In the world of API testing, effective handling of authentication mechanisms is critical to ensure the security and integrity of the data being exchanged. For working with RESTful APIs or using tools like Rest Assured to automate testing, understanding how to manage various authentication types such as OAuth and JWT is essential.
In this blog, we will cover the fundamentals of authentication, explore different methods like OAuth, JWT, Basic Authentication, and API Keys, and show how to handle them effectively in Rest Assured. Among the top 10 automation testing tools, Rest Assured stands out for its ability to handle complex authentication processes like OAuth and JWT, making it a go-to tool for API testing. REST services provide a standardized way for applications to communicate over the web, using HTTP requests to perform operations on resources, often requiring robust authentication mechanisms.
📌 Why Read This Blog?
- Learn how to handle various authentication methods in Rest Assured for secure API testing.
- Discover practical examples for OAuth, JWT, and more, integrated with top automation testing tools.
- Explore best practices for securing your API tests and managing authentication tokens.
- Understand how API automation testing tools can enhance your test coverage and efficiency.
📌 What You'll Discover:
- How to automate OAuth and JWT authentication in Rest Assured?
- Tips for securely managing API keys and tokens.
- The role of automation solutions in streamlining secure API testing.
Introduction to Authentication in API Testing
API testing forms the backbone of modern software applications, helping ensure functionality, security, and performance across endpoints. One of the key elements in API testing is authentication, which acts as a gatekeeper to access protected resources. Before diving into how to manage authentication using Rest Assured, let’s first discuss why authentication is so important.
Authentication ensures that only authorized users or systems can access sensitive resources. With the rise of automation testing tools, incorporating authentication handling into your test cases becomes essential for end-to-end testing. REST API testing involves validating various aspects of API functionality, including authentication mechanisms like OAuth and JWT, to ensure secure and reliable interactions.
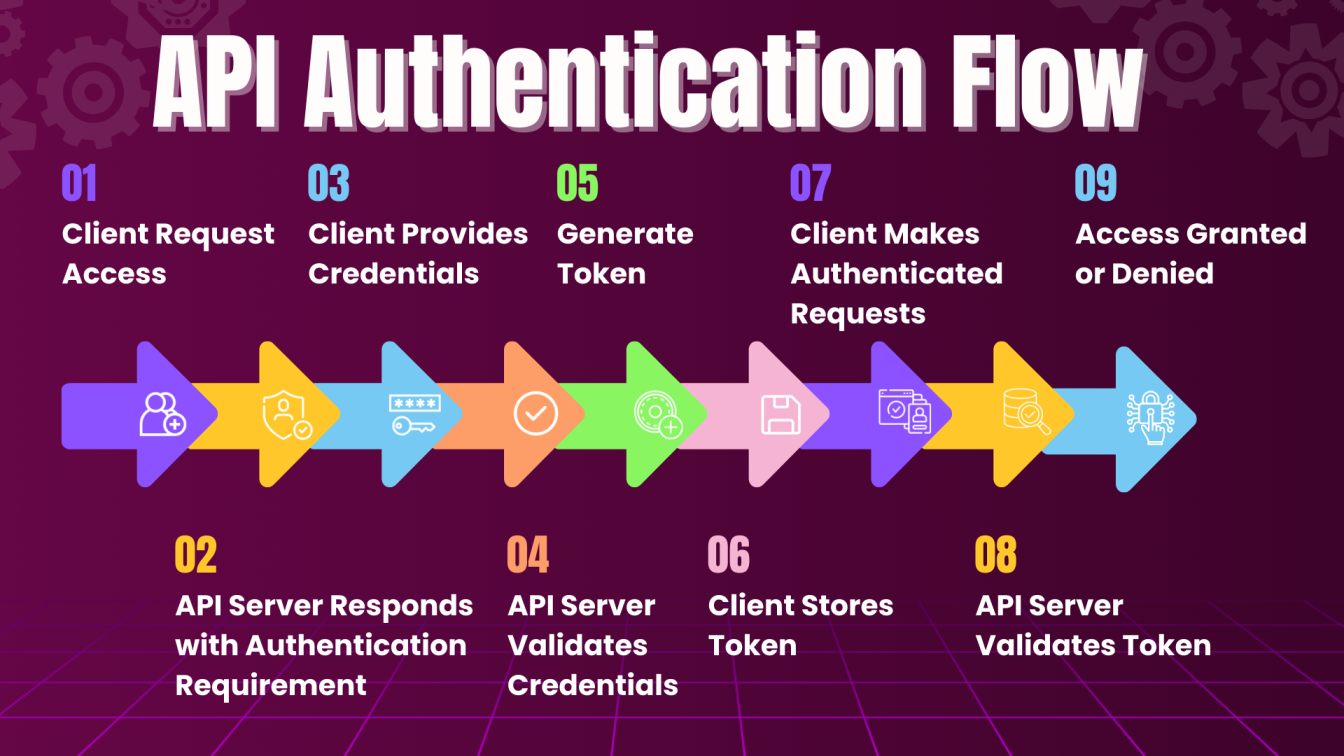
Common Authentication Types
In the context of APIs, several authentication types exist, each serving different purposes and offering various security levels. Selecting the best automation testing tools ensures that your API tests are not only efficient but also scalable, allowing seamless integration with various authentication mechanisms like OAuth and JWT.
Selecting the right authentication scheme, for Basic Authentication, OAuth, or JWT, is crucial for securing APIs, and Rest Assured makes it easy to test these schemes effectively. When testing APIs, the client application needs to authenticate itself using schemes like OAuth or JWT to securely access protected resources, and Rest Assured helps automate this process effectively.
Integrating third-party applications often requires robust authentication mechanisms like OAuth, allowing these applications to securely access user data without compromising security. Authentication details, including client IDs, secrets, and access tokens, are essential for verifying user identity and ensuring secure access to protected resources in API interactions. Several widely used types of authentication include:
- Basic Authentication
- OAuth 1.0 and OAuth 2.0
- JWT (JSON Web Tokens)
- API Key Authentication
- Bearer Token Authentication
Each method serves specific use cases, and with API automation testing tools like Rest Assured, you can easily incorporate these into your test automation suites. Authentication credentials, such as usernames, passwords, and access tokens, are vital for securing API endpoints and ensuring that only authorized clients can access sensitive data.
The response body contains the data returned by the API after processing a request, which can include user information or error messages related to authentication. A user session is initiated upon successful authentication, allowing users to interact with the API securely until the session expires or is terminated.
