Data-driven testing (DDT) stands out for its efficiency and flexibility, especially for REST API testing. By structuring your tests to run with multiple sets of data, you can cover a wider range of test cases and improve the accuracy of API testing.
With REST Assured, a popular open-source Java library for API testing, data-driven testing becomes a powerful and maintainable testing process.
In this blog, we’ll cover:
📌Data-Driven Testing: Understand its significance in API testing. 📊
📌Setting Up REST Assured: Configure for effective REST Assured API testing. ⚙️
📌Data Sources: Use Excel and JSON files to parameterize tests. 📁
📌Implementing Tests: Create and execute data-driven tests with TestNG. 🧪
📌Best Practices: Explore tips for efficient API testing. 📝
Understanding Data-Driven Testing
Data-driven testing is a powerful technique where test data is externalized, allowing multiple test scenarios to be executed with different data sets. This method enhances test coverage, minimizes redundancy, and supports comprehensive validation by automating tests with varying input combinations and expected outcomes.
What is Data-Driven Testing?
Data-driven testing is a testing methodology where test cases are executed multiple times with different sets of input data. Instead of hardcoding values directly in the test code, you store input data in external files, such as Excel, CSV, or JSON.
This allows for greater flexibility and reusability of test scripts. By running tests with varied inputs, you can validate the behavior of APIs under different conditions, ensuring that they handle all scenarios effectively.
In REST API testing, data-driven testing helps ensure that APIs respond correctly to a wide range of inputs, thus increasing the accuracy of API testing. It enables you to automate repetitive tests and reduces the need to rewrite test cases.

Benefits of Data-Driven Testing in API Automation
Data-driven testing offers numerous advantages for API automation:
- Improved Test Coverage: Running tests with multiple sets of input data helps identify edge cases and bugs that may not be apparent with a single data set.
- Enhanced Maintainability: Since test data is separated from the test logic, making changes to the data does not require rewriting the actual test code. This leads to a more maintainable testing process.
- Increased Reusability: You can use the same test logic across different data sets, making your tests more versatile and easier to manage.
- Efficient API Test Automation: By automating data-driven tests, you can quickly validate various API endpoints without the need for manual intervention, which saves time and effort.
- Seamless Integration with CI/CD Pipelines: Data-driven tests can be integrated into continuous integration/continuous deployment (CI/CD) pipelines, ensuring that your APIs are consistently tested with every code change.
With these benefits in mind, let’s move on to setting up REST Assured for data-driven testing!
Setting Up REST Assured for Data-Driven Testing
Before building data-driven tests with REST Assured, setting up the right environment is essential. This involves integrating REST Assured into a project, ensuring dependencies are included, and configuring test frameworks like TestNG or JUnit.
Prerequisites for Using REST Assured
Before diving into REST Assured, ensure you have the following prerequisites:
- Java Development Kit (JDK): REST Assured is a Java-based library, so make sure you have JDK 8 or higher installed on your machine.
- Maven Project: REST Assured relies on Maven to manage dependencies. If you don’t have a Maven project set up, you can easily create one.
- Integrated Development Environment (IDE): Using an IDE like IntelliJ IDEA or Eclipse will help streamline your development and testing process.
- Basic Understanding of REST APIs: Familiarity with RESTful principles and HTTP methods will be beneficial as you implement your API tests.
