method in the code has been called during testing. It ensures that all functions are tested, regardless of the conditions they may contain.
- Condition Coverage: This measures whether individual boolean expressions within conditions have been evaluated to both true and false. It helps test complex conditions within control structures.
- Path Coverage: This is a more advanced metric that ensures all possible paths through the code are executed. It’s useful for catching complex bugs that might be missed by simpler metrics.
Code Coverage vs. Test Coverage: What’s the Difference?
Code Coverage
Measures the percentage of the source code that is executed during testing. It focuses on ensuring that all parts of the codebase are exercised through the tests.
Test Coverage
Measures the percentage of functionality or features that are covered by tests. It focuses on verifying the behavior of the software and ensuring that all critical features are tested.
Top Code Coverage Tools for Effective Testing
Effective code coverage tools are essential for ensuring thorough testing and improving quality through quality assurance software. These tools help developers track code execution, identify untested parts, and optimize their testing process. Some of the top code coverage testing tools used across different programming languages include JaCoCo, Istanbul, Coverage.py, SonarQube, and Codecov.
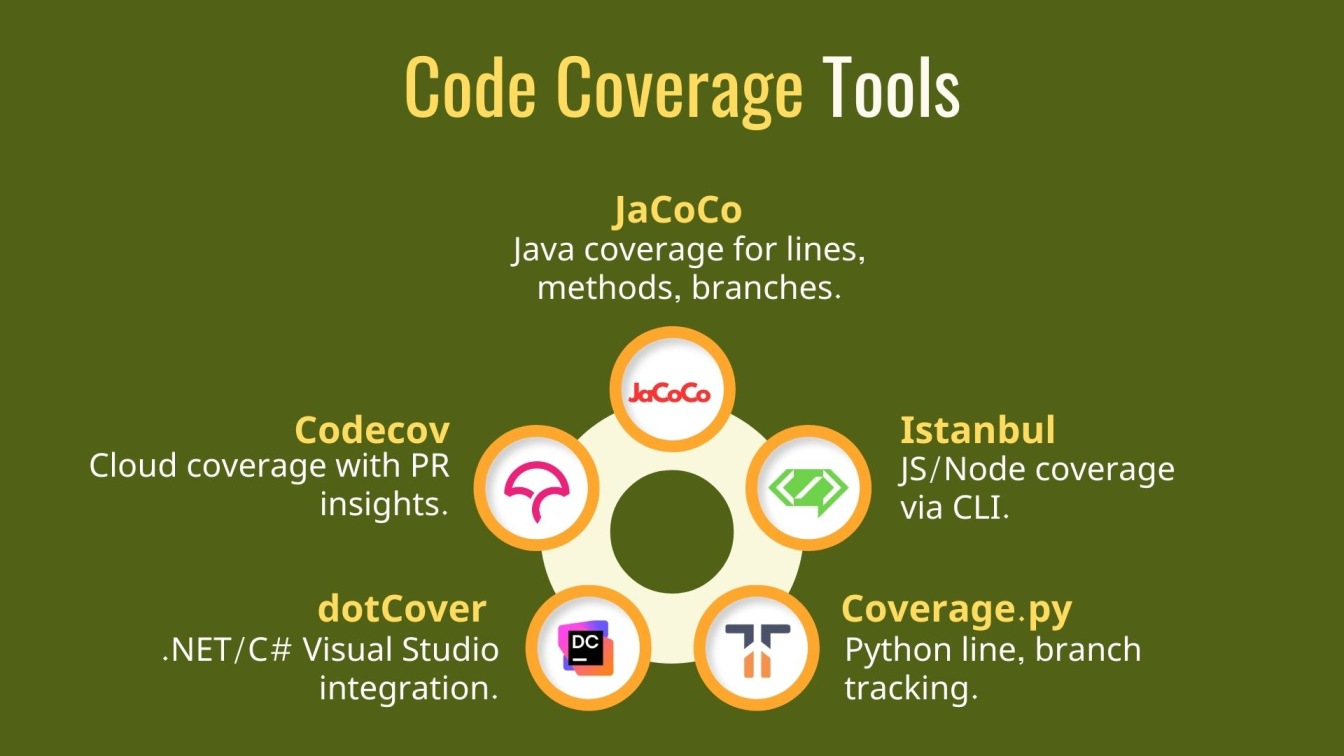
JaCoCo (Java Code Coverage Library)
JaCoCo is a widely used open-source Java code coverage tool that provides insights into how much of your codebase is exercised by unit tests and integration tests. It supports different types of coverage metrics, such as line, method, and branch coverage, helping developers identify untested areas in Java applications.