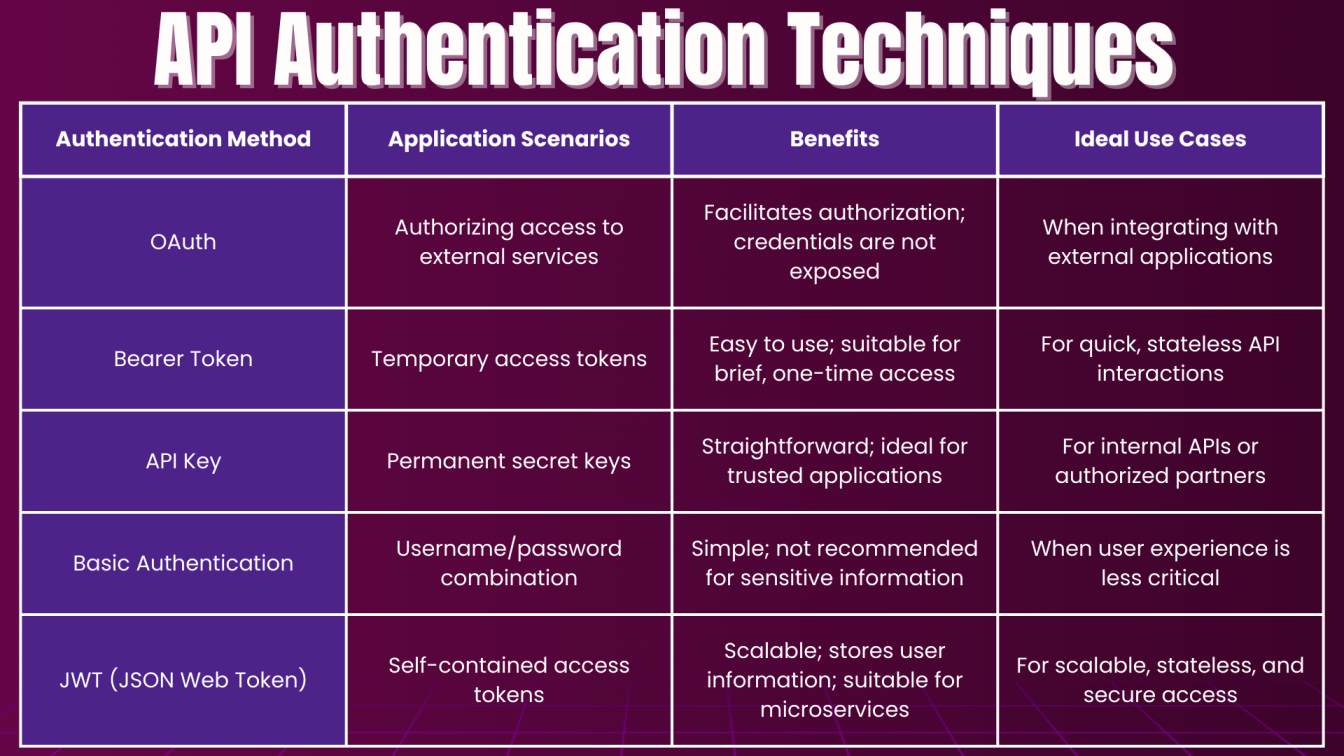
Basic Authentication in Rest Assured
Basic authentication is one of the simplest forms of authentication and is still supported by many APIs. Using automation testing tools, such as Rest Assured, you can easily test APIs secured with basic authentication. Incorporating this example into your automation software testing tools ensures you have end-to-end test coverage for even the simplest authentication mechanisms.
With the latest automation testing tools, testers can efficiently handle modern authentication methods like OAuth and JWT, simplifying the process of securing API endpoints. The best automation testing software, such as Rest Assured, enables seamless handling of authentication protocols like OAuth and JWT, ensuring that API tests are both secure and scalable.
The request method, such as GET, POST, PUT, or DELETE, determines how the client interacts with the API, and it is crucial to select the correct method for authentication processes like OAuth and JWT.
A POST request is commonly used to submit data to an API, such as sending authentication credentials to obtain an access token in OAuth workflows. The request body in a POST request is essential for transmitting data, such as authentication credentials or user details, to the API for processing.
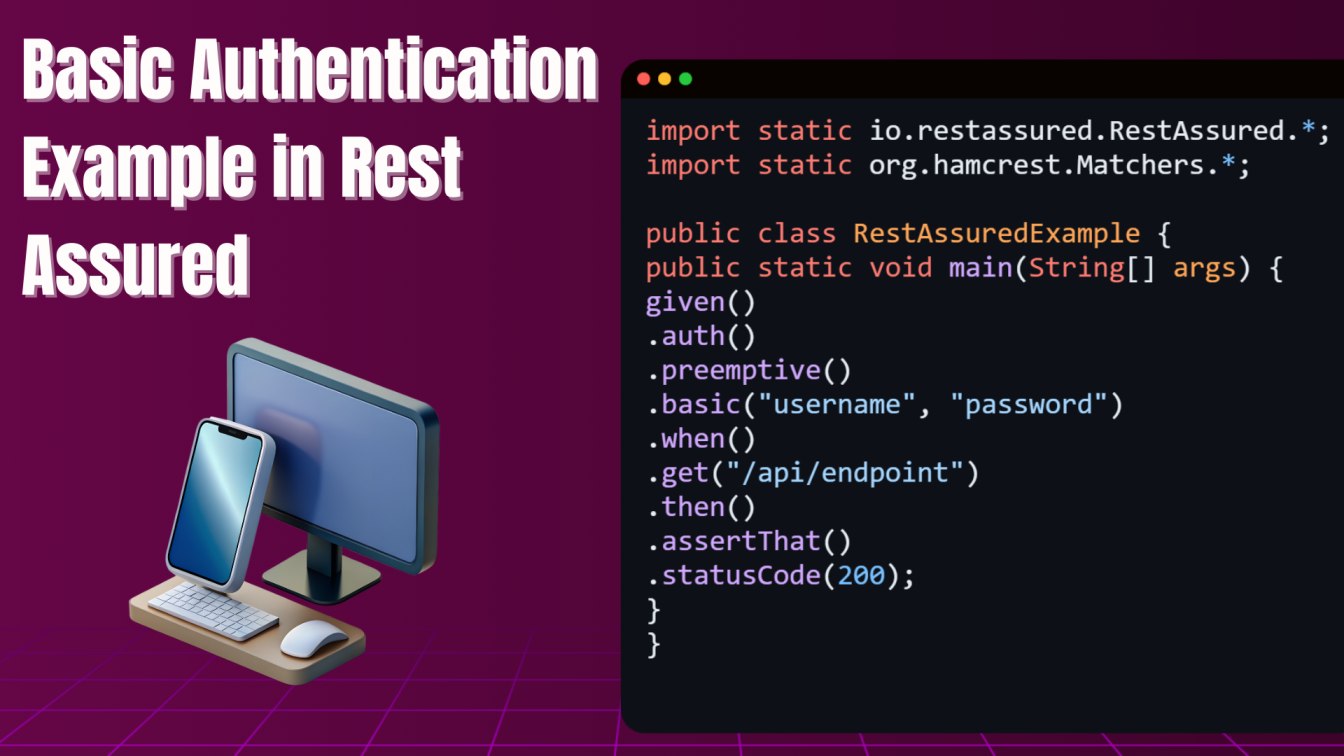
OAuth Authentication in Rest Assured
OAuth (Open Authorization) is widely used for secure token-based access. There are two main types: OAuth 1.0 and OAuth 2.0, with OAuth 2.0 being the most common in modern applications. In OAuth 1.0, the token secret is critical for signing requests, ensuring that only authorized clients can access the API, and Rest Assured simplifies the process of testing these secure interactions.
