Are you getting ready for a Selenium interview and finding the sheer number of concepts to learn a little daunting? You've come to the correct place, so don't worry! The most common Selenium interview questions, ranging from the fundamentals to the more complex ones, are collected in this blog.
This guide has something for everyone, for a new employee trying to make a good first impression or an experienced professional hoping to advance to a senior position. You'll be confident when you enter your interview with questions about important ideas, practical situations, and problem-solving strategies.
You will know exactly what to anticipate and how to ace the Selenium WebDriver, locators, waits, frameworks, and other questions by the end of this blog. Keep reading - success is just a scroll away! 📜
Here’s what’s coming up in this blog:
📌 Basics: Selenium components, locators, WebDriver, alerts, pop-ups, windows.
📌 Testing Techniques: POM, data-driven testing, dynamic elements, Selenium Grid.
📌 Advanced Skills: AJAX, Fluent Wait, cross-browser, headless file handling.
📌 Frameworks & Tools: Hybrid frameworks, TestNG, Jenkins, database testing.
📌 Best Practices: Test suite maintenance, dependencies, notifications, broken links
Beginner-Level Selenium Interview Questions
What is Selenium, and what are its components?
Selenium is an open-source automation testing tool used to test web applications across multiple browsers and platforms. It supports various programming languages like Java, Python, C#, and JavaScript. Its key components include:
- Selenium IDE: A browser extension for record-and-playback testing.
- Selenium RC is a deprecated tool that enabled browser automation via a proxy server
- Selenium WebDriver: A tool for cross-browser automation using browser-specific drivers like Chrome WebDriver and Firefox WebDriver.
- Selenium Grid: Enables parallel execution of tests across multiple machines and browsers.
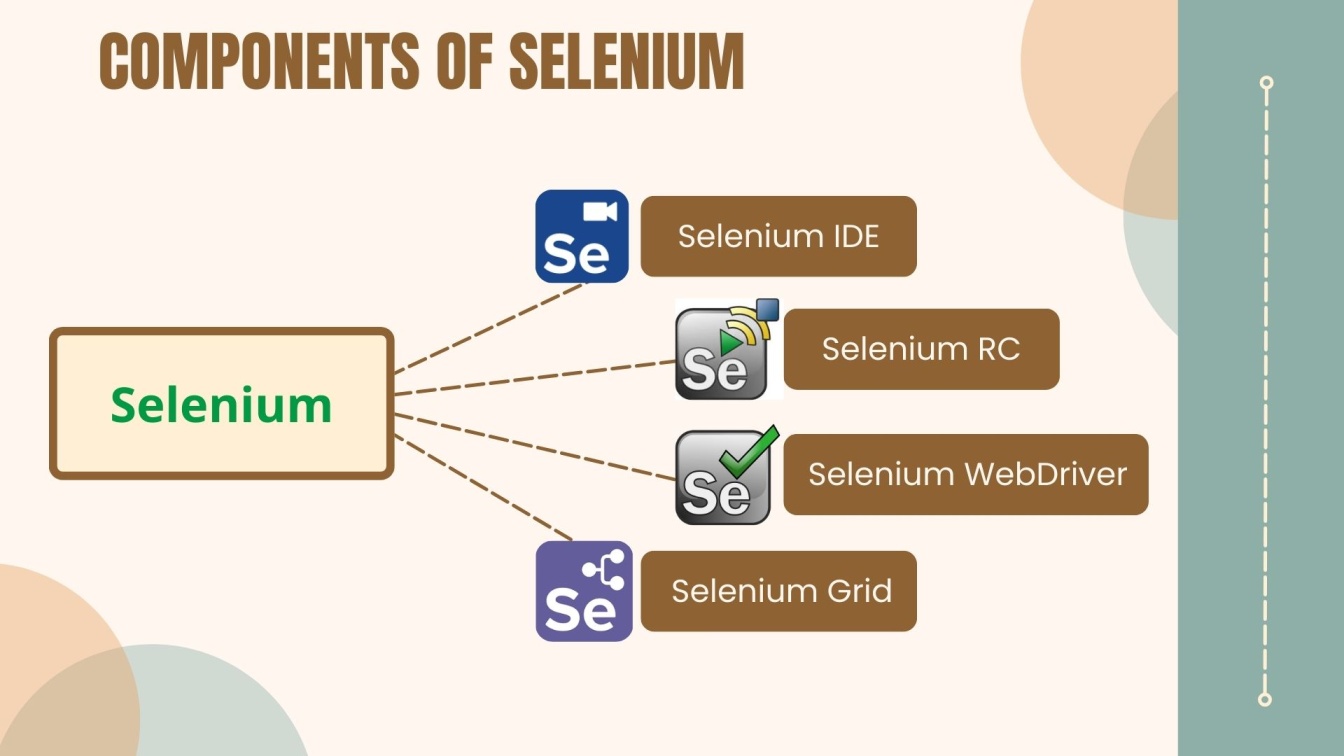
How does Selenium WebDriver differ from Selenium IDE?
The Selenium IDE is a browser plug-in that is intended for recording and replaying tests, which is how it differs from WebDriver. A code library of APIs for managing browsers is called Selenium WebDriver. Although Selenium WebDriver is more expensive and more powerful, Selenium IDE is easier to install and learn.
