Unit testing plays a key role in improving code quality, reducing bugs, and managing the development environment. Testing independent units of code in isolation allows the developers to identify potential issues early, saving valuable time and effort.
But what is unit testing? How does it contribute to better software quality and fewer bugs? Let’s dive deeper into its impact. 🚀
What will be covered in this blog:
Understanding Unit Testing: Learn the fundamentals of unit testing and how it contributes to better software quality.
Key Benefits: Explore how unit testing improves code reliability, maintainability, and debugging efficiency. 🔧
Best Practices for Bug Prevention: Discover strategies to detect and fix bugs early, ensuring smoother development cycles with unit testing.
Tools and Frameworks: Gain insights into popular unit testing tools like JUnit, TestNG, and Pytest for efficient implementation. 🛠️
Real-Life Examples: See practical examples of how unit tests handle edge cases, improve APIs, and ensure modular designs. 💡

Why Unit Testing is Essential for Software Quality?
Unit testing lies at the heart of a reliable and maintainable software development lifecycle. It concentrates on small, testable units of code. This approach to software testing guarantees that every component operates as intended.
Improves Code Quality: Unit testing validates individual units of code, ensuring they function correctly. This process improves software quality and encourages trust in code changes.
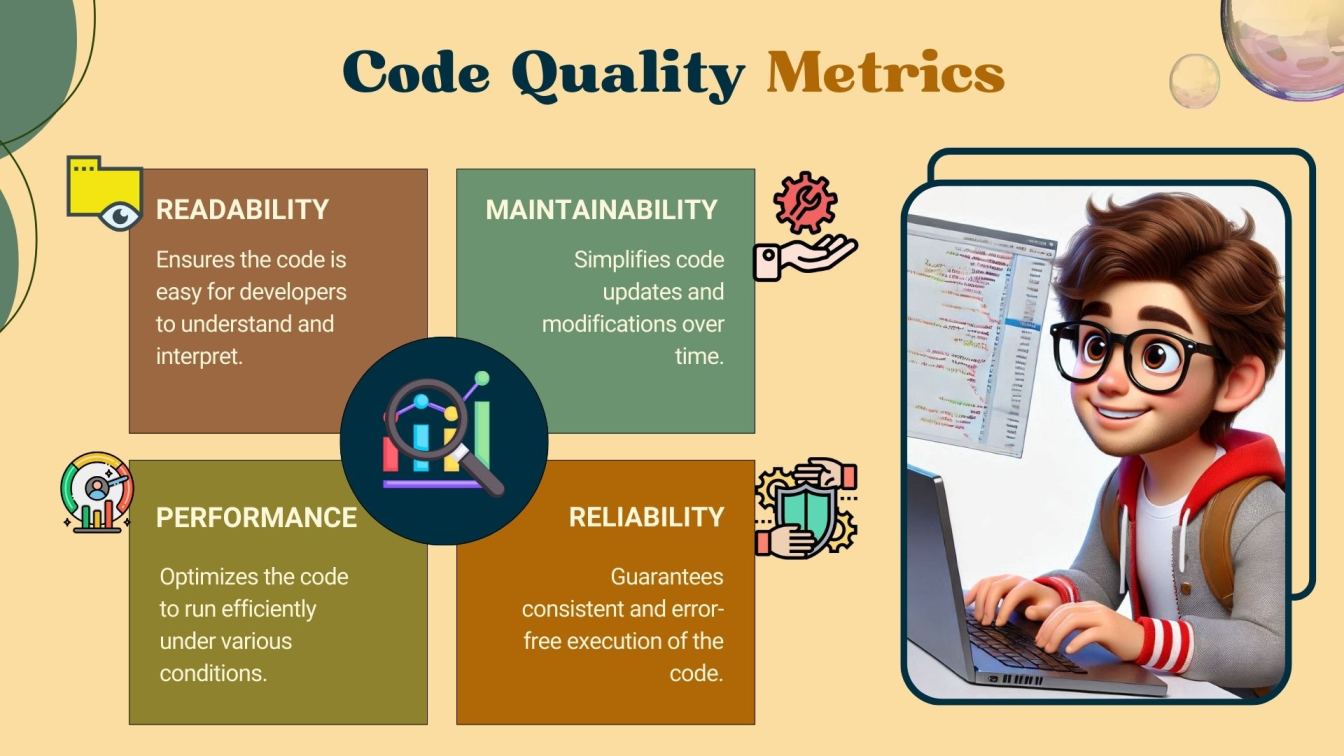
- Encourages Modular Design: Testing small units encourages modular coding practices, where the components in isolation are self-contained and reusable.