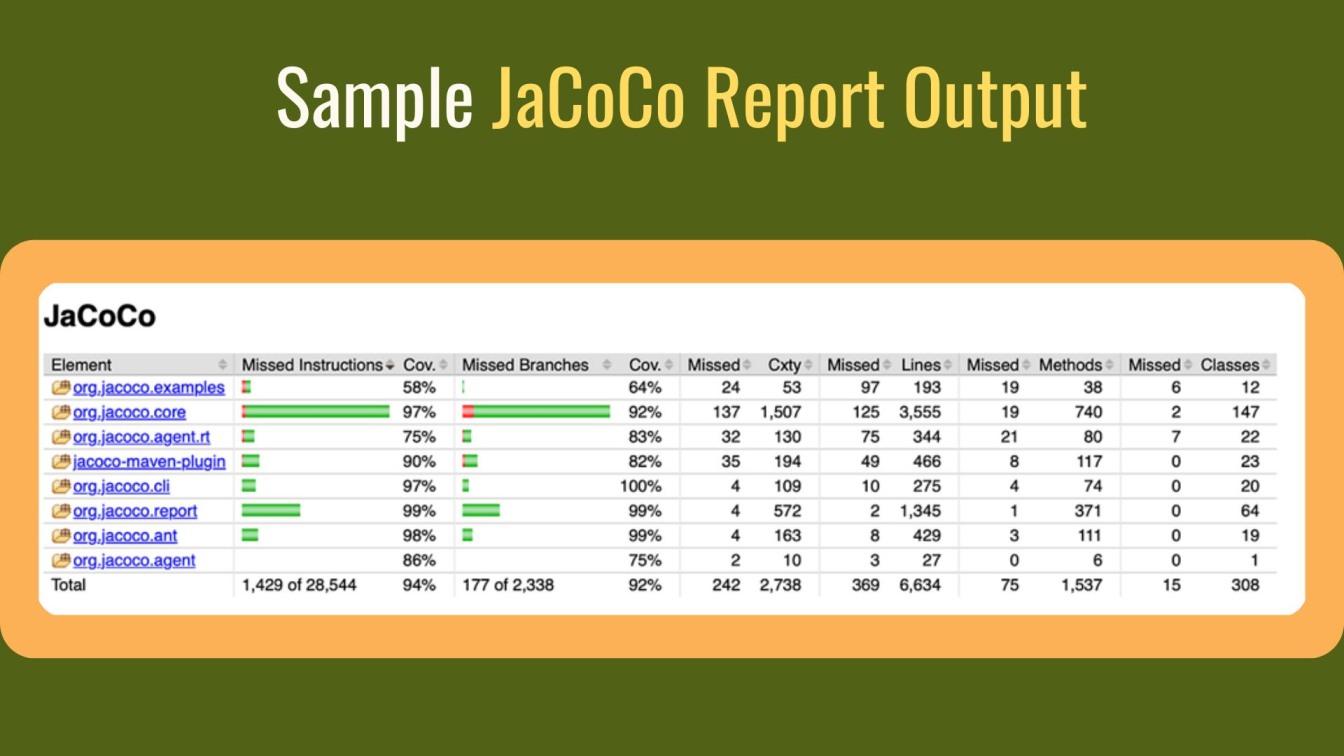
Services Provided by A JaCoCo:
- Integrates seamlessly with JUnit, TestNG, and major build tools like Maven and Gradle.
- Compatible with CI/CD tools like Jenkins for real-time coverage tracking.
- Generates reports in multiple formats (HTML, CSV, XML) for easy analysis.
- Enables code coverage analysis by visualizing which parts of the code are covered.
- Promotes software quality assurance by helping maintain high testing standards in Java projects.
Istanbul (NYC) for JavaScript/Node.js
Istanbul (also known as NYC in CLI form) is a leading code coverage tool for JavaScript applications, especially useful in Node.js environments. It provides comprehensive insights into function, branch, and statement coverage during unit testing.

Services Provided by Istanbul:
- Works effectively with test runners like Mocha, Jest, and AVA.
- Supports integration into CI/CD pipelines for automated coverage validation.