What programming languages are supported by Selenium?
Selenium supports multiple programming languages for automation testing and scripting. The most commonly used languages for writing Selenium WebDriver scripts are:
- Java: The most popular language for testing Selenium automation.
- Python: Known for its simplicity and readability, making it ideal for beginners.
- C#: Preferred in Microsoft environments for testing .NET applications.
- JavaScript: Used with Node.js for cross-platform testing.
- Ruby and Kotlin: Less commonly used but still supported for Selenium WebDriver testing.
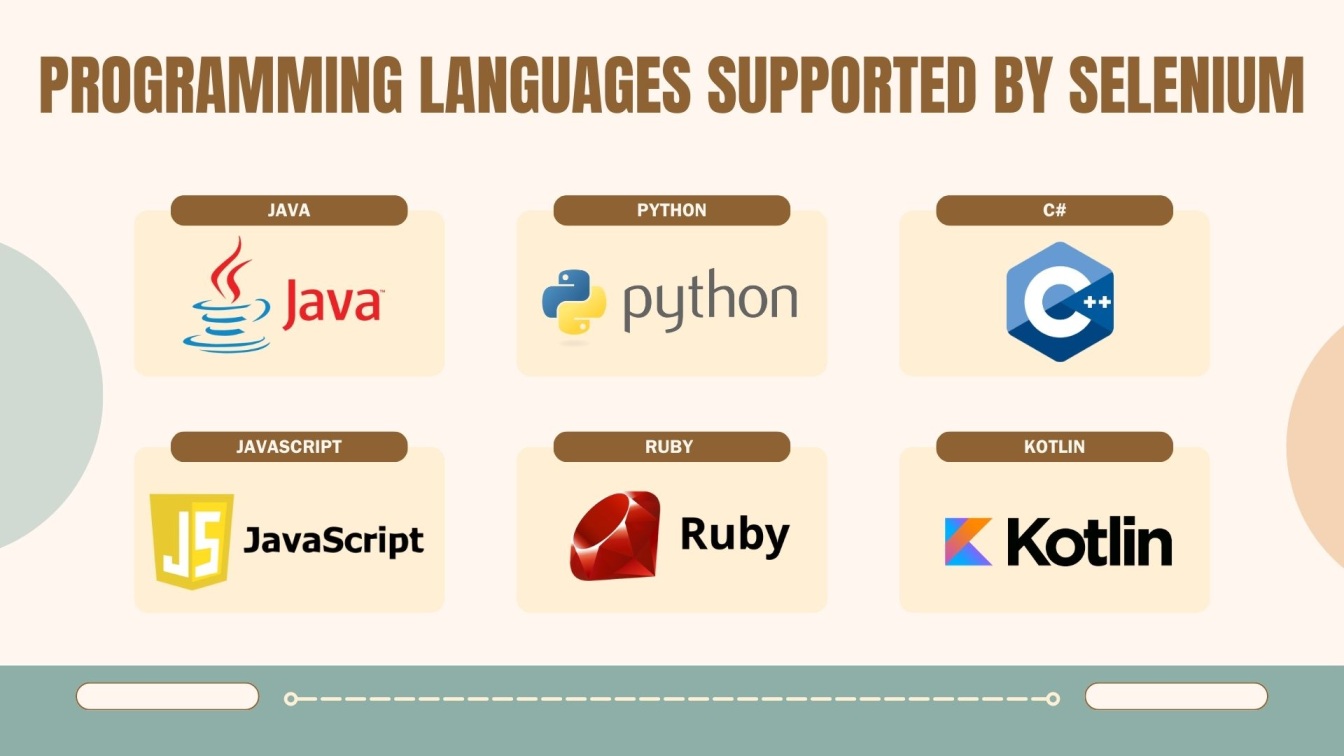
How do you locate an element using Selenium WebDriver?
Selenium WebDriver locates web elements using different locator strategies like ID, name, XPath, CSS selector, link text, and partial link text. These locators help identify unique elements on a web page, enabling automated testing and interaction with web applications.
What are the different types of locators in Selenium?
Here are the different types of locators in Selenium WebDriver:
- ID: Locates elements by their unique ID attribute.
- Name: Locates elements by their name attribute.
- Class Name: Locates elements by their class name attribute.
- Tag Name: Locates elements by their HTML tag name.
- Link Text: Locates anchor elements by their exact link text.
- Partial Link Text: Locates anchor elements by a partial match of their link text.
- CSS Selector: Locates elements using CSS selectors, providing flexibility in targeting elements.
- XPath: A powerful locator strategy that uses XPath expressions to navigate and locate elements within the HTML DOM.
Explain the difference between findElement() and findElements() methods.
- findElement() - Returns the first matching element.
- findElements() - Returns a list of all matching elements.
What is XPath, and how is it used in Selenium?
XPath is a language for navigating XML or HTML documents. In Selenium WebDriver, it's used to locate elements on a web page based on their attributes, text content, and relative positions. XPath provides flexibility and reliability, especially for dynamically generated elements. However, it's important to use XPath judiciously to avoid complex and brittle test scripts.