OAuth 1.0 in Rest Assured:
OAuth 1.0 requires the client to sign requests using a consumer key and secret.

OAuth 2.0 in Rest Assured:
OAuth 2.0 simplifies the process by using access tokens instead of signed requests.
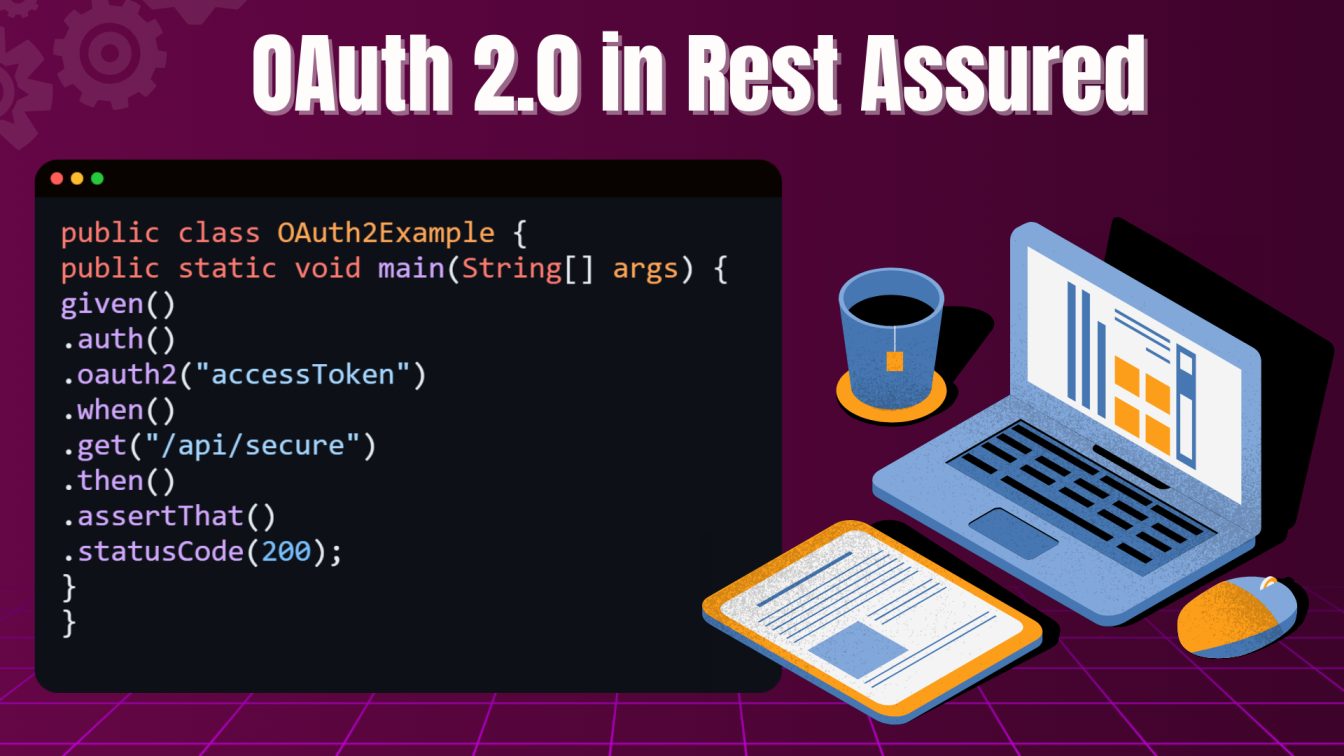
OAuth 2.0 is commonly used in applications such as Google and Facebook API integrations, where secure access to resources is needed.
JWT (JSON Web Tokens) Authentication
JWT (JSON Web Tokens) have become an increasingly popular method of securing APIs, especially for modern, stateless systems. Unlike OAuth, which typically requires interaction with an authorization server, JWT is self-contained, meaning that all the information needed to verify the user or client is encoded within the token itself. JWTs are ideal for Single Page Applications (SPAs) and mobile apps, which require minimal interaction with servers after authentication.
Rest Assured makes it simple to include JWTs in your automated API tests. Once a user authenticates, the server issues a JWT that can be used for future requests. This allows secure communication without requiring the user to send credentials repeatedly.
The JWT token is passed in the HTTP header, and using Rest Assured, you can automate the process of adding this token to your requests, ensuring that all endpoints that rely on JWT authentication are properly tested. Request parameters are essential for passing authentication credentials in API calls, such as access tokens and API keys, and Rest Assured offers a simple way to include them in your tests.
JWT also works well in automation testing tools and can easily integrate with other security mechanisms like OAuth, enabling hybrid authentication solutions for robust API security.