- Acts as a Safety Net: Unit tests act as a safety net during code refactoring or feature additions and help developers avoid introducing new bugs.
With the growing complexity of software applications, unit testing is no longer just a good-to-have practice but an essential step toward reliable and maintainable code.
Ensuring Code Reliability with Unit Tests
Code reliability is one of the primary goals of unit testing. A suite of unit tests ensures that each function or component works as intended, even if the software evolves.
- Testing in Isolation: By isolating the unit under test from external components, developers can verify its functionality without interference and ensure accurate feedback on code behavior.
- Achieving Confidence in Code Changes: Unit tests provide a sense of security during development, allowing teams to make changes with a higher level of confidence.
- Boosting Code Maintainability: Reliable code is maintainable code. Unit testing promotes maintainable codebases by reducing the chances of introducing regressions during updates.
By adopting practices like Test-Driven Development (TDD), developers can utilize the power of unit testing to ensure each line of code is functional and reliable before progressing to the next step.
Detecting and Fixing Bugs Early in Development
Early bug detection is crucial for reducing development costs and ensuring faster development cycles. Bugs identified later in the software development process often take longer to fix and can disrupt the development workflow.
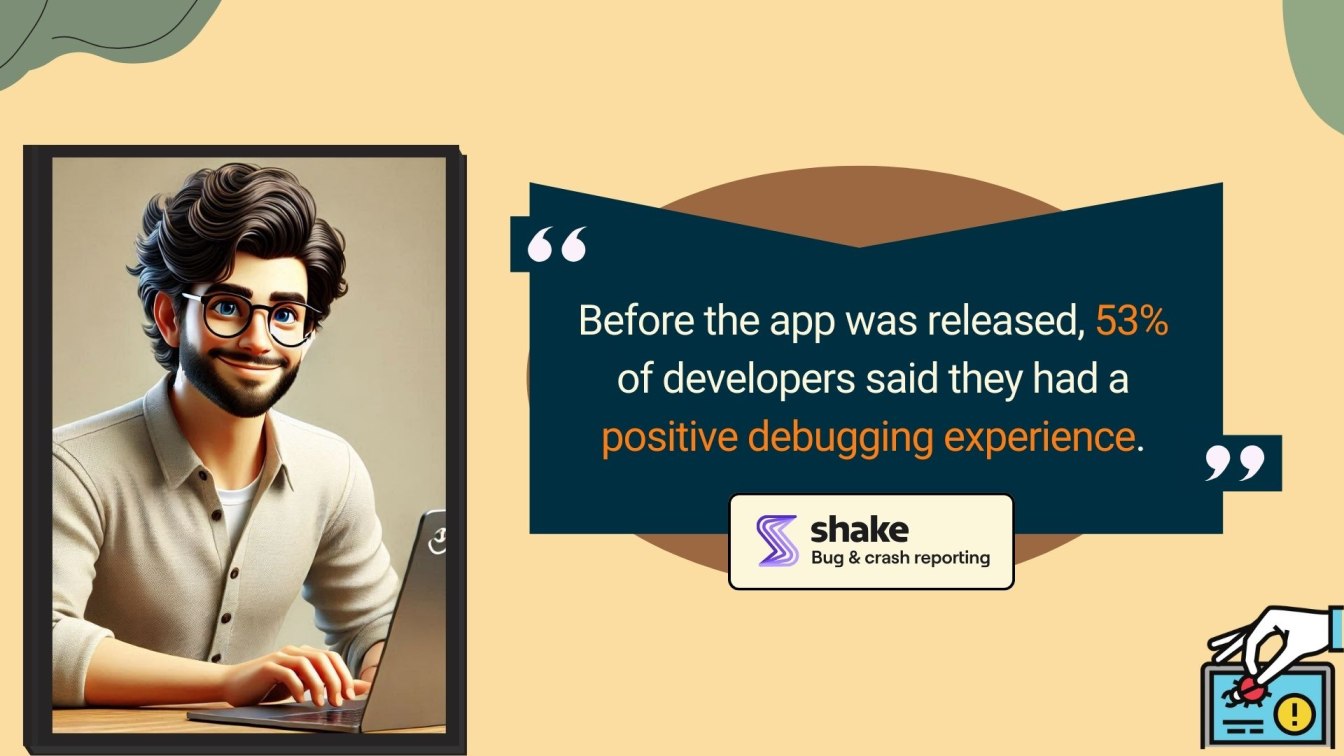
- Cost-Effective Debugging: Fixing bugs during the initial testing phase is significantly cheaper than addressing them after deployment.