Differentiate between absolute and relative XPath
- Absolute XPath starts from the root node (`/html`) and traverses the entire DOM structure to locate the target element. It's less flexible as changes in the HTML structure can break the XPath.
- Relative XPath starts from a specific element and navigates relative to it, making it more resilient to DOM changes. It's often preferred due to its adaptability and shorter, more maintainable expressions.

What is the purpose of the get() and navigate() methods in WebDriver?
The get() method in WebDriver is used to load a specific URL into the browser. It takes a URL as an argument and opens the webpage in the browser.
The navigate() method offers various functionalities for browser navigation:
- navigate().to(url): Loads a new URL into the browser.
- navigate().back(): Navigates back to the previous page in the browser history.
- navigate().forward(): Navigates forward to the next page in the browser history.
- navigate().refresh(): Reloads the current page.
How do you handle dropdowns in Selenium WebDriver?
To handle dropdowns in Selenium WebDriver, you can use the Select class. This class provides methods to select options from a dropdown:

- selectByVisibleText(text): Selects an option by its visible text.
- selectByValue(value): Selects an option by its value attribute.
- selectByIndex(index): Selects an option by its index, starting from 0.
What is the use of the sendKeys() method in Selenium?
The sendKeys() method in Selenium WebDriver is used to simulate keyboard input into a web element. It takes a string as an argument and enters the characters into the specified element, often used for filling forms, searching, or interacting with text fields.
How can you perform mouse hover actions in Selenium?
Imagine you're using a mouse to hover over a button on a webpage. In Selenium, we can simulate this action using the Actions class.
- Create an action: We first create an Actions object.
- Identify the element: We locate the specific element (e.g., a button) where we want to hover.
- Perform the action: Using the moveToElement() method, we instruct Selenium to move the cursor over the specified element. Finally, perform() executes this action.

What are the different types of waits available in Selenium WebDriver?
Selenium WebDriver offers two main types of waits:
- Implicit Wait: Sets a default time for the WebDriver to wait for elements to load before throwing an exception.
- Explicit Wait: Allows you to create custom wait conditions for specific elements. You can define conditions like element visibility, invisibility, or presence. This provides more control over the waiting behavior.

What is Implicit Wait in Selenium?
Implicit wait sets a default time for WebDriver to wait for elements to load before throwing an exception. This helps avoid test failures due to slow-loading elements.
How do you handle Alerts and Pop-ups in Selenium?
Selenium handles alerts and pop-ups using methods like accept(), dismiss(), and getText(). These methods allow you to interact with the alert's content and perform actions like clicking "OK" or "Cancel."
What is the purpose of the switchTo() method in WebDriver?
The switchTo() method allows you to switch focus between different frames, windows, or iframes within a webpage. This is essential when dealing with complex web page layouts.
How can you handle Multiple Windows in Selenium?
To handle multiple windows, you can use methods like getWindowHandles() to get a list of open windows and switchTo().window(handle) to switch to a specific window.

What is TestNG, and how is it used with Selenium?
TestNG is a testing framework that provides features like annotations, test grouping, reporting, and more. It can be used with Selenium to create well-structured and organized test automation suites.

How do you capture Screenshots in Selenium WebDriver?
You can capture screenshots in Selenium using the TakesScreenshot interface. This is helpful for debugging and analyzing test failures.

What are the advantages of using Selenium for Automation Testing?
Selenium offers several advantages, including:
- Open-source: Free to use and widely supported.
- Cross-browser compatibility: Supports multiple browsers.
- Flexibility: Can automate various web application functionalities.
- Community support: A large and active community.
- Cost-effective: Reduces manual testing efforts and costs.
- Improved test reliability: Ensures consistent and reliable testing.
Intermediate Level Selenium Interview Questions
What is the Page Object Model (POM), and what are its advantages?
Page Object Model (POM) is a design pattern that organizes web page elements and their corresponding actions into reusable classes. This approach makes test automation code more organized, maintainable, and easier to understand.
Advantages of POM:
- Improved code organization: Clear separation of concerns.
- Enhanced code reusability: Reusable page objects across multiple tests.
- Better maintainability: Easier to update and modify test cases.
- Increased test stability: Reduced impact of UI changes on test cases.
- Improved test readability: More understandable and maintainable test code.
How do you implement the Page Factory in Selenium?
Page Factory is a feature in Selenium that simplifies the initialization of web elements. It automatically initializes elements using annotations like @FindBy. This reduces code redundancy and makes your test code more concise and readable.