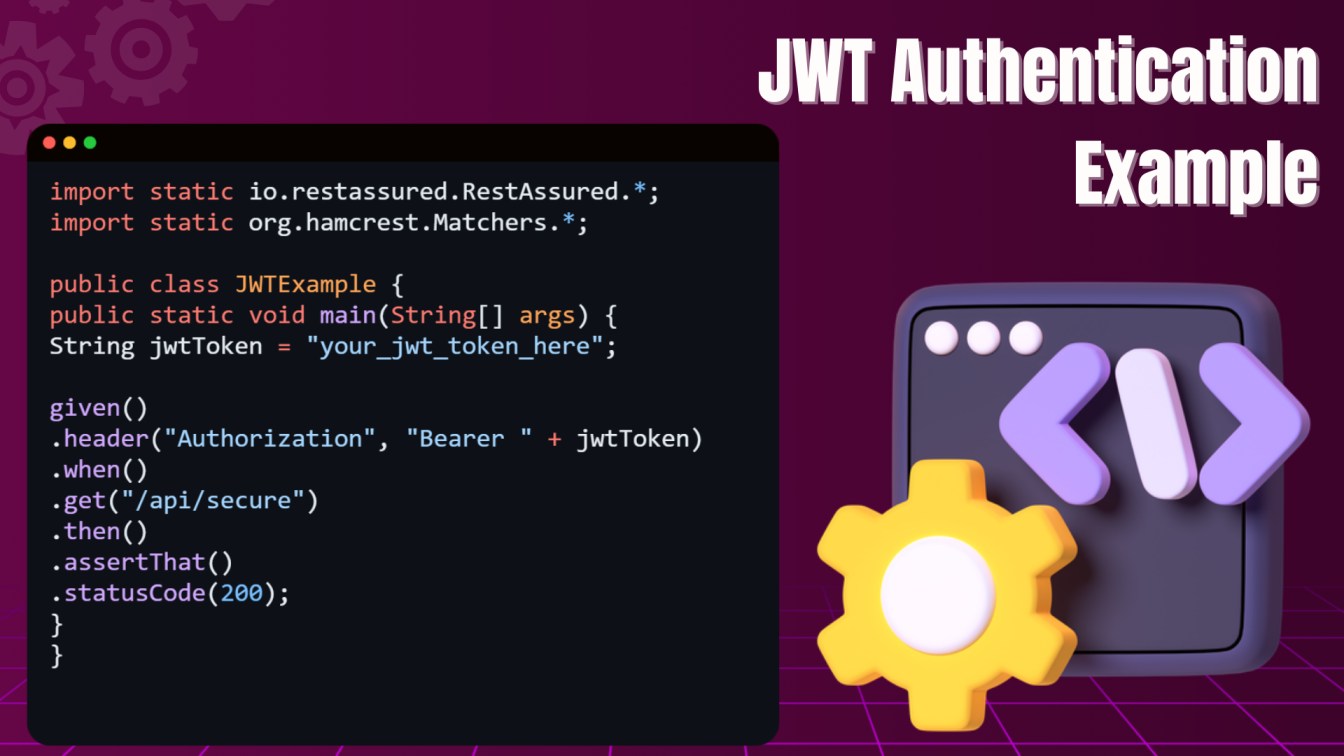
API Key Authentication
API Key authentication is one of the simplest, yet widely used methods to authenticate access to an API. Many public-facing APIs use API keys to grant access, making it an essential feature to include in your test automation suite. Unlike OAuth or JWT, API Key authentication does not involve complex token management or multiple layers of security. Instead, the client passes a unique API key as part of the request, which the server uses to validate access.
The response status code is crucial in determining the outcome of an API call, indicating whether authentication was successful or if there were issues such as unauthorized access. The content type in API requests and responses indicates the media type of the data being sent, which is essential for properly handling authentication credentials in formats like JSON or XML.
Rest Assured allows you to automate this process easily. By embedding API keys in the query parameters or headers, you can simulate multiple client requests and test how your API responds to valid and invalid keys. With automation solutions like Postman and Rest Assured, handling API Key authentication becomes straightforward, but it’s important to validate that the API handles incorrect, expired, or compromised keys gracefully. Creating a request in Postman is a user-friendly way to test APIs, allowing you to easily configure authentication methods and view responses in real-time.
Given the simplicity of this approach, API Key authentication is often used for internal APIs, making it crucial for automation tools for API testing to thoroughly validate how this method is integrated into your API’s security model.
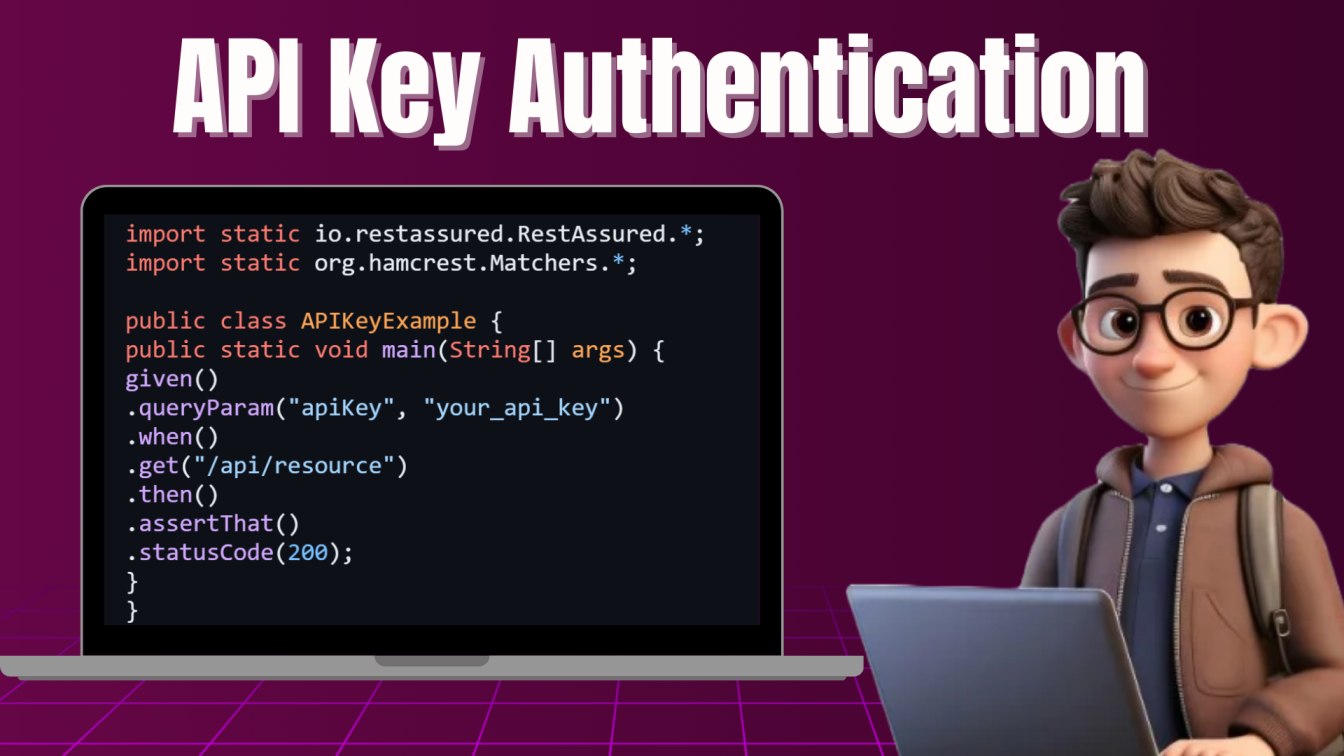
Bearer Token Authentication
Bearer tokens are commonly used in conjunction with OAuth 2.0 and have become a favored method for securing APIs in both enterprise and small-scale applications. A bearer token is essentially a cryptographic string that represents the user's session, allowing them to access various resources without re-entering their credentials. One of the advantages of bearer tokens is their flexibility, as they can be issued for various levels of access, making them highly customizable for different roles and permissions within a system.