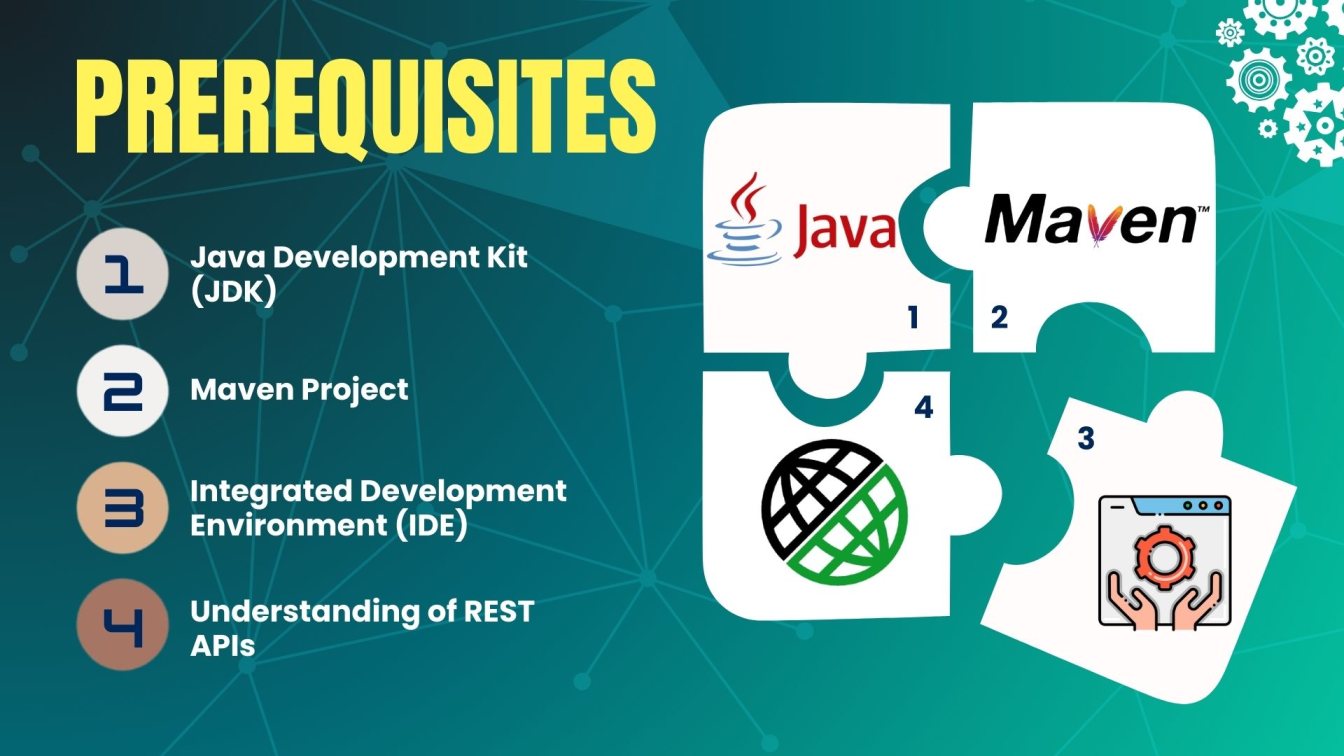
Installing Required Dependencies in Maven
To use REST Assured, you need to add the necessary dependencies to your pom.xml file. Below is an example of the dependencies required for REST Assured, TestNG, and Apache POI (for handling Excel files):
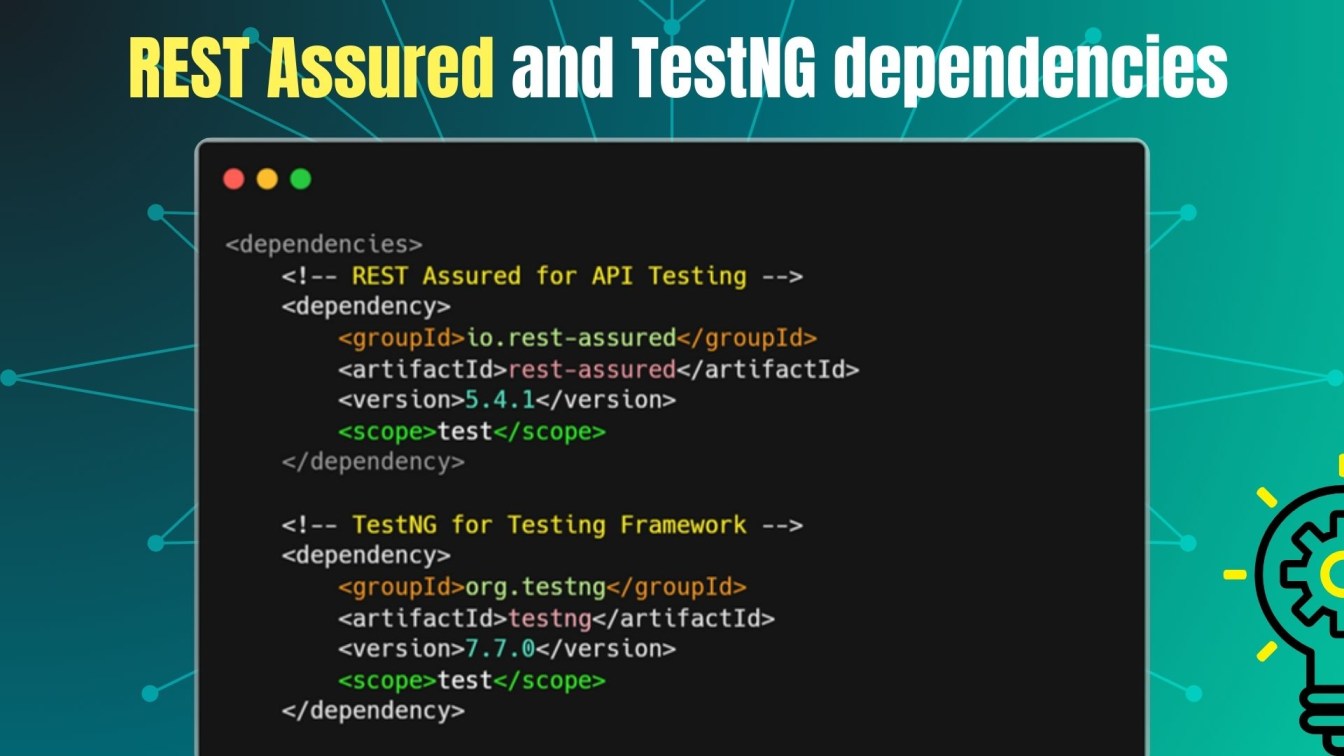

This configuration allows you to leverage REST Assured for API tests and Apache POI for reading test data from Excel files. With these dependencies in place, you're ready to start building your data-driven testing framework!
Creating a Data-Driven Test Framework with REST Assured
A well-structured framework facilitates reusable and scalable data-driven testing. This section focuses on designing a framework where REST Assured handles API requests and external sources like CSV, Excel, or databases provide the input data, enabling automated test iterations and simplified maintenance.
Structuring a Data-Driven Test Framework
Creating a well-structured framework is crucial for organizing your tests and ensuring maintainability. A typical project structure for a data-driven testing framework using REST Assured could look like this:

- src/main/java: Contains your application logic, if necessary.
- src/test/java: Holds your test classes and helper methods.
- src/test/resources: Stores external data files, such as Excel and CSV files, for test data.
- pom.xml: Manages your Maven dependencies.
This structure allows you to keep your actual test code organized and facilitates easy access to your test data files.
Configuring Test Data Sources (Excel, CSV, JSON)