- Streamlined Development Workflow: Detecting issues early helps keep development workflows smooth and uninterrupted.
- Avoiding Rework: Bugs caught early reduce the likelihood of major rework during later development stages.
Imagine a scenario where a unit test identifies a null pointer exception in a function responsible for handling user inputs. Detecting this issue early prevents downstream failures and saves the team from extensive debugging later. 🔍
Facilitating Better Code Refactoring
Refactoring is one of the most important practices that helps to keep the codebase clean and efficient, but refactoring can be dangerous if it is done without testing. Unit tests as a safeguard that allows developers to refactor code with confidence.
- Encouraging Cleaner Code: This is because developers will be encouraged to refactor code knowing that any issues that may arise will be identified by the unit tests.
- Supporting Agile Practices: Refactoring is important in agile development cycles, enabling teams to adapt and improve continuously.
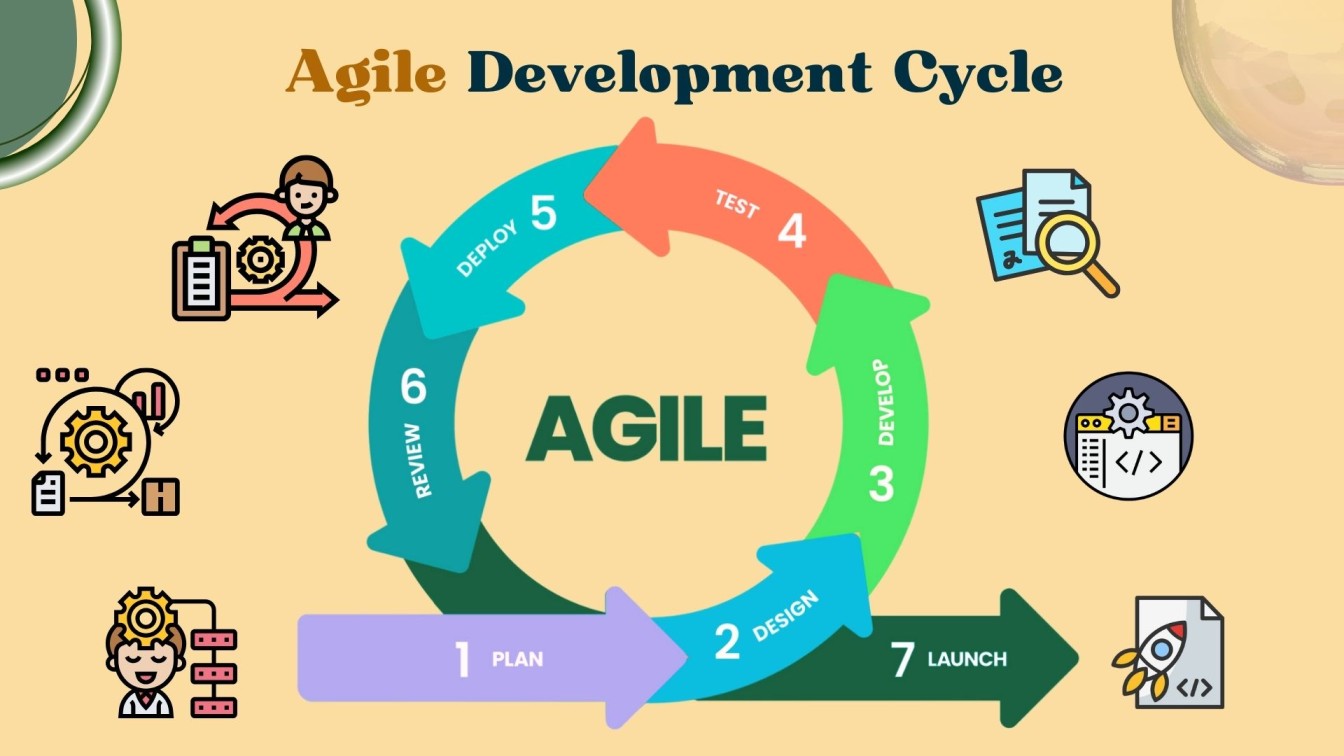
For instance, refactoring a payment gateway function to improve performance might seem daunting, but with reliable unit tests in place, developers can confidently make the necessary changes. 🔄
Key Benefits of Unit Testing
Unit testing produces high-quality, reliable software. It offers several benefits that make software development smoother, faster, and more collaborative. Let’s explore these in detail! 🚀
Improved Code Quality and Maintainability
One of the advantages of unit testing is its direct impact on code quality. The power of unit testing lies in its ability to validate significant lines of code, ensuring quality and maintainability throughout the software lifecycle
- Promotes Scalability: Well-tested code can be scaled or extended without risking system integrity.
- Simplifies Maintenance: With unit tests in place, maintaining the codebase becomes easier. Developers can quickly verify whether a change affects any existing functionality.