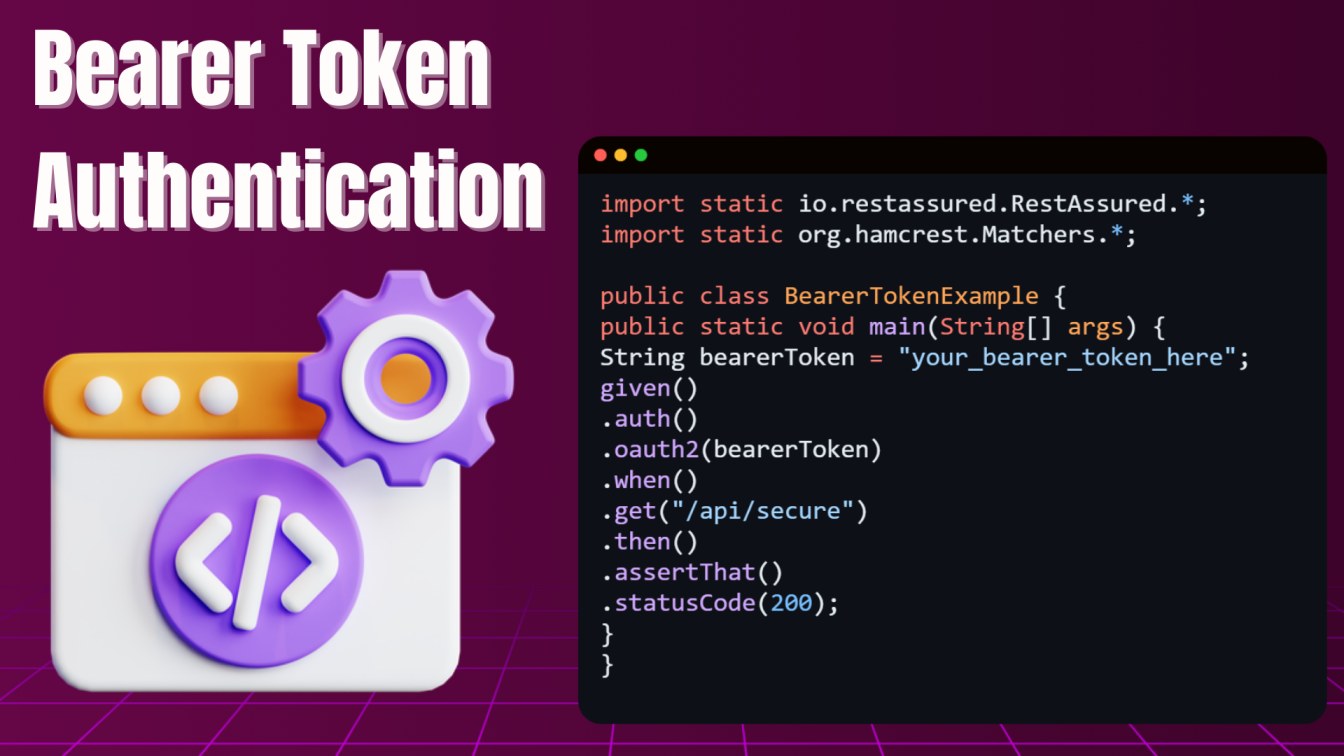
Rest Assured allows you to easily integrate bearer tokens into your automated API tests. By using the .auth().oauth2() method, you can pass the token in the Authorization header and simulate authenticated requests. This feature is essential in ensuring that your API properly verifies bearer tokens, handles expired tokens, and returns the correct status codes when token-based authentication fails. This capability is key in types of automation testing tools that need to validate real-world API security mechanisms, particularly for public-facing systems.

Handling Multi-factor Authentication (MFA)
Multi-factor authentication adds another layer of security by requiring a second form of verification beyond just a password or token. While handling MFA in automated testing can be challenging, Rest Assured allows for scripting both the initial login and the retrieval of the second factor.
You can use Rest Assured to simulate the first login, capture the OTP (One-Time Password), and then pass it for further authentication.
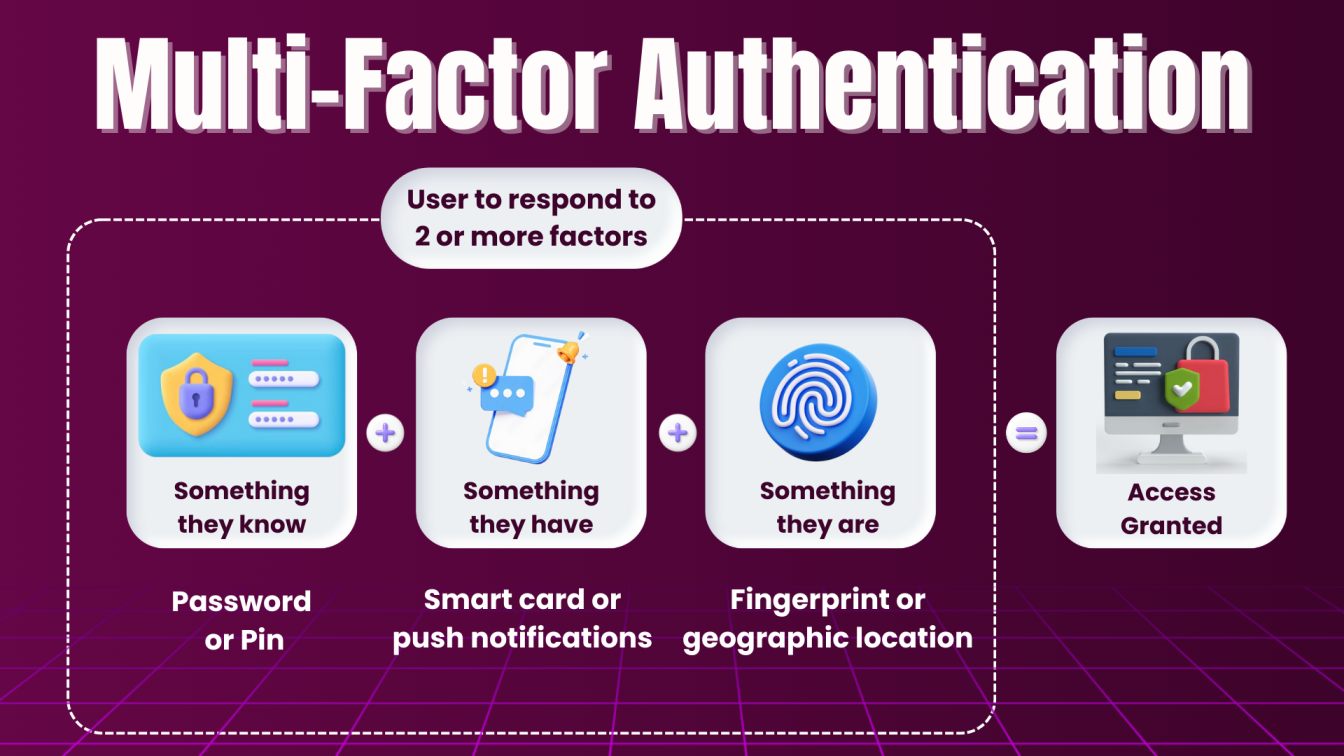
Refreshing Expired Tokens in Rest Assured
In token-based systems like OAuth and JWT, access tokens often have an expiration time. You may need to refresh tokens during your tests. Additional requests may be necessary to handle specific authentication flows, such as obtaining access tokens or refreshing expired tokens, which can be seamlessly managed using Rest Assured. The current token is essential for maintaining user sessions in API authentication, allowing clients to access protected resources without repeatedly providing credentials.