- Generates clean and understandable reports in formats like HTML, LCOV, and text.
- Helps ensure high software quality assurance services by revealing gaps in test cases.
- Assists in building reliable and maintainable JavaScript code through consistent testing.
Coverage.py for Python
Coverag.py is a robust Python code coverage tool that determines the percentage of code executed during test runs. It's especially effective for identifying dead code and ensuring comprehensive test coverage in Python applications.

Services Provided by Coverage.py:
- Supports popular test frameworks like unittest, pytest, and nose.
- Provides detailed HTML and XML reports showing executed and skipped lines.
- Can highlight missing branches, helping in branch condition testing.
- Easily integrates into CI/CD workflows for automated testing coverage tracking.
- Supports thorough software unit testing and boosts confidence in Python codebases.
dotCover (for .NET and C#)
dotCover is a powerful .NET unit test runner and code coverage tool by JetBrains. It helps .NET and C# developers ensure their code is well-tested and maintains high-quality standards. It seamlessly integrates into the development workflow, making it easy to track which parts of your application are thoroughly tested and which are not.

Services Provided by dotCover:
- Supports MSTest, NUnit, XUnit, and other .NET test frameworks
- Provides statement, branch, and method coverage
- Fully integrates with Visual Studio and ReSharper.
- Allows merging of coverage results from multiple runs
- Helps identify redundant or untested code in enterprise .NET projects
Codecov
Codecov is a cloud-hosted code coverage tool that integrates with your version control and CI/CD systems to show how much of your code is tested. It simplifies the process of understanding test coverage and encourages collaborative testing efforts.

Services Provided by Codecov:
- Seamlessly connects with platforms like GitHub, Bitbucket, GitLab, and CircleCI.
- Post coverage changes directly in pull requests, aiding in code reviews.
- Supports a wide range of languages and testing tools, including Java, Python, and JavaScript.
- Provides visual dashboards and detailed analytics for continuous code coverage analysis.
- Encourages better testing unit practices in both small and large development teams.
Key Benefits of Code Coverage in Software Development
Code coverage is a fundamental practice in testing software that helps determine how much of the source code is executed while running automated software testing tools. This insight enables development teams to improve software quality, reduce risks, and build more resilient applications.
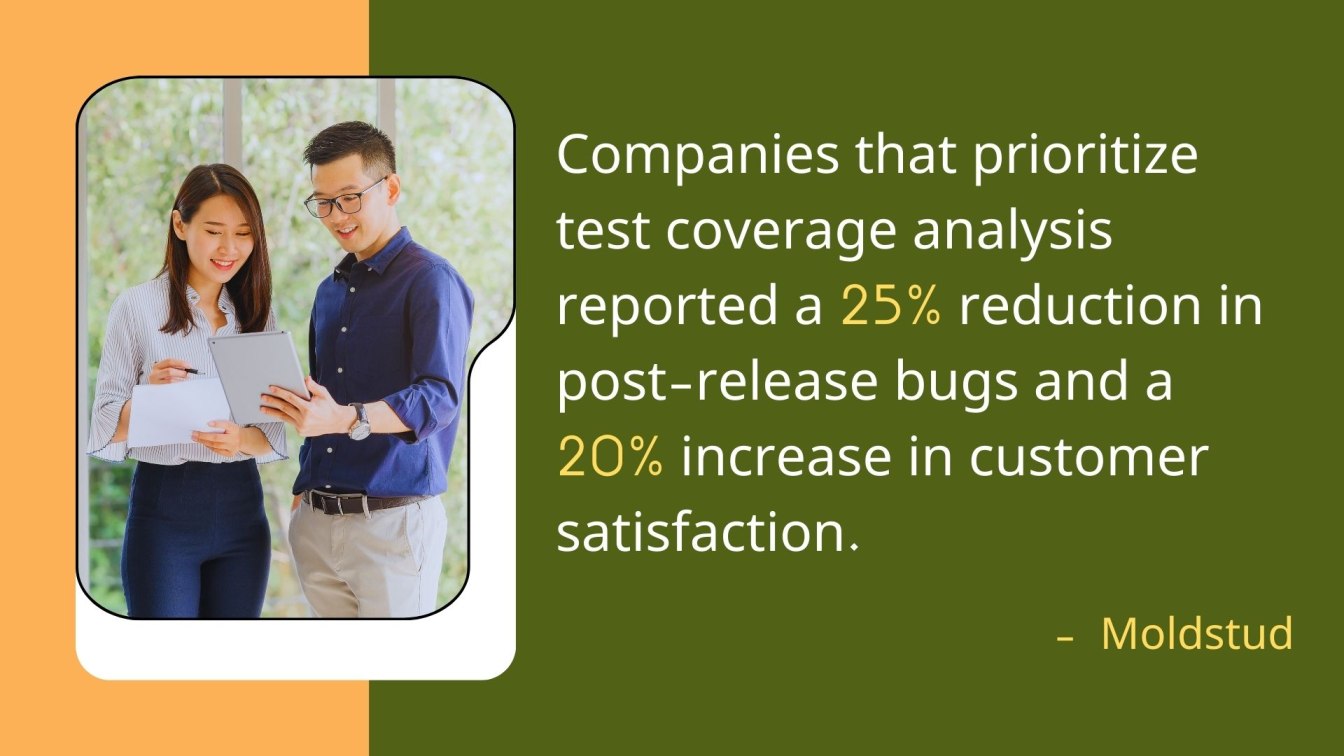
Key benefits include:
- Improved Software Quality and Reliability: Code coverage ensures better unit testing, identifying untested branches and logic, which boosts software quality assurance.