To implement data-driven testing effectively, you'll need to configure various data sources. REST Assured can work seamlessly with different file formats:
- Excel: Great for structured data where you need to manage large datasets. Reading Excel files can be efficiently managed using Apache POI.
- CSV: Suitable for simpler datasets, CSV files are easy to manage and can be read using libraries like OpenCSV.
- JSON: Ideal for hierarchical or nested data. You can easily parse JSON files using libraries like Gson or Jackson.
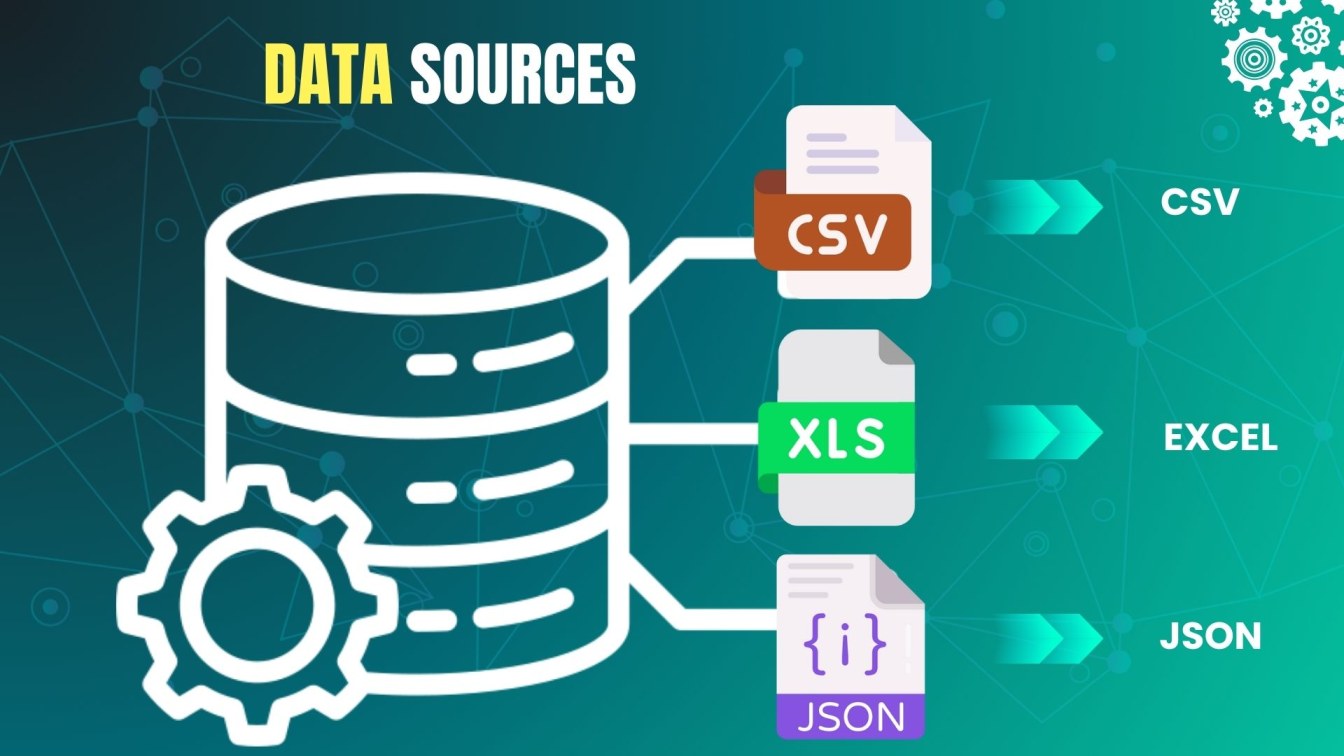
Now that we’ve covered the framework setup, let’s dive into how to use these external data sources in REST Assured tests.
Using External Data in REST-Assured Tests
Integrating external data sources with REST Assured is key to making your tests truly data-driven. Learn how to import data from spreadsheets, JSON files, or databases to execute tests dynamically. This practice allows for extensive test coverage with various input combinations, making testing more flexible and robust.
Reading Data from Excel Files
Reading data from Excel files allows you to manage complex datasets effectively. Let’s create a utility class that will handle reading data from an Excel file. Below is an example of how to read data from an Excel file using Apache POI:
Example Excel File
Imagine you have an Excel file named TestData.xlsx with the following structure:

Java Code for Reading Excel Data
Here’s how to implement the code to read data from the Excel file:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
public class ExcelUtils {
public static Object[][] excelData(String filePath, String sheetName) {