For example, in a RESTful API, unit tests can ensure that each endpoint returns the expected response body, response code, and response headers, making the API more dependable. ✅
Faster Debugging and Reduced Downtime
The most time-consuming part of developing software is frequent debugging. Unit testing saves time by identifying the exact point of failure in the code.

- Pinpoints Issues Quickly: Since unit tests cover individual components, they make it easier to locate the root cause of a failure without scanning the entire codebase.
- Minimizes Disruptions: By catching and fixing bugs early, unit testing minimizes disruptions, ensuring smoother development cycles and quicker product delivery.
Imagine working on a complex application where a unit test flags an issue with the request payload of a POST request. This saves countless hours of manual debugging and ensures the application's functionality and reliability. 🔧
Streamlining Collaboration Among Developers
In a collaborative environment, ensuring everyone is on the same page can be challenging. Unit testing helps bridge this gap by creating a shared understanding of the codebase.
- Encourages Code Ownership: Unit tests make developers more accountable for their code, ensuring quality standards are maintained across the team.
- Supports Continuous Integration (CI): Unit tests integrate seamlessly into CI pipelines, enabling teams to identify and fix issues during early development stages.
For instance, in a Maven project, running unit tests automatically as part of the CI/CD pipeline ensures all changes are validated before deployment. This fosters collaboration and trust among team members. 🤝
How Unit Testing Reduces Bugs
Reducing bugs effectively is one of the major benefits of Unit Testing. By focusing on small, testable units of code, developers can identify and address issues before they escalate.