To refresh a token:
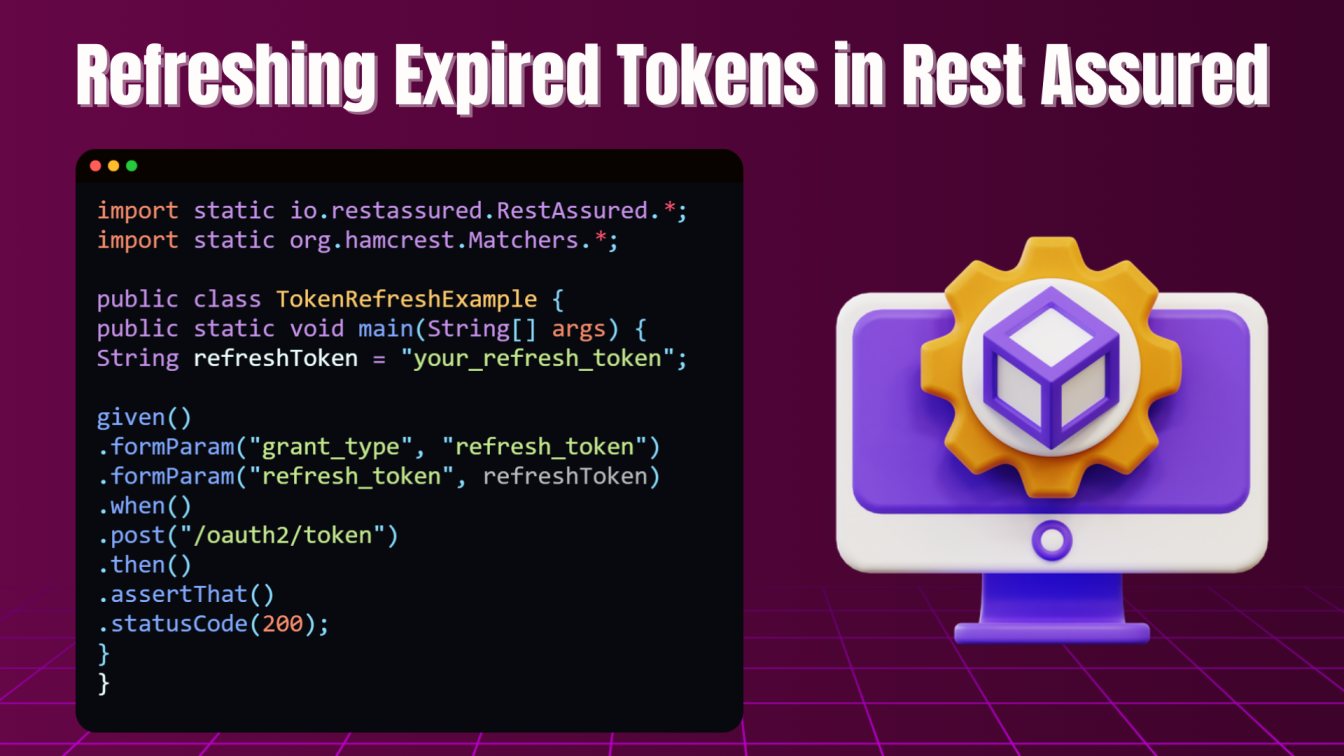
Refreshing tokens ensures that long-running automated tests do not fail due to token expiry.
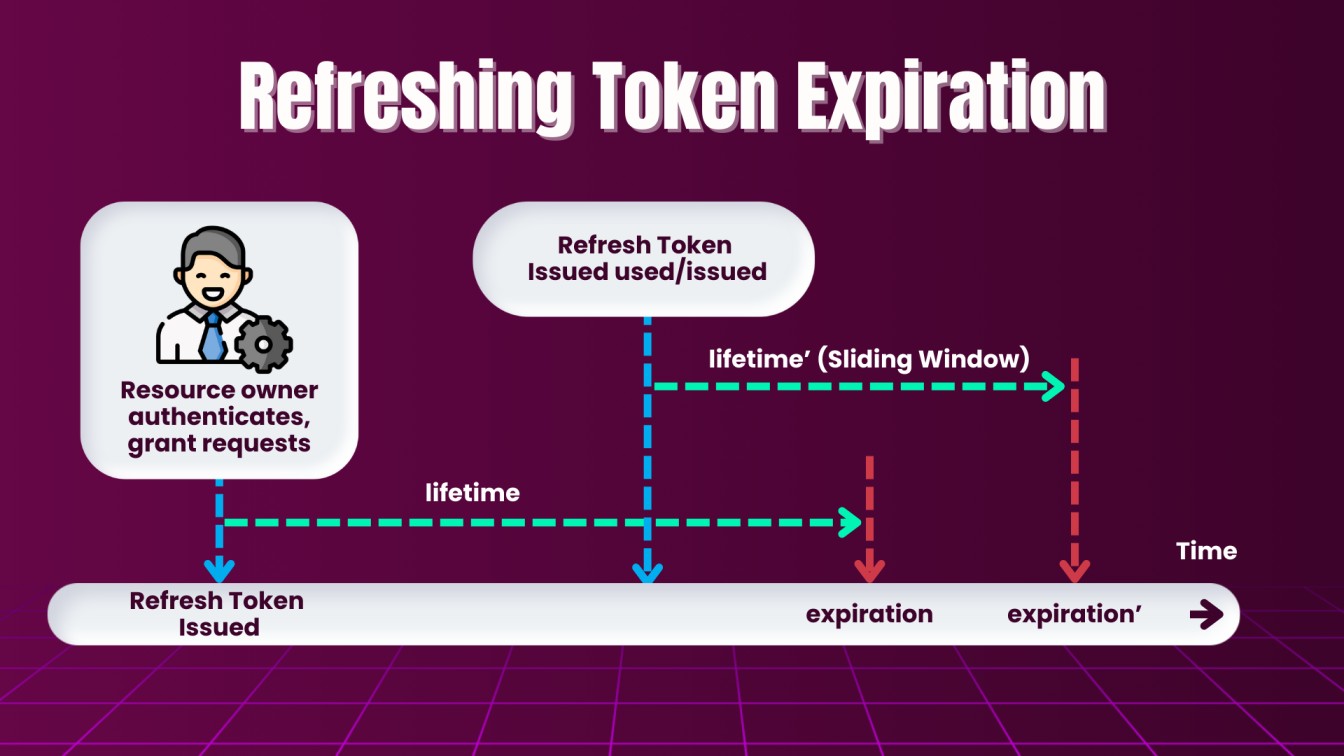
Testing Authorization Failures
When automating API testing with Rest Assured, it’s crucial to test scenarios where incorrect or missing credentials lead to authentication failures. This helps ensure your APIs handle unauthorized access appropriately.