Object[][] data = null;
try (FileInputStream fileStream = new FileInputStream(new File(filePath));
Workbook workbook = new XSSFWorkbook(fileStream)) {
Sheet sheet = workbook.getSheet(sheetName);
int row_Count = sheet.getPhysicalNumberOfRows();
int col_Count = sheet.getRow(0).getPhysicalNumberOfCells();
data = new Object[row_Count - 1][col_Count];
for (int i = 1; i < row_Count; i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < col_Count; j++) {
Cell cell = row.getCell(j);
data[i - 1][j] = getCellValue(cell);
}
}
} catch (Exception e) {
System.err.println("Error reading Excel file: " + e.getMessage());
e.printStackTrace();
}
return data;
}
private static Object getCellValue(Cell cell) {
if (cell == null) {
return "";
}
switch (cell.getCellType()) {
case STRING:
return cell.getStringCellValue();
case NUMERIC:
if (DateUtil.isCellDateFormatted(cell)) {
return cell.getDateCellValue();
} else {
return cell.getNumericCellValue();
}
case BOOLEAN:
return cell.getBooleanCellValue();
case FORMULA:
return cell.getCellFormula();
default:
return "";
}
}
}
Using CSV Files for Test Data
CSV files are simple to work with in data-driven testing. Libraries like OpenCSV make reading CSV files convenient. Below is a practical example to illustrate this process.
Example CSV File
You might have a file called TestData.csv structured like this:

Java Code for Reading Data from a CSV File
Below is a method to read data from a CSV file:
.jpeg)
Configuring JSON Data Sources
JSON files are another excellent option for structuring test data. They are particularly useful for complex datasets with nested properties.