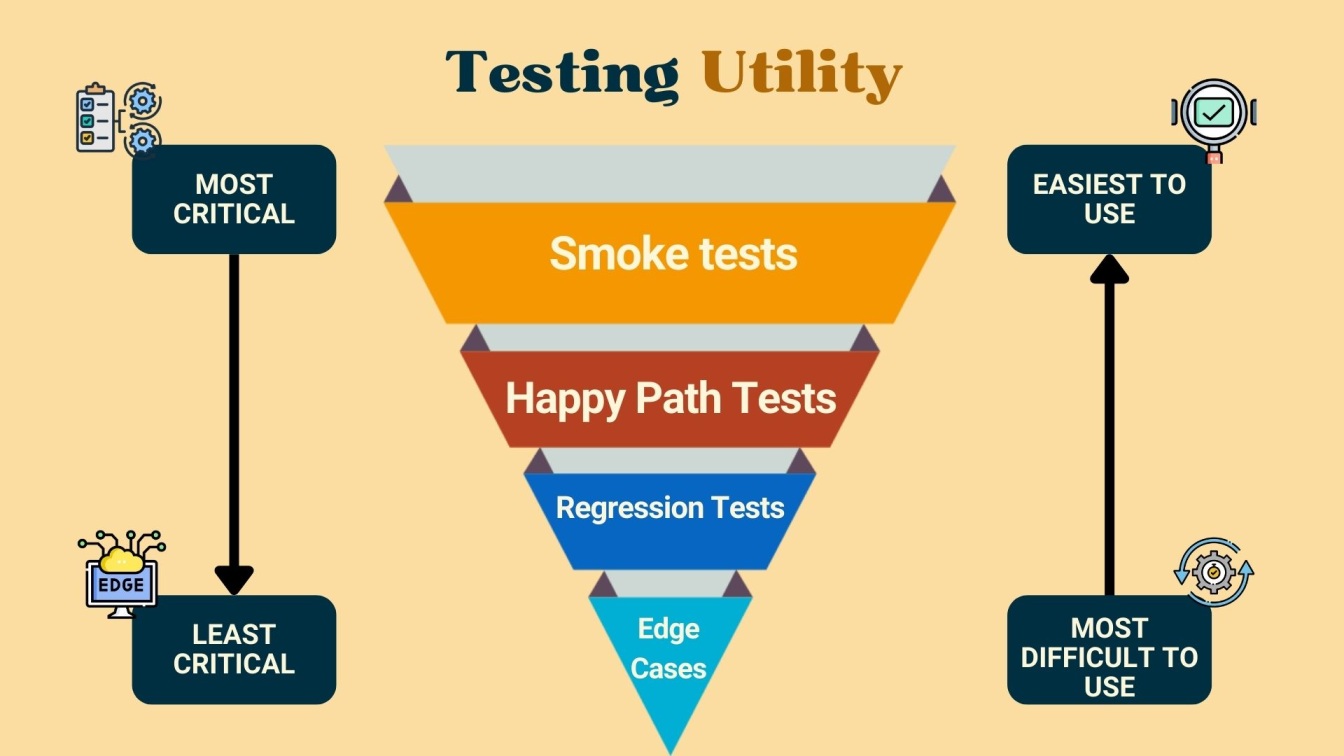
Identifying Edge Cases and Code Vulnerabilities
Unit tests play a vital role in identifying edge cases; the rare scenarios that might not follow the standard workflow but can cause unexpected failures if left unaddressed.
- Handles Unexpected Inputs: Unit tests ensure the code gracefully handles unexpected or invalid inputs, enhancing its reliability.
- Detects Security Vulnerabilities: Testing edge cases helps uncover vulnerabilities like buffer overflows or injection attacks, strengthening software security.
For example, in an e-commerce platform, unit tests can simulate edge cases such as applying invalid coupon codes or attempting to place an order with an empty cart to validate how the system reacts. 🛒
Reducing Regression Issues with Frequent Testing
Regression problems happen when updates unintentionally disrupt functionality that already exists. Unit testing acts as a shield against such issues.
- Ensuring Stability Over Time: Teams can refactor code without worrying about breaking existing functionality with a comprehensive suite of unit tests.
- Saves Time in the Long Run: Early detection and resolution of regressions reduce debugging efforts, saving significant time and resources.
Consider a scenario where an update to the login module of an application breaks the profile creation feature. A well-designed suite of unit tests would immediately flag this issue, allowing the development team to address it promptly. 🔄
Unit Testing Tools and Frameworks
Unit testing serves as a cornerstone for developing reliable, bug-free, and maintainable software. But it’s not just the methodology that matters—it’s also the tools and frameworks developers use to implement unit tests. Let’s explore the essential tools and frameworks for popular languages like Java, Python, and JavaScript.
Popular Unit Testing Frameworks in Java, Python, and JavaScript
Each programming language has its unique ecosystem of unit testing frameworks. Picking the right tool can simplify workflows and enhance code reliability.