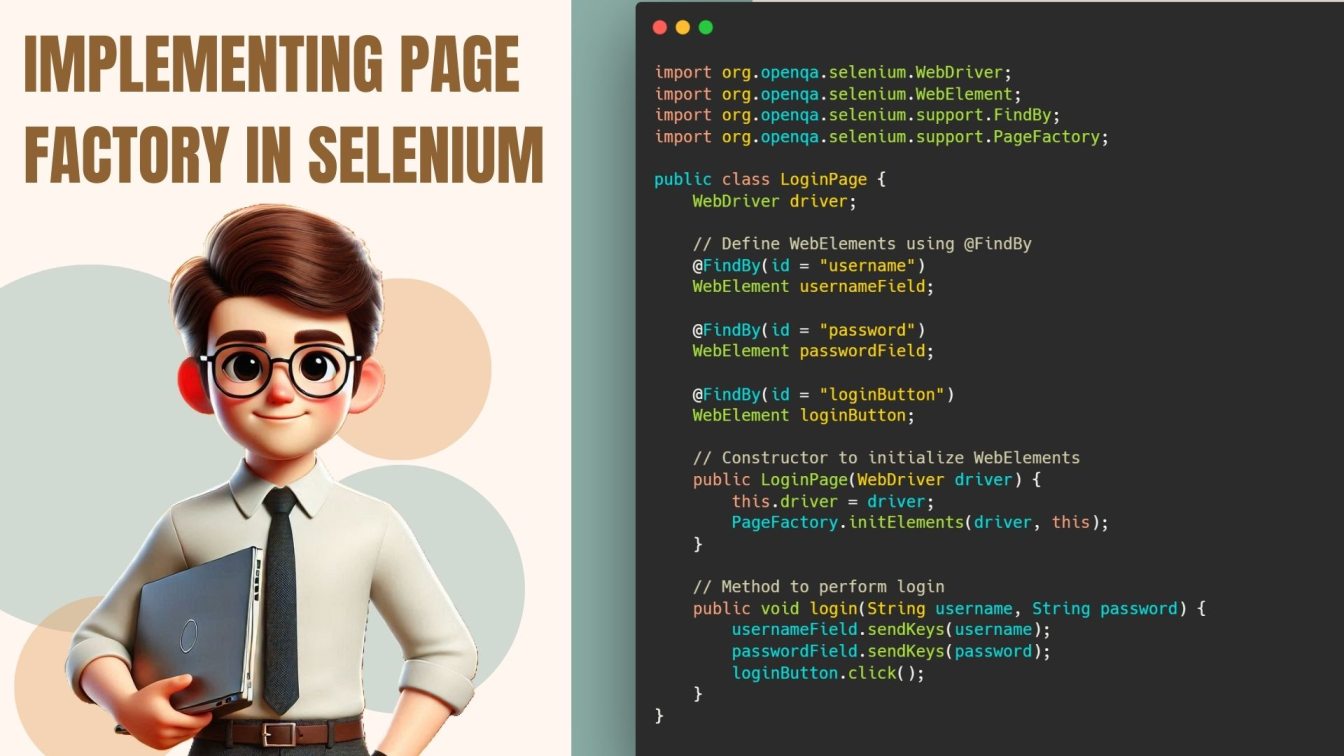
Explain the concept of data-driven testing in Selenium.
Data-driven testing is a testing approach where test cases are executed multiple times with different sets of data. This helps in testing various scenarios and edge cases, improving test coverage and reliability.
How can you read data from an Excel file in Selenium?
To read data from an Excel file in Selenium, you can use libraries like Apache POI or JXL. These libraries allow you to read data from Excel files and use them as input for your test cases. This is helpful for data-driven testing, where you can execute the same test case with multiple sets of data.
What is the difference between assert and verify commands in Selenium?
Assert commands immediately stop the test execution if the condition fails. Verify commands continue the test execution even if the condition fails, logging the failure for later analysis.
How do you handle dynamic web elements in Selenium?
Dynamic web elements are elements that change their attributes or position on the page. To handle them in Selenium, you can use techniques like explicit waits, dynamic locators (XPath or CSS selectors), and JavaScriptExecutor. These techniques help you locate and interact with dynamic elements even if their attributes change.
What are the common exceptions in Selenium WebDriver, and how do you handle them?
Common exceptions in Selenium WebDriver include NoSuchElementException, ElementNotVisibleException, ElementNotSelectableException, and TimeoutException. You can handle these exceptions using try-catch blocks, explicit waits, and error-handling mechanisms to make your tests more robust.
How can you perform drag-and-drop actions in Selenium?