Example JSON File
Consider a JSON file called TestData.json structured like this:

Java Code for Reading JSON Data
You can use libraries like Gson to read JSON data easily:
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import java.io.FileReader;
import java.util.List;
public class JSONUtils {
public static List<User> getJSONData(String filePath) {
try (FileReader reader = new FileReader(filePath)) {
Gson gson = new Gson();
return gson.fromJson(reader, new TypeToken<List<User>>() {}.getType());
} catch (Exception e) {
System.err.println("Error reading JSON file: " + filePath + " - " + e.getMessage());
e.printStackTrace();
return null;
}
}
public static class User {
private int userId;
private String expectedTitle;
// Getters and Setters
public int getUserId() {
return userId;
}
public void setUserId(int userId) {
this.userId = userId;
}
public String getExpectedTitle() {
return expectedTitle;
}
public void setExpectedTitle(String expectedTitle) {
this.expectedTitle= expectedTitle;
}
}
}
Implementing Data-Driven Tests with REST Assured
This section will cover practical examples of creating and running data-driven tests in REST Assured. It will involve step-by-step guidance on reading data from external sources and feeding it into test scripts to execute different scenarios in one go, ensuring better automation practices.
Parameterizing API Requests
With our data sources configured, we can now implement data-driven tests in REST Assured. Here’s an example of how to parameterize your API requests using data from an Excel file:
import io.restassured.RestAssured;
import io.restassured.response.Response;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import static org.hamcrest.Matchers.equalTo;
import static org.testng.Assert.assertEquals;
public class ApiTest {
@DataProvider(name = "excelData")
public Object[][] excelDataProvider() {
return ExcelUtils.excelData("src/test/resources/TestData.xlsx", "Sheet1");
}
@Test(dataProvider = "excelData")
public void validateTitle(int userId, String expectedTitle) {
RestAssured.baseURI = "https://api.example.com";
Response response = RestAssured.given()
.pathParam("userId", userId)
.when()
.get("/users/{userId}");
// Validate the response
response.then().assertThat()
.statusCode(200)
.body("title", equalTo(expectedTitle));
}
@Test(dataProvider = "excelData")
public void validateApiResponse(int userId, String expectedTitle) {
Response response = RestAssured.given()
.pathParam("userId", userId)
.when()
.get("/users/{userId}");
// Assert the response code and body
assertEquals(response.getStatusCode(), 200);
assertEquals(response.jsonPath().getString("title"), expectedTitle);
}
@Test(dataProvider = "excelData")
public void logTestResults(int userId, String expectedTitle) {
Response response = RestAssured.given()
.pathParam("userId", userId)
.when()
.get("/users/{userId}");
// Log results
System.out.println("Testing User ID: " + userId);
System.out.println("Expected Title: " + expectedTitle);
System.out.println("Response Code: " + response.getStatusCode());
System.out.println("Actual Title: " + response.jsonPath().getString("title"));
}
}
Using TestNG for Data-Driven Testing with REST Assured
The TestNG framework is a powerful testing framework that integrates seamlessly with REST Assured. By utilizing TestNG’s data providers and TestNG annotations, you can easily implement data-driven tests without repetitive code. The above example demonstrates how to use the @DataProvider annotation to supply data from an Excel file to the test method.
This approach ensures that each test run utilizes different sets of input, allowing you to validate the API’s response accurately.
Validating API Responses in Data-Driven Tests
Validating responses accurately is a crucial part of any test. Here is how REST Assured can be used to assert response codes, response bodies, headers, and more, even in data-driven tests. This ensures that each test case verifies API behavior against expected outcomes effectively.
Handling Response Assertions for Multiple Data Sets
Validating the response is a critical step when performing API tests. REST Assured provides a fluent API for asserting various response attributes. Here’s how to validate the response body, response code, and response status code for different input sets:

In this example, we validate the response code and body for each data set provided by the data provider. This approach ensures that the API behaves as expected across multiple scenarios, enhancing the reliability of your tests.