1. Java
In Java-based applications, the most commonly used frameworks are:
- JUnit: JUnit is a Java-based library and one of the most popular unit testing frameworks. It integrates effortlessly with Maven projects and offers annotations like @Test and @BeforeEach for seamless test management. With the right Maven dependencies, you can set up your unit testing environment in minutes.
- TestNG: Known for its advanced features like parallel testing, TestNG is perfect for handling large-scale applications and RESTful web services testing. It enables developers to test API request methods such as GET, POST, DELETE, and PUT more efficiently.

This example demonstrates how Java developers can test small, focused functionalities like addition logic. The use of assertions ensures the test validates both the expected and actual outcomes.
2. Python
Python developers also have a wealth of unit testing frameworks to choose from, including:
- Pytest: Pytest is a domain-specific language for Python-based unit testing. It excels in simplicity and allows concise code snippets for complex test scenarios.
- Unittest: As a built-in Python library, Unittest is favored for its robust, modular test structures.

Pytest’s ability to handle edge cases and its compatibility with REST web services make it a go-to framework for API test automation.
3. JavaScript
JavaScript’s rise in modern web applications has made unit testing indispensable. The most commonly used frameworks are:
- Jest: Jest is a comprehensive testing framework favored for React and Angular applications. It simplifies testing UI elements, REST endpoints, and even mock services.
- Mocha: Mocha provides flexibility with asynchronous testing, making it ideal for projects requiring API automation testing and response validation.

This Jest example is simple yet powerful for testing the logic of JavaScript functions, ensuring clean and reusable code.
Automating Tests for Improved Efficiency
Automation is critical for managing modern web applications, reducing testing efforts, and improving response time for issue detection. The idea of unit testing evolves significantly when Automated testing becomes part of the development pipeline. Below are key ways automation enhances efficiency:

Benefits of Automated Unit Testing
- Continuous Integration Pipelines: Tools like Jenkins and GitHub Actions integrate with unit testing frameworks, triggering automated unit tests after each code commit. These tools ensure a seamless unit testing process by catching software bugs at the early stages of development and reducing development time.
- Error Detection in Functional Testing: Automating functional tests, such as validating RESTful APIs for various types of tests (e.g., POST requests and DELETE requests), ensures every piece of code performs as expected. Automation helps verify the actual code behavior while minimizing manual testing efforts.

- Enhanced Collaboration: Automation fosters teamwork by providing a consistent testing strategy, especially during complex software development. Collaboration among software developers is improved with shared insights into the functional code and source code stability.
Best Practices for Writing Effective Unit Tests
Effective unit testing isn’t just about running tests; it’s about ensuring the tests are meaningful, maintainable, and scalable over time.
Writing Clean and Independent Test Cases
Clean and independent test cases improve debugging and enable better code design. Proper practices in software development, such as writing reusable unit test code, ensure smooth code flow and long-term scalability.
Best Practices for Writing Test Cases
- Use the AAA Pattern: Arrange, Act, Assert. This pattern ensures a logical structure for your test cases, making them easier to read and maintain.