- Faster Bug Detection and Resolution: With tools like JaCoCo, Coverage.py, and Clover code coverage, teams can catch bugs earlier, saving time and reducing costs.
- Increased Confidence in Releases: A detailed code coverage report gives assurance that the most critical parts of the application are well-tested.
- Optimized Testing Efficiency: Code coverage highlights weak or redundant test cases, improving the effectiveness of the test suite.
- Seamless CI/CD Integration: Coverage metrics integrate with modern CI/CD pipelines, such as Jenkins CI/CD, to automate quality checks in every deployment.
Overcoming Common Challenges in Code Coverage
While code coverage is vital for improving software quality, teams often face several hurdles when trying to implement and maintain it effectively within their software testing workflows.
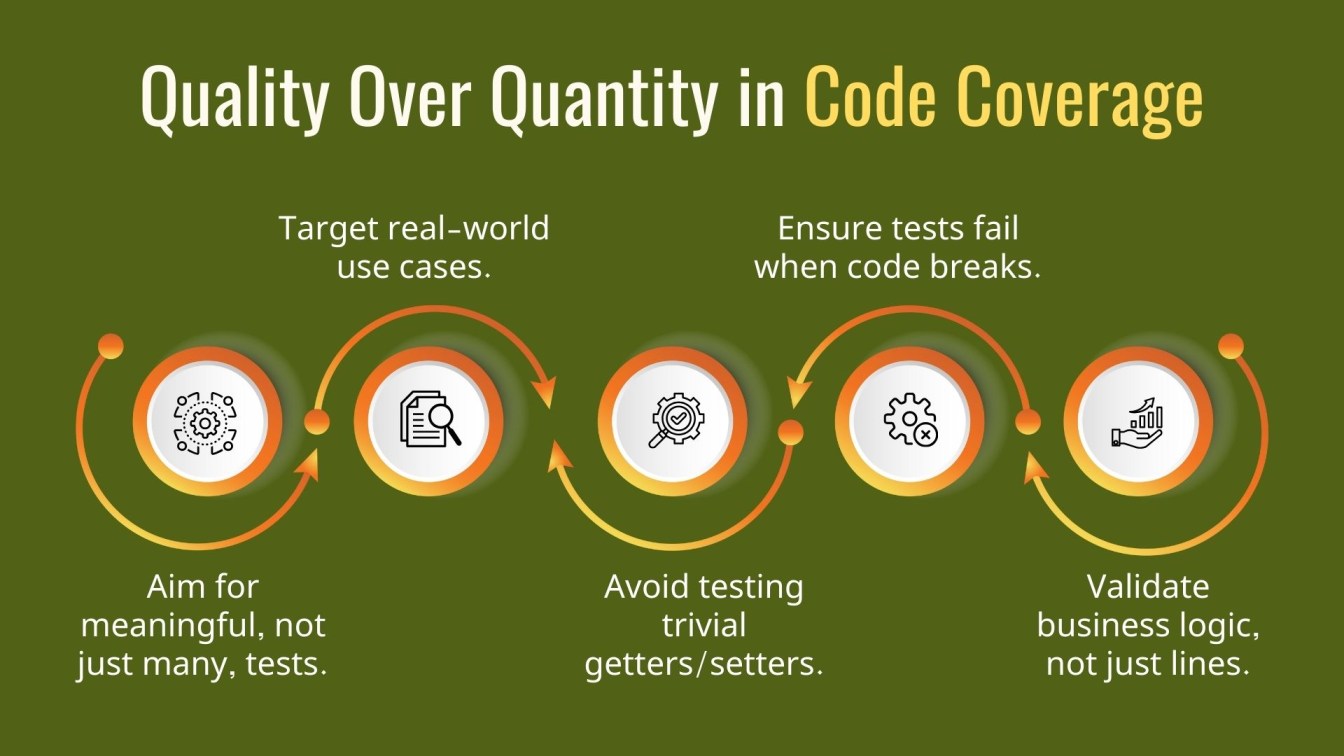
Here are common challenges and how to overcome them:
- False Sense of Security: Achieving high coverage doesn’t always mean better testing. Focus on meaningful unit testing that validates functionality, not just hitting lines of code.