To perform drag and drop actions in Selenium WebDriver, you can use the Actions class. By combining methods like clickAndHold(), moveToElement(), and release(), you can simulate the drag-and-drop behavior, making it suitable for testing interactive elements like calendars, file uploads, or other drag-and-drop functionalities on web pages
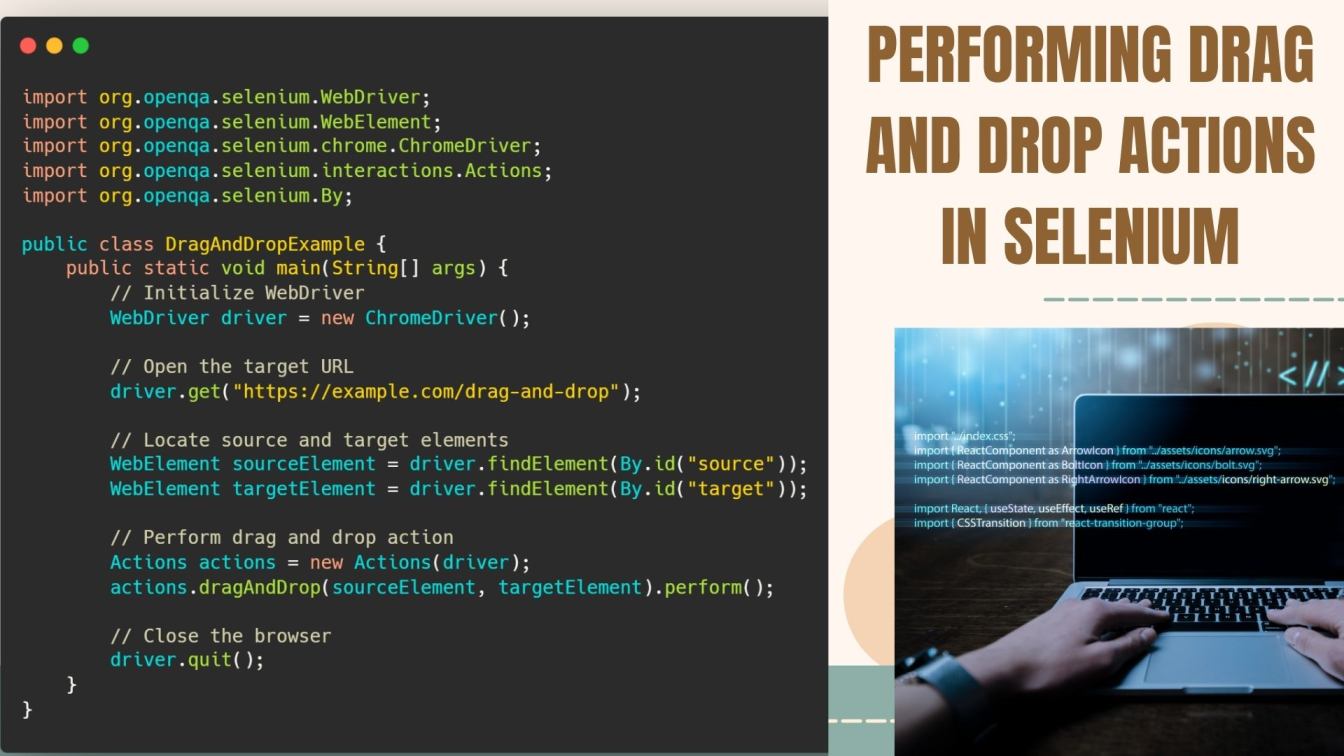
What is Selenium Grid, and how does it work?
Selenium Grid is a tool that allows you to distribute your Selenium tests across multiple machines and browsers. It helps in parallel test execution, reducing test execution time and maximizing test coverage. By setting up a Selenium Grid hub and nodes, you can run tests on different browsers and operating systems simultaneously, improving overall testing efficiency.
How do you execute tests in parallel using Selenium Grid?
To execute tests in parallel using Selenium Grid, you need to set up a Grid hub and register nodes with different browser and operating system configurations. Then, you can configure your test framework (like TestNG or JUnit) to distribute test cases across these nodes. This allows you to run multiple tests simultaneously, significantly reducing test execution time.
What are the desired capabilities in Selenium WebDriver?
Desired capabilities are a set of key-value pairs that define the browser, version, platform, and other specific settings for your Selenium WebDriver tests. By setting desired capabilities, you can customize the browser environment and ensure that your tests run on the desired configurations. This is particularly useful when running tests on a Selenium Grid, where you can specify different browser and platform combinations for parallel execution.
How do you handle SSL certificate errors in Selenium?
To handle SSL certificate errors in Selenium, you can use the DesiredCapabilities class to set the acceptInsecureCerts capability to true. This allows Selenium to bypass certificate validation and access the website, even if the certificate is not trusted. However, it's important to use this approach cautiously, as it can compromise security.
What is the role of the JavascriptExecutor in Selenium?
JavaScriptExecutor is an interface in Selenium that allows you to execute JavaScript code directly within the browser. This is particularly useful for handling dynamic elements, scrolling the page, clicking on hidden elements, and performing actions that are not directly supported by Selenium WebDriver's built-in methods. By executing custom JavaScript commands, you can gain more flexibility and control over browser interactions.
How can you perform file upload and download operations in Selenium?
To perform file upload operations in Selenium, you can use the sendKeys() method to directly input the file path into the file input element. For file download operations, you can induce the download using Selenium actions and then handle the downloaded file using automation tools or system-level interactions.
Explain the concept of a hybrid framework in Selenium.
A hybrid framework in Selenium combines the best aspects of different testing frameworks and methodologies. It typically involves a modular architecture, data-driven testing, keyword-driven testing, and a page object model. This approach promotes code reusability, maintainability, and flexibility, making it suitable for large-scale and complex test automation projects.
How do you manage dependencies in Selenium projects?
To manage dependencies in Selenium projects, you can use build tools like Maven or Gradle. These tools help automate the process of downloading and managing dependencies, ensuring that your project has the necessary libraries and their correct versions. By using a build tool, you can easily update dependencies, resolve conflicts, and maintain a clean and organized project structure.
What is the significance of the setProperty() method in Selenium?
The setProperty() method in Selenium WebDriver is used to set system properties that configure the WebDriver environment. It allows you to specify the browser driver path, browser version, and other relevant settings. By using setProperty(), you can customize the WebDriver behavior and ensure that your tests run on the desired browser and version.
How do you handle frames and iframes in Selenium?