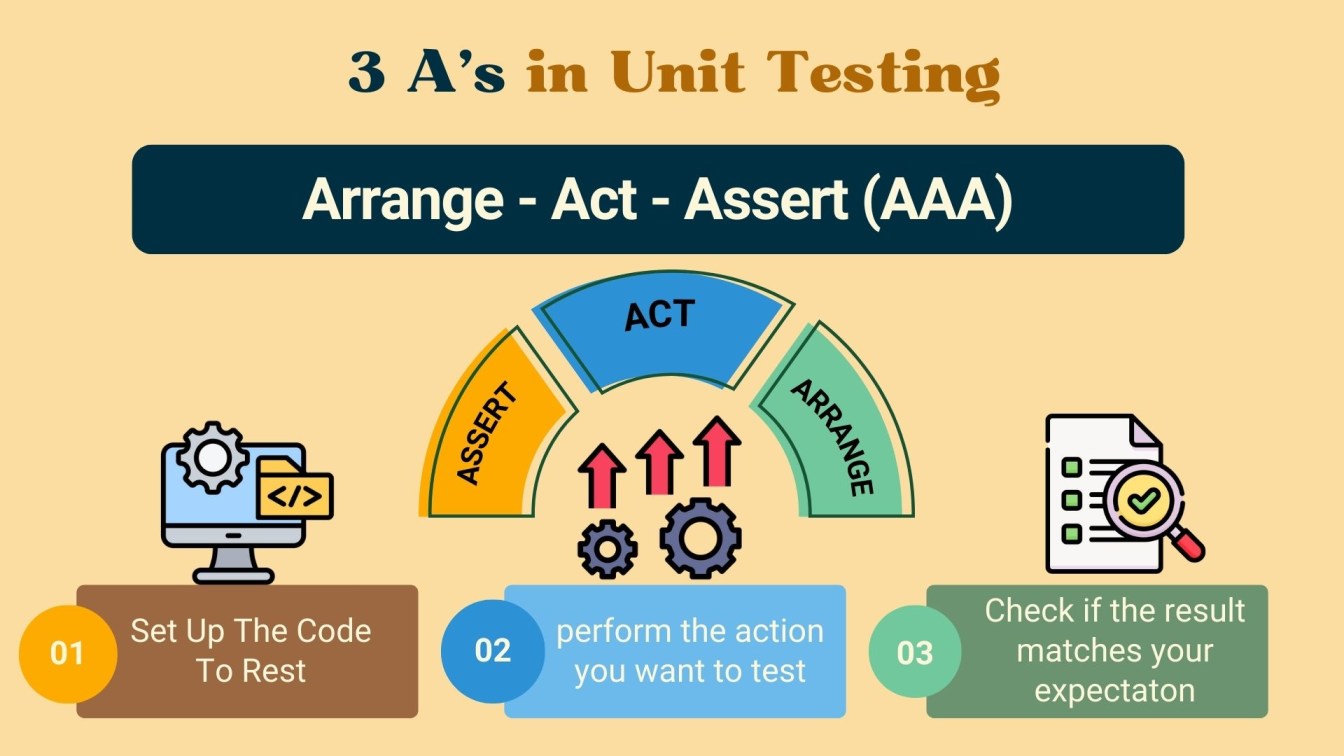
- Mock External Dependencies: Mock objects simulate real-world scenarios without needing access to software components like APIs, databases, or third-party services. This supports effective integration testing for verifying component interactions.

- Descriptive Naming: Always name your test cases(positive tests, negative tests, etc.) clearly to convey their purpose. Descriptive names prevent confusion during future developers’ debugging efforts.

- Condition Coverage: Test conditions extensively to achieve optimal types of code coverage, such as condition coverage and statement coverage, ensuring the detection of error conditions and reducing the likelihood of false positives.
Maintaining Test Coverage Across the Codebase
High test coverage ensures that the most critical parts of your application, like application components and individual functions, are rigorously tested. Achieving extensive types of testing coverage reduces development risk and ensures a comprehensive testing strategy.
Steps to Maintain Test Coverage
- Focus on Core Logic: Prioritize testing the core code base and the application logic. This includes testing critical RESTful web services, such as authentication endpoints and integration between component functions.
- Regular Audits: Use tools like JaCoCo (Java) or Istanbul (JavaScript) to track coverage percentages and identify untested areas in the current code. Reports generated by these tools provide feedback on code quality.
- Integrate Coverage Tools: Automation tools within continuous integration pipelines and continuous deployment pipelines are essential for maintaining consistent test coverage across all stages of development.
- Include Broader Testing: Complement Automated testing with broader approaches like black-box testing and white-box testing to ensure both functional tests and non-functional requirements are met.