- Neglecting Edge Cases: Some code paths, like error handling or rare conditions, are often skipped. Ensure your tests include these edge cases for better coverage.
- Tool Compatibility Issues: Integrating code coverage tools like JaCoCo, Codecov, or Coverage.py into your build or CI/CD pipeline can be tricky. Choose tools that align with your tech stack and support seamless integration.
- Performance Overhead: Running coverage analysis can slow down the test cycle. Use it smartly, schedule heavy reports in nightly builds, and lighter checks in every commit.
- Lack of Developer Awareness: Educate teams about the value of code coverage and make code coverage reports a regular part of the development review process.
Best Practices for Implementing Code Coverage in Your Projects
Code coverage is most effective when implemented strategically rather than blindly aiming for high percentages. The goal is to build reliable, maintainable tests that genuinely improve the quality of your application.
Here are some best practices to follow:
- Set realistic goals: Instead of chasing 100% coverage, focus on covering the most critical and high-risk areas of your codebase.
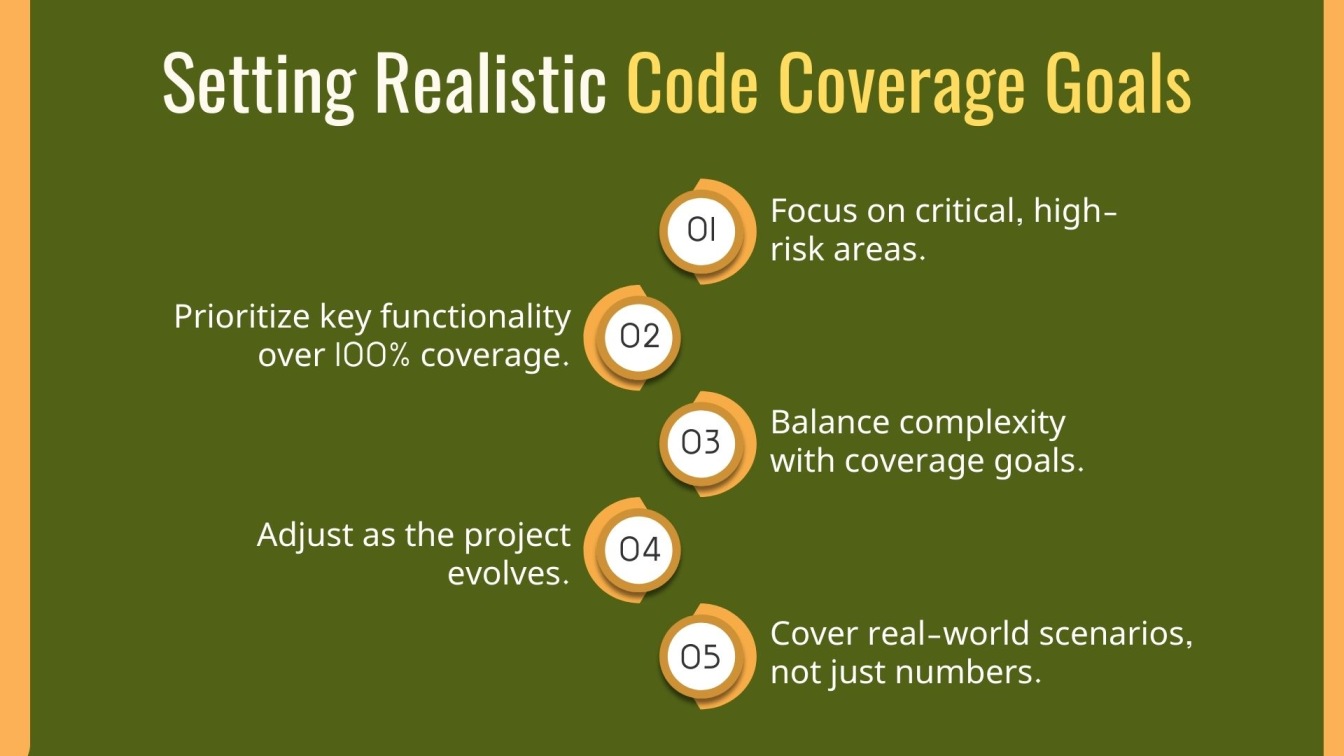
- Analyze detailed reports: Review detailed coverage reports regularly to detect untested code, especially business logic and error-handling blocks.