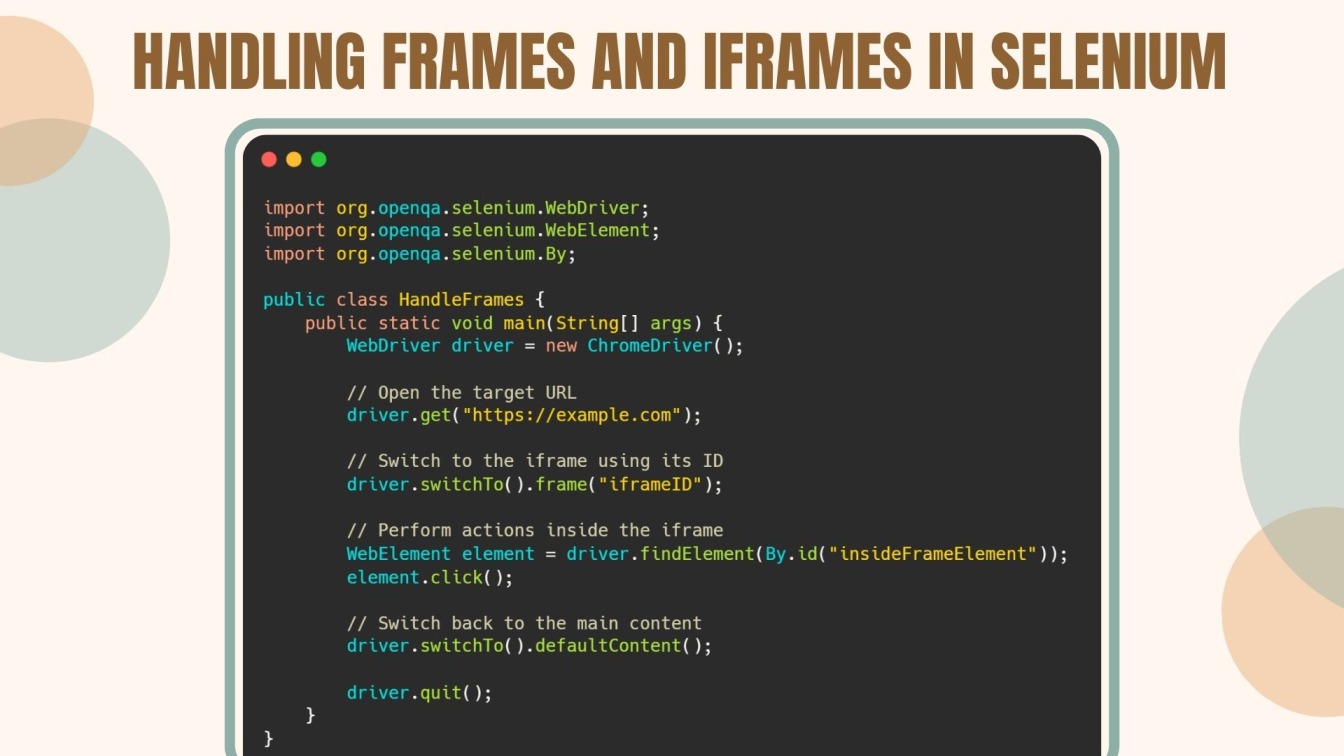
To handle frames and iframes in Selenium, you can use the switchTo() method. You can switch to a specific frame by its name, index, or WebElement. To switch back to the main window, use switchTo().defaultContent(). For nested iframes, you can use a combination of switchTo().frame() and switchTo().parentFrame().
hat are the limitations of Selenium WebDriver?
Selenium WebDriver is primarily designed for web application testing. It may not be suitable for testing non-web applications like desktop or mobile apps. Additionally, it can be challenging to handle dynamic web elements, asynchronous operations, and complex web applications that require advanced JavaScript interactions.