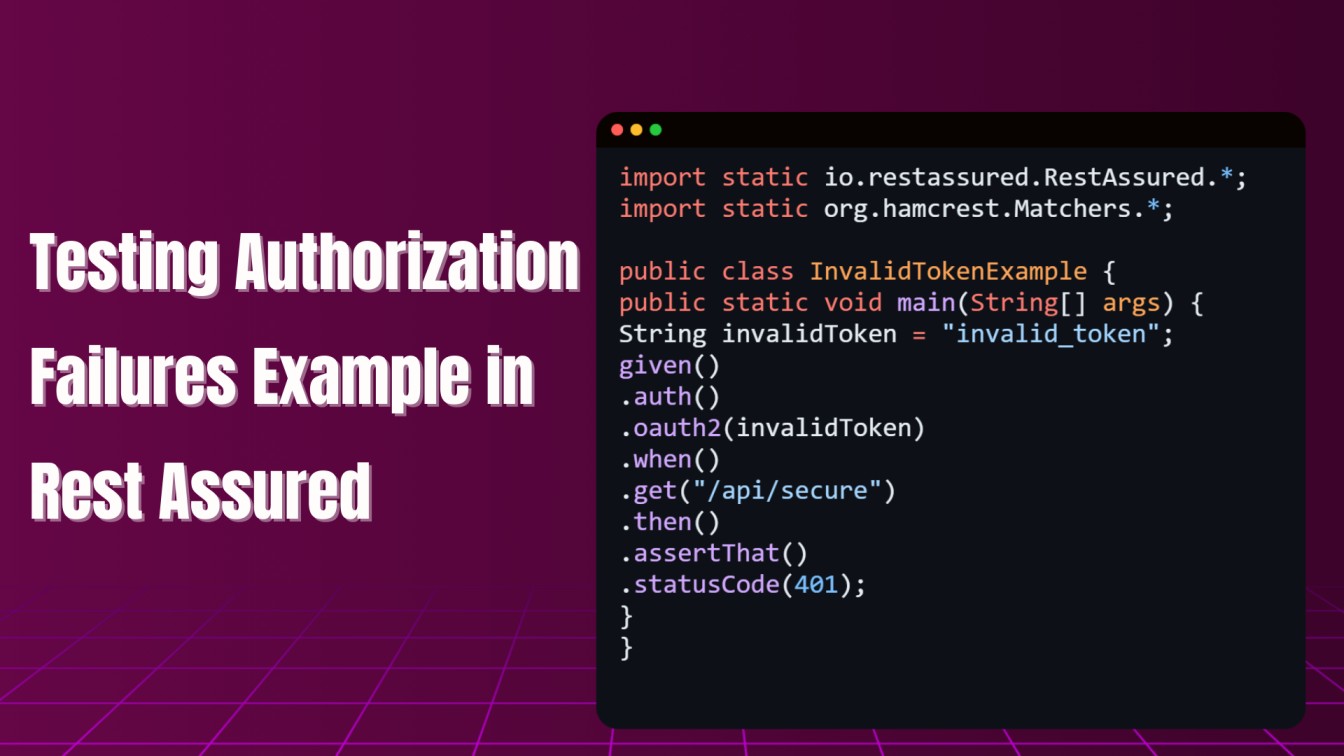
In addition to handling incorrect credentials, it’s equally important to understand the factors that can lead to authorization failures. This allows for proactive identification and testing of areas that may present security risks.
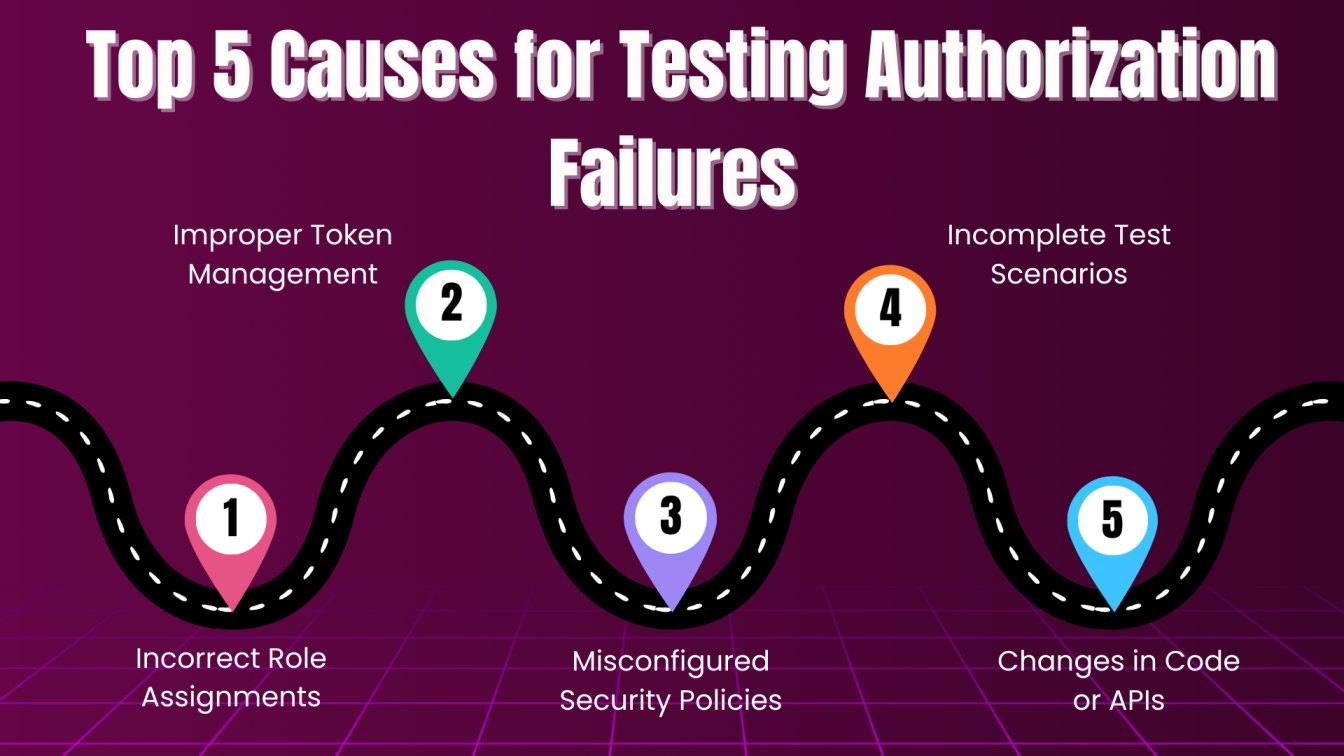
Debugging Authentication Issues in Rest Assured
Debugging authentication issues can be one of the more challenging aspects of API testing. Whether it's due to incorrect tokens, expired credentials, or misconfigured security settings, failing to authenticate properly can cause a range of issues in your test suite. When handling complex authentication mechanisms like OAuth or JWT, it’s essential to have a way to capture and analyze the authentication flow.