- Combine different test types: Use a mix of unit, integration, and functional tests for comprehensive testing.
- Focus on quality over quantity: High coverage doesn't always mean good tests. Ensure your tests are meaningful and validate real scenarios.
- Use TDD when possible: Test-Driven Development promotes writing clean, testable code from the start.
Optimizing Code Coverage in CI/CD Workflows
Integrating code coverage into CI/CD workflows is essential for maintaining high software quality and delivering reliable releases at speed. By embedding code coverage tools within your pipeline, you ensure that every change is automatically tested and evaluated.

Here’s how to optimize it effectively:
- Automated Code Coverage Reports: Use tools like JaCoCo (for Java code coverage), Istanbul/nyc, or Coverage.py to generate coverage reports on each commit. These tools can be integrated directly into your CI/CD platforms like Jenkins CI/CD or GitHub Actions.
- Set Coverage Thresholds: Define minimum coverage percentages in your CI/CD configuration. If coverage drops below the threshold, the build fails, enforcing better unit testing discipline.
- Incremental Coverage Tracking: Instead of tracking total project coverage, monitor coverage for changed files to ensure recent code is well tested.
- Feedback in Pull Requests: Tools like Codecov and SonarQube add coverage insights directly into PRs, helping developers make quick, informed decisions.
- Scalable Integration: Use lightweight coverage for frequent builds and full reports in nightly or staging builds to balance performance and detail.
How to Analyze Code Coverage Reports for Actionable Insights
A code coverage report is more than just a percentage—it’s a powerful tool in software testing services that can guide developers toward writing more effective tests and improving software quality through detailed coverage reports.
Here’s how to break down and extract actionable insights:
- Review Covered vs. Uncovered Code: Identify which parts of the codebase are untested. Pay close attention to critical business logic, complex methods, and error-handling blocks.
- Focus on Low-Coverage Hotspots: If certain files or functions are heavily used but poorly tested, prioritize adding unit testing there to reduce risk.

- Check Coverage by Type: Analyze different metrics like statement, branch, and path coverage using tools like JaCoCo, Clover code coverage, or SonarQube for more depth.
- Integrate with CI/CD Dashboards: Automate the generation of detailed reports using CI/CD tools such as Jenkins CI/CD, making them easily accessible to the whole team.
- Track Coverage Trends Over Time: Monitor progress and prevent regressions using tools like Codecov or Jest code coverage, which provide historical insights.
Conclusion: The Role of Code Coverage in Modern Software Testing
In modern software testing, code coverage is vital for ensuring a software application is thoroughly tested before deployment. While it doesn't guarantee a bug-free product, it highlights untested areas, such as missed conditional statements, helping teams enhance their test suites. Tools like JaCoCo, Codecov, and Coverage.py - each a popular tool—support this effort.
Integrated into CI/CD pipelines, code coverage tracks code health across development environments. Using open-source code coverage tools, teams can generate automated, detailed coverage reports and fix issues early. Metrics like decision coverage ensure all logical paths are tested for better software quality.