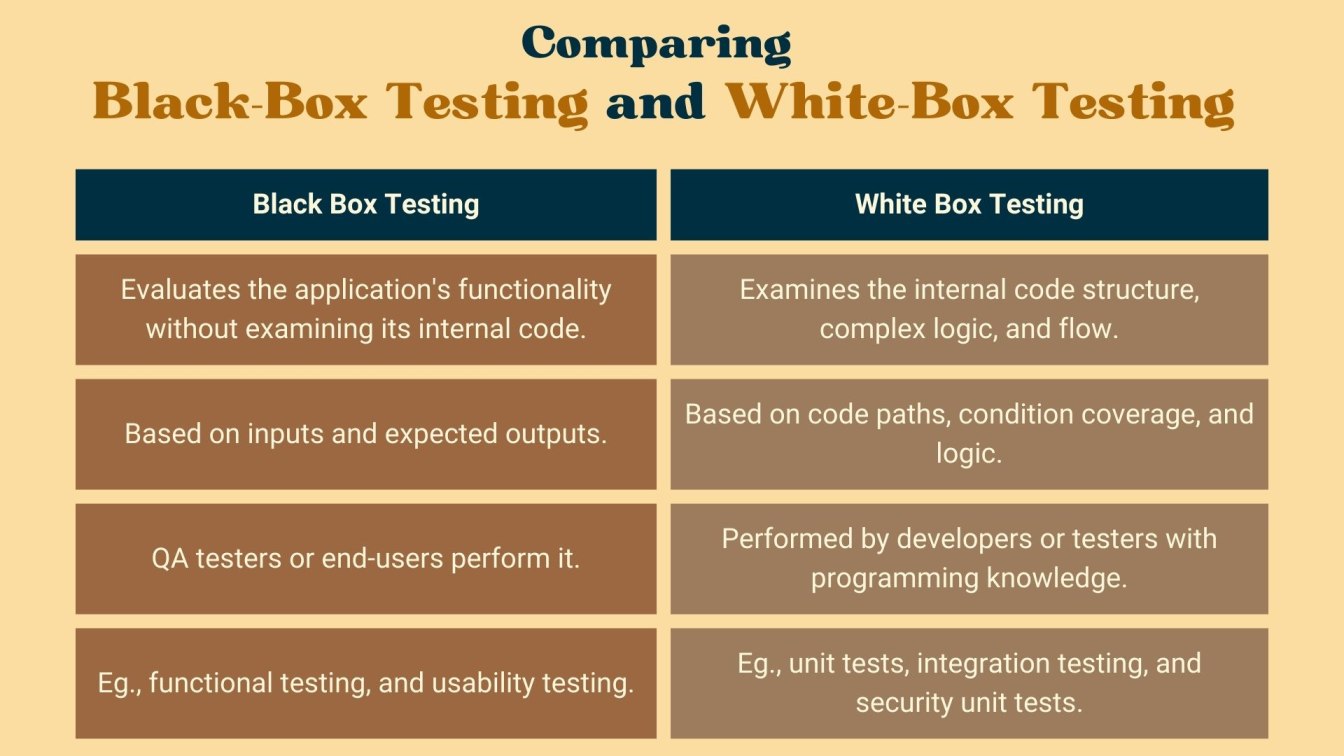
As we bring this to a close
Unit testing plays a crucial role in modern software development, especially for RESTful web services, APIs, and large-scale applications. By using tools like JUnit, Pytest, or Jest, automating repetitive tasks, and sticking to best practices, developers can produce reliable software with fewer bugs and faster delivery.
Strive for complete test coverage, keep test cases simple and well-structured, and build a strong automation framework to make your testing process efficient. Happy coding and testing! 🎉
People also asked
👉How is unit testing different from QA testing?
Unit testing tests the individual units/parts of code to ensure they work as expected, while QA testing looks at the application as a whole, focusing on overall functionality and user experience.
👉What are some limitations of unit testing?
Unit testing often doesn’t catch issues that occur when different components work together. It also requires a time investment upfront and can create overconfidence if tests aren’t thorough.
👉How do you prioritize fixing bugs?
Bug priority is based on how much the issue affects functionality, user experience, and the project’s timeline or goals.
👉Why are unit tests essential in Agile development?
Unit tests allow quick feedback on new code changes, support continuous testing, and reduce the risk of unexpected issues in fast-paced Agile environments.
👉How can I improve my unit test coverage?
Focus on critical code paths, including edge cases, use condition coverage techniques, and integrate testing into continuous integration pipelines.