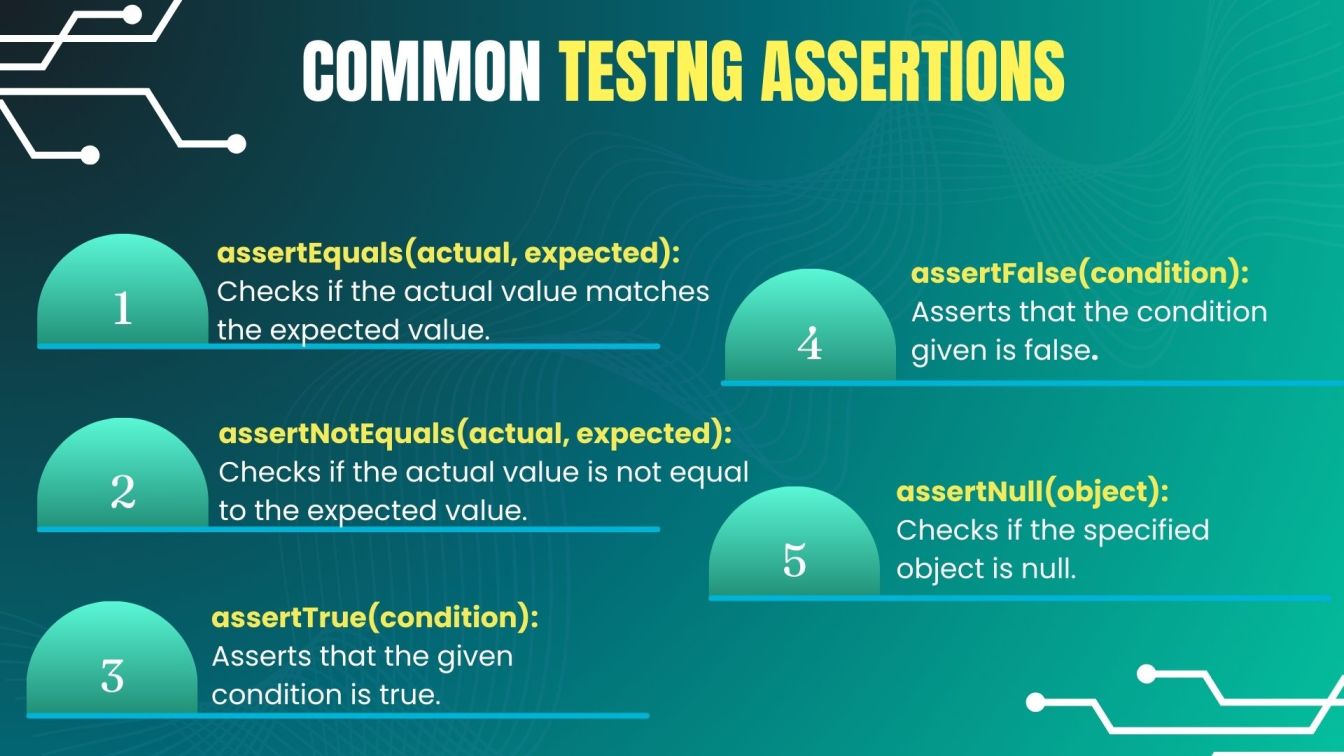
Logging Test Results for Different Data Scenarios
To effectively track the outcomes of your data-driven tests, consider logging the results for different scenarios. You can use TestNG’s listeners or custom logging to capture important details about each test run.

By logging test results, you can easily review the behavior of your API and identify any discrepancies that may require further investigation.
Best Practices for Data-Driven Testing with REST Assured
Organizing Test Data for Reusability
When managing multiple test data files, organizing your test data is key to maintaining a clean project structure. Consider creating separate packages for different data sources or types of tests. This organization promotes reusability and reduces confusion when accessing test data.
Tips for Efficient Data-Driven API Testing
- Keep Data Sources Simple: Use simple formats for your test data files (like CSV) when possible, as they are easier to read and maintain.
- Use Meaningful Test Data: Ensure your test data represents real-world scenarios to improve the accuracy of your tests. This will help you discover potential issues more effectively.
- Automate Test Execution: Integrate your data-driven tests into your CI/CD pipeline to automate execution with every code change. This practice allows for continuous validation of your APIs.
- Log and Report Results: Capture and report the results of your tests for better visibility and analysis. This information can help your team make informed decisions regarding the API’s stability and performance.
- Regularly Review and Update Test Data: As your application evolves, regularly review and update your test data to ensure it remains relevant. Outdated test data generation can lead to misleading results.