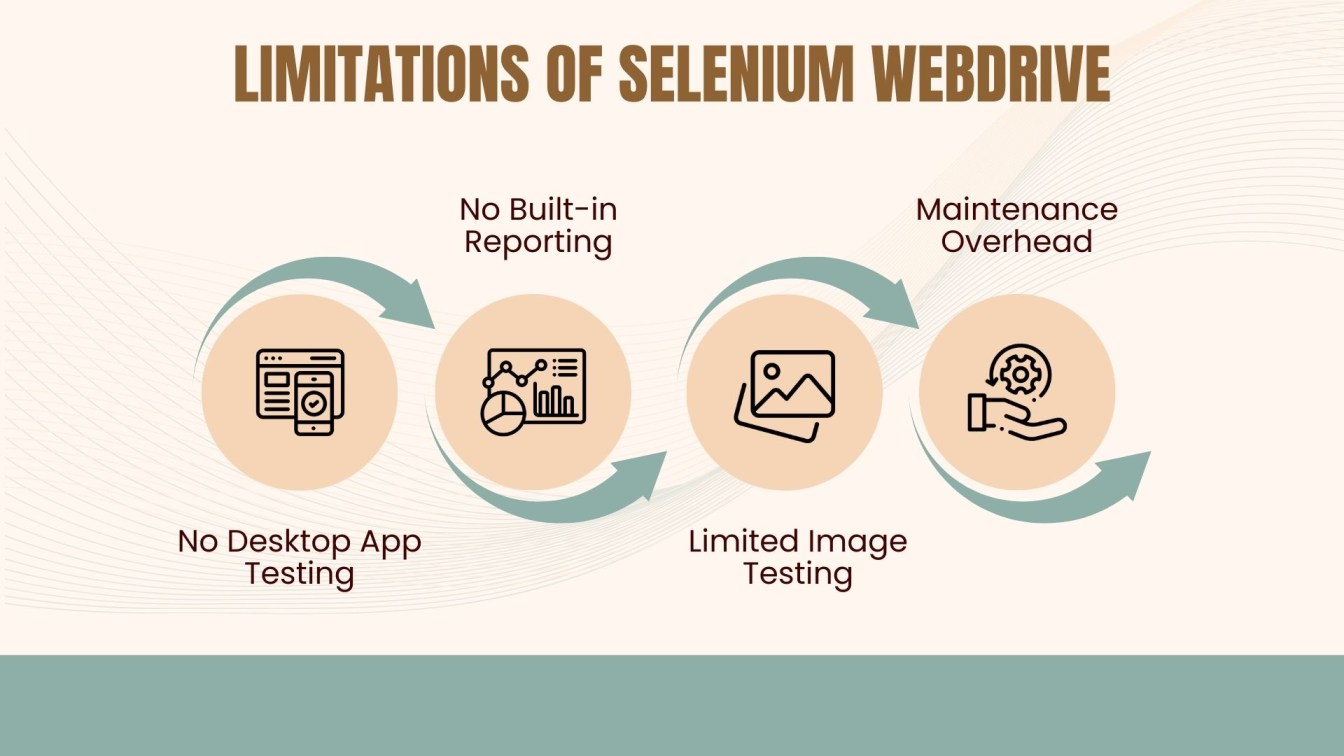
How do you integrate Selenium with continuous integration tools like Jenkins?
To integrate Selenium with Jenkins, you can create a Jenkins pipeline job that triggers your Selenium test suite. This involves setting up the necessary build steps, configuring the Selenium environment, and executing your tests. By integrating Selenium with Jenkins, you can automate the testing process, achieve continuous integration, and ensure faster feedback on code changes.
Advanced Level Selenium Interview Questions
How do you handle AJAX calls in Selenium WebDriver?
To handle AJAX calls in Selenium WebDriver, you can use explicit waits or implicit waits to wait for dynamic content to load. Explicit waits allow you to define custom conditions for waiting, while implicit waits set a global timeout for all elements. Additionally, you can use JavaScriptExecutor to execute JavaScript commands and check for dynamic content changes.
What is Fluent Wait, and how is it different from Explicit Wait in Selenium?
Fluent Wait is a more flexible and customizable approach to waiting for elements. Unlike Explicit Wait, it allows you to set multiple conditions and polling intervals, making it ideal for handling dynamic web elements and asynchronous operations. Fluent Wait can be configured to retry the condition multiple times within a specified time frame, increasing the chances of locating the element.
How can you achieve cross-browser testing using Selenium?
To achieve cross-browser testing using Selenium, you can configure your test environment to run tests on multiple browsers and operating systems. You can use tools like Selenium Grid or cloud-based testing platforms to execute your tests on different browser-OS combinations. This helps ensure that your web application works correctly across various browsers and devices.
Explain the architecture of Selenium WebDriver.
Selenium WebDriver follows a client-server architecture. The Selenium client libraries (like WebDriver) act as clients that communicate with the WebDriver server. The server, in turn, interacts with the browser driver to control the browser's actions. This architecture enables cross-browser testing and allows you to write test scripts in various programming languages.
How do you handle authentication pop-ups in Selenium?
To Handle Auth Pop-ups:
- Pass Credentials in URL: https://username:password@website.com.
- Use Alert Interface: driver.switchTo().alert().accept().
- ChromeOptions: Disable pop-ups via browser options.
- Use AutoIT/Robot Class: Automate pop-ups WebDriver can't handle.

What is the difference between Selenium 3 and Selenium 4?
Selenium 4 introduced significant improvements over Selenium 3, including a more stable WebDriver API, enhanced browser compatibility, and improved support for modern web technologies. It also offers new features like relative locators, W3C WebDriver conformance, and better error-handling mechanisms, making it a more robust and efficient tool for web automation.
How do you implement custom expected conditions in Selenium?
To implement custom expected conditions in Selenium, you can create a class that implements the ExpectedCondition interface. This interface requires you to define a method that returns a boolean value indicating whether the condition is met. You can then use this custom condition with WebDriverWait to wait for specific elements or page states.
What are the best practices for writing Selenium test scripts?
The best practices for writing Selenium test scripts include using the Page Object Model (POM), handling dynamic waits with Explicit Waits, and maintaining clean, reusable code. Following these practices ensures better test automation, enhanced script maintainability, and efficient Selenium WebDriver testing.
How do you handle browser-specific settings in Selenium?
To handle browser-specific settings in Selenium, you can use Desired Capabilities. By setting specific capabilities, you can configure the browser's behavior, such as enabling or disabling JavaScript, setting the browser window size, or specifying the browser version. This allows you to tailor your tests to specific browser configurations and ensure compatibility.