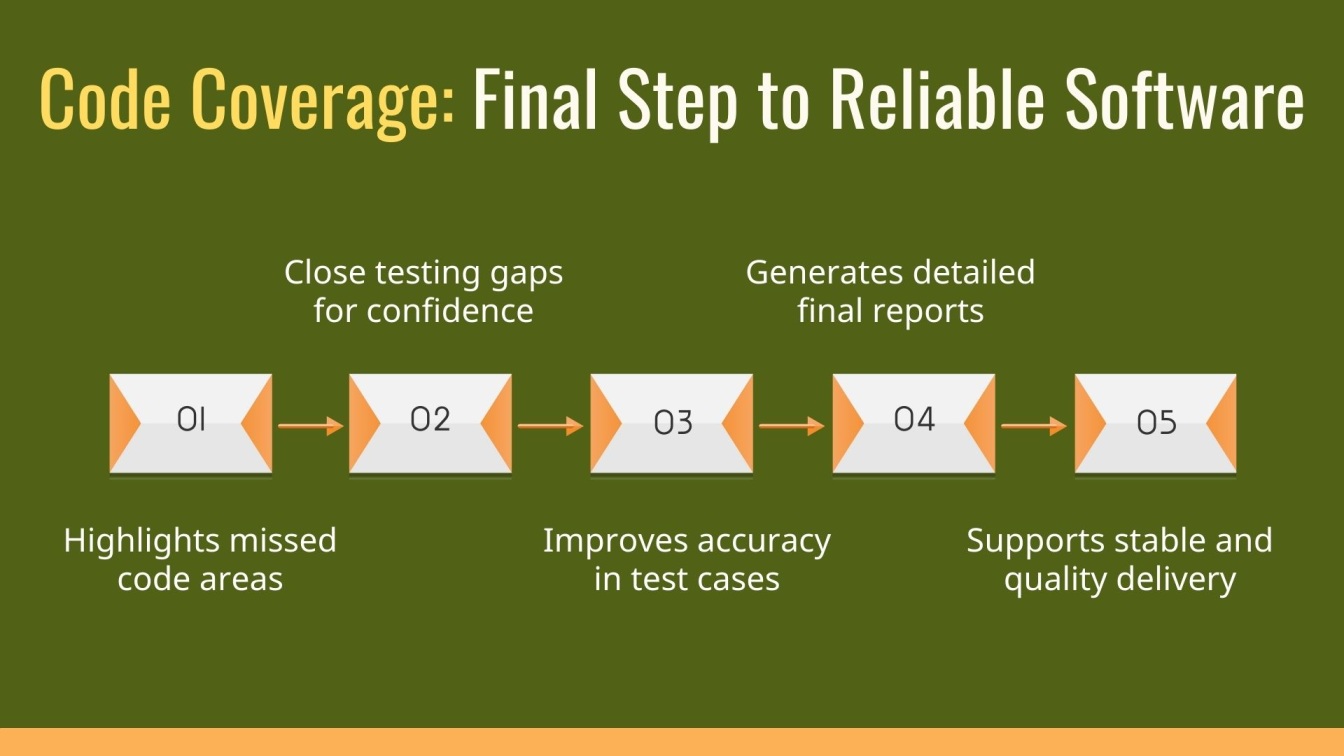
Overall, code coverage improves testing efficiency, reduces risks, and supports faster, more reliable delivery. It’s essential for scalable, high-quality development Overall, code coverage improves testing efficiency and supports continuous integration by enabling faster, more reliable delivery. It reduces risks and is essential for scalable, high-quality development workflows.Frugal Testing is a leading SaaS application testing company known for its AI-driven test automation services. Among the services offered by Frugal Testing are cloud-based test automation services that help businesses improve testing efficiency, ensure software reliability, and achieve cost-effective, high-quality product delivery.
People Also Ask
Does Cobertura do code coverage?
Yes, Cobertura is a popular code coverage tool for Java that calculates and reports code coverage metrics for unit tests.
Is 100% code coverage necessary?
No, 100% code coverage is not always necessary. While high coverage is beneficial, it’s more important to focus on testing critical and complex paths rather than aiming for 100%.
How does code coverage affect refactoring?
Code coverage ensures that existing functionality is preserved during refactoring by validating that tests pass after code changes, reducing the risk of introducing bugs.
Is it worth tracking code coverage in small projects?
Yes, tracking code coverage in small projects helps ensure quality from the start, making it easier to maintain and scale as the project grows.
How do code coverage tools work under the hood?
Code coverage tools monitor the execution of code during tests, tracking which lines, branches, or paths are executed, and then generate reports that highlight untested areas.