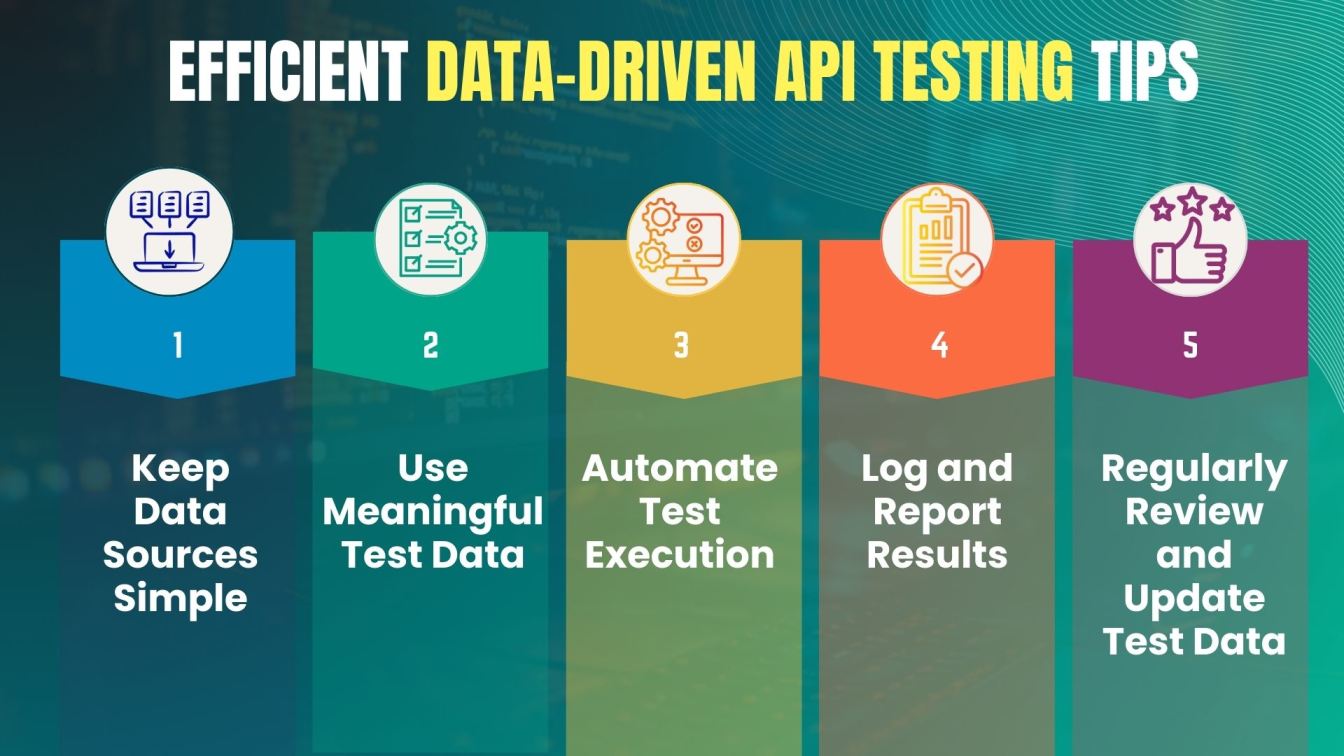
Conclusion
Data-driven API testing is a powerful methodology that enhances the efficiency and effectiveness of your testing efforts. By using REST Assured, you can create a robust and maintainable testing framework that handles various input scenarios seamlessly. 🌟
In this blog, we've explored the fundamentals of data-driven testing, how to set up REST Assured, implement data-driven tests, and best practices for efficient API testing. By following these guidelines and utilizing the examples provided, you can improve your API test automation process and ensure the reliability of your APIs.
Happy testing! 🛠️✨

People also asked
👉How Does data-driven testing improve API test coverage?
It allows running the same test with multiple data sets, uncovering edge cases, and ensuring thorough validation of different input scenarios, which enhances overall test reliability.
👉Do I need TestNG to perform data-driven testing with REST Assured?
No, TestNG is not mandatory but provides convenient features for data-driven testing. You can also use JUnit or create custom data-handling solutions.
👉What are some best practices for organizing test data in data-driven testing?
Organize test data by storing it in external files, using clear and consistent naming, and avoiding hard coding to ensure maintainability and flexibility.
👉Can I combine data-driven tests with other testing frameworks like Cucumber?
Yes, Cucumber supports data-driven testing using scenario outlines, making it easy to integrate REST Assured framework for comprehensive BDD testing.
👉Is it possible to use REST Assured for testing SOAP APIs?
Yes, REST Assured can be used for testing SOAP APIs with additional configurations to handle XML responses and SOAP-specific headers.