What is the role of listeners in TestNG, and how do you use them with Selenium?
Listeners in TestNG provide a way to customize the behavior of your test execution. They allow you to perform actions before or after a test, test suite, or test class. In Selenium, you can use listeners to generate custom reports, take screenshots on test failure, send email notifications, or integrate with other tools. By implementing custom listeners, you can enhance the reporting and analysis capabilities of your Selenium tests.
How can you perform database testing using Selenium?
While Selenium WebDriver is primarily for web application testing, you can indirectly test database interactions by verifying the UI changes resulting from database operations. You can also use tools like JUnit and TestNG to write database test cases and integrate them with your Selenium tests. This allows you to ensure that database operations are performed correctly and that the UI reflects the expected database state.
What is the significance of the proxy settings in WebDriver?
Bypass firewalls: Proxy settings can help you bypass firewalls and network restrictions, allowing you to access websites that might be blocked.
Anonymize traffic: Using a proxy server can mask your IP address, making it harder to track your online activity.
How do you handle file downloads in headless browser mode using Selenium?
You can handle file downloads in headless browser mode using Selenium WebDriver by configuring the browser's download preferences using ChromeOptions or FirefoxOptions. Set the desired download directory and enable automatic downloads to avoid pop-ups.
Explain the concept of session handling in Selenium WebDriver.
Session handling in Selenium WebDriver involves maintaining a unique session for each browser instance. Selenium assigns a session ID to track interactions, ensuring commands are executed in the correct browser session during automation testing.
How do you manage test data in Selenium frameworks?
To manage test data in Selenium frameworks, use Excel, CSV, JSON, or databases to store dynamic data and implement data-driven testing with TestNG or JUnit. This ensures efficient Selenium automation testing with reusable and maintainable test scripts.
What are the challenges you have faced while working with Selenium, and how did you overcome them?
Common challenges in Selenium automation testing include handling dynamic web elements, managing synchronization issues, and working with browser compatibility. These were overcome using Explicit Waits, robust XPath/CSS Selectors, and cross-browser testing with Selenium Grid. Effective use of frameworks like TestNG and proper exception handling ensures smooth test execution
How do you ensure the maintainability of Selenium test suites?
To ensure the maintainability of Selenium test suites, it's essential to follow best practices that promote reusability, scalability, and ease of updates. Here are key strategies:
- Use POM for structured, maintainable scripts.
- Create Reusable Methods to avoid redundancy.
- Apply Data-Driven Testing for dynamic data.
- Optimize Locators for handling dynamic elements.
- Use CI/CD Tools for continuous testing and updates.
What is the role of the RemoteWebDriver class in Selenium?
The RemoteWebDriver class in Selenium enables the execution of test scripts on remote machines using Selenium Grid. It allows for cross-browser testing across multiple platforms, ensuring distributed testing and faster execution.
How do you handle browser notifications in Selenium?
To handle Browser notifications:
- Use ChromeOptions: Disable notifications using options.addArguments("--disable-notifications").
- Use FirefoxOptions: Set preferences with options.setPreference("dom.webnotifications.enabled", false).
- Handle Pop-ups with Alert: Use driver.switchTo().alert().dismiss() for notification pop-ups.

Explain how you can find broken links on a page using Selenium WebDriver
To Handle Broken Links:
- Extract All Links: Use driver.findElements(By.tagName("a")) to get all page links.
- Check HTTP Status: Use HttpURLConnection to verify each link's HTTP response code.
- Validate Status Code: Ensure status is 200 (OK) to confirm the link is working.
- Report Broken Links: Log or report links with 4xx or 5xx errors.
Conclusion
This guide has equipped you with the knowledge to excel in Selenium testing interviews, covering essential topics like Selenium WebDriver, element locators, and handling current browser windows. Whether it's automating web-based applications, creating efficient Selenium scripts, or using frameworks like data-driven or keyword-driven frameworks, mastering these concepts ensures success in Selenium automation testing.
By understanding techniques like handling desktop applications, managing driver objects, and implementing cross-browser testing, you'll be ready to tackle real-world challenges. From handling radio buttons and dropdowns to using external files and optimizing the root element with absolute and relative paths, Selenium provides robust tools for automation. For advanced scenarios, using third-party tools, avoiding code duplication, and incorporating source code with code snippets make your approach more professional.
Whether working with Selenium IDE for simpler tasks or handling double slashes in XPath, this comprehensive knowledge ensures you're prepared for any challenge. Keep refining your skills, and let Selenium drive your success!
People Also Ask
👉Is Selenium a tool or API?
Selenium is a tool that provides an API for automating software applications such as web-based and mobile applications.
👉Is Selenium part of Python?
Selenium is not part of Python but integrates seamlessly through its Python library, enabling tasks like functional testing and data-driven frameworks.
👉Does Selenium IDE require coding?
No, Selenium IDE is a record-and-playback tool that doesn't require coding but can support complete path automation for simple software applications.
👉Is Selenium used for manual or automation?
Selenium is exclusively used for automation testing, especially for functional testing, cross-browser testing, and resolving compatibility issues.
👉Is Selenium Java or JavaScript?
Selenium supports both Java (e.g., using public void or static void methods) and JavaScript, but it is primarily a tool designed to automate software applications.