Once basic logging is enabled, you can move deeper into analyzing common causes of failed authentication. This includes double-checking your API documentation, endpoint URLs, request headers, and other vital components to ensure they align with the API’s expected authentication requirements.

Security Best Practices in API Automation
Software testing automation tools like Rest Assured play a critical role in ensuring APIs are securely authenticated through mechanisms such as OAuth and JWT, streamlining the testing process. When handling sensitive data such as API keys and tokens, follow security best practices like:
- Storing credentials securely using environment variables
- Rotating access tokens regularly
- Avoid hardcoding sensitive information in your automation testing tools

Integration with CI/CD Pipelines
One of the core benefits of using automation testing tools like Rest Assured is the ability to integrate your API tests into CI/CD pipelines. This ensures that every change in the codebase is tested against your API’s authentication mechanisms, reducing the risk of deploying insecure or broken APIs to production. By automating authentication tests in your pipeline, you guarantee that security is always a top priority, with tests running continuously and automatically.
With tools like Jenkins, Travis CI, or GitHub Actions, you can trigger automated API tests whenever new code is committed. This integration allows for immediate feedback on whether your authentication flow is working as expected. Furthermore, when combined with robotic process automation solutions, these tests ensure that authentication across different microservices is thoroughly vetted. This helps organizations detect issues early and avoid the costly repercussions of failed security implementations in production.
Role-Based Authentication in Testing
In many applications, different user roles (e.g., admin, user, guest) have varying levels of access to resources. Properly testing these role-based access controls (RBAC) is essential to ensuring that users only have access to the resources they are permitted to use. With Rest Assured, you can automate tests to validate these access controls by simulating different roles and verifying the API’s response.
For instance, you can automate tests for scenarios where an admin can access protected resources, while a regular user receives a “403 Forbidden” error when attempting the same action. This type of testing ensures that RBAC is implemented correctly and that sensitive data is protected from unauthorized access. By leveraging API automation testing tools, you can scale these tests to cover all role-based scenarios, ensuring that every user type is properly validated across all API endpoints.
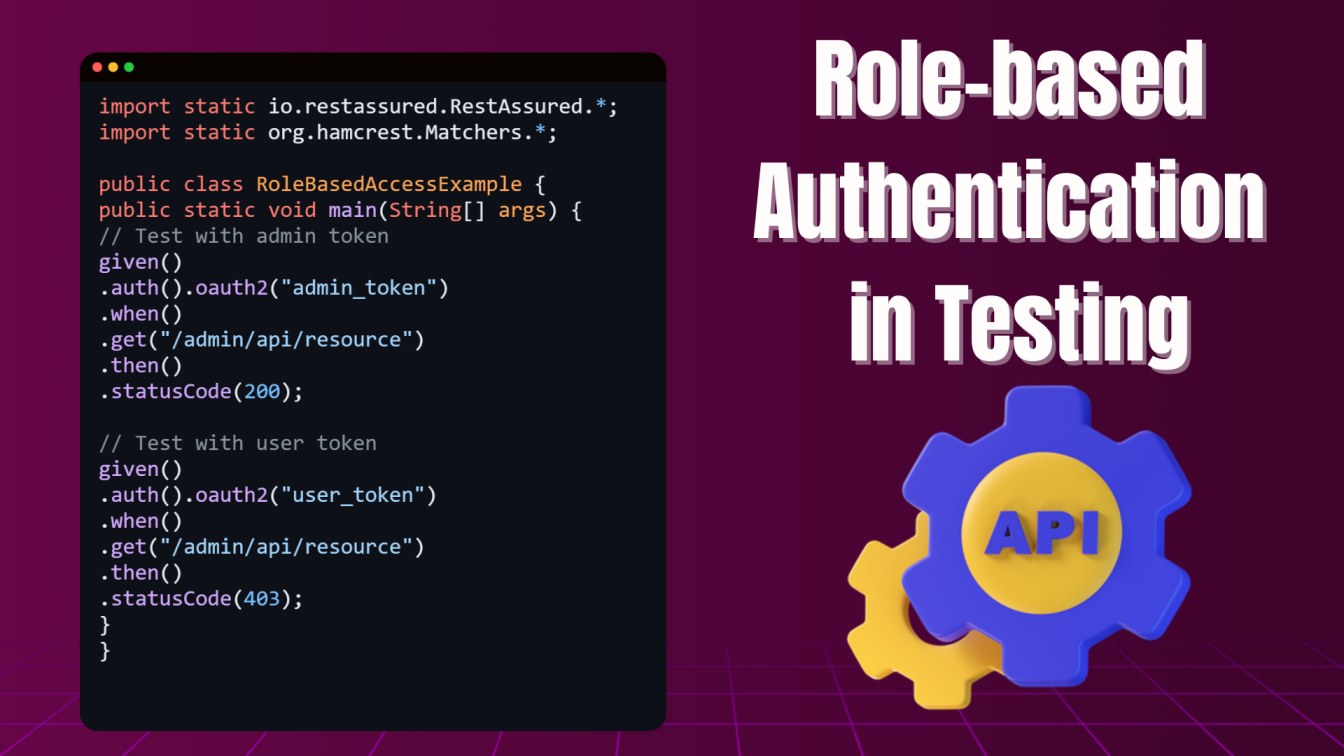
Conclusion
As API security becomes more important, properly handling authentication using automation software testing tools like Rest Assured is critical. For dealing with OAuth, JWT, or basic authentication, incorporating these mechanisms into your automated tests ensures comprehensive coverage.
Including proper authentication management in your API test suite unlocks the full potential of automation testing tools and helps deliver secure, reliable software. Whether you're using the best automation tools for API security or testing multiple authentication types, proper handling of authentication is key to a successful API automation strategy.
People also asked
👉 How to handle OAuth in Rest Assured?
You can handle OAuth in Rest Assured using .auth().oauth2("accessToken") for OAuth 2.0 or .auth().oauth() for OAuth 1.0.
👉 Can OAuth and JWT be used together?
Yes, OAuth can issue JWT tokens, and they are often used together for secure API authentication.
👉 Is JWT applicable for both authentication and authorization purposes?
Yes, JWT can be used for both authentication (verifying identity) and authorization (granting permissions).
👉 What is the difference between JWT authentication and basic authentication?
JWT uses tokens for stateless authentication, while basic authentication transmits a username and password with each request.
👉 What is the difference between JWT and authentication bearer?
A JWT is a type of token, while a bearer token is a format in which JWT or other tokens are passed in the Authorization header.