An essential component of exception handling in Selenium scripts is managing unforeseen issues to ensure smooth test execution. Timeout exceptions, missing elements, and script failures are common challenges that can disrupt the automation process. By implementing an effective exception-handling mechanism, testers can control these failures, allowing tests to either continue running or fail gracefully with meaningful error messages.
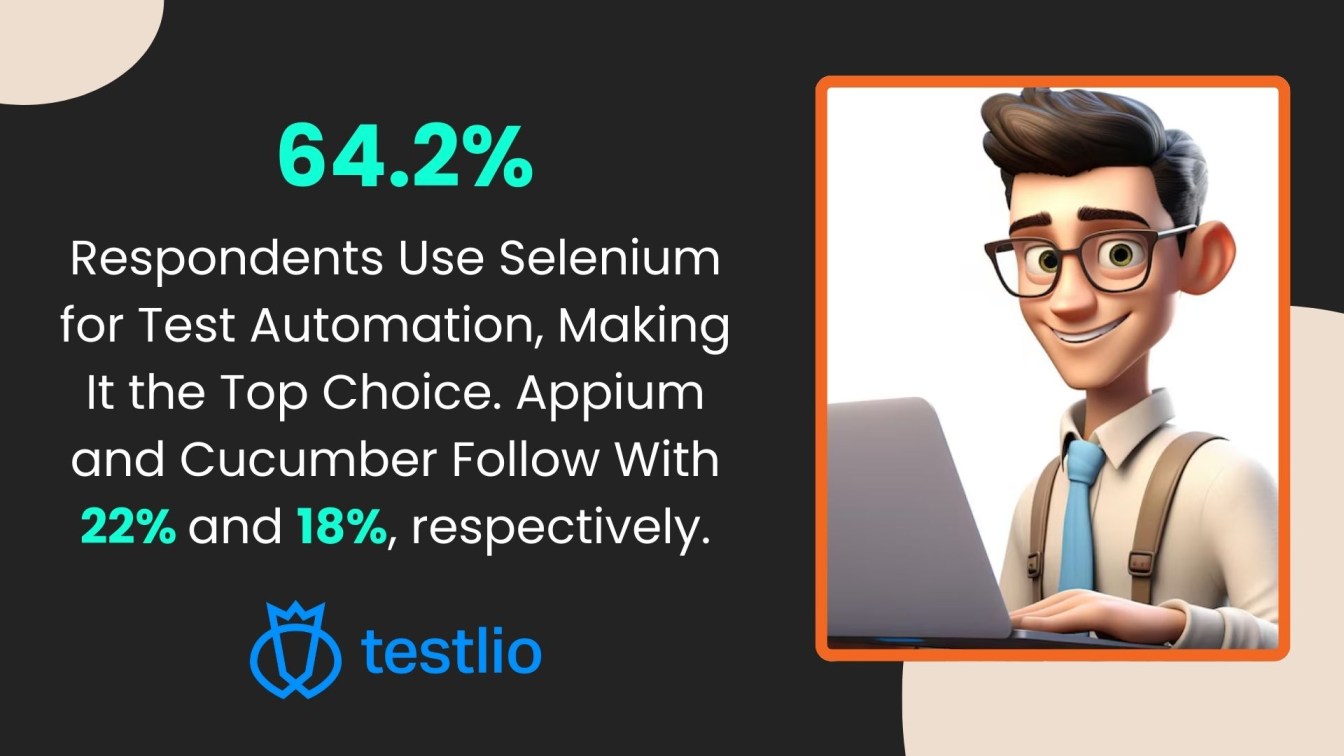
Selenium encounters various kinds of exceptions, including NoSuchElementException, TimeoutException, and StaleElementReferenceException. Understanding the hierarchy of exceptions and using proper handling techniques like try-catch blocks helps in identifying, logging exceptions, and managing errors efficiently. Creating structured exception messages and utilizing an exception object ensures clarity in debugging.
By integrating robust error-handling practices and leveraging the right method signatures, testers can enhance the stability of automation scripts, minimizing disruptions and improving overall test reliability.
What's next? Keep scrolling to find out:
🚀 Importance of exception handling in Selenium for test stability.
🚀 How to manage common exceptions like NoSuchElementException and TimeoutException.
🚀 Using explicit waits to prevent errors and improve automation flow.
🚀 Best practices for handling unexpected alerts and element interaction issues.
🚀 Advanced techniques for efficient exception handling in Selenium.
What is Exception Handling in Selenium?
Introduction to Selenium Exceptions is crucial for managing runtime errors and programming errors that occur during test execution, preventing unnecessary disruptions. Exceptions in Selenium scripts can arise due to missing elements, timeouts, or issues with the target element, leading to test failures. Using structured exception handler techniques ensures smooth execution and minimizes Assertion errors.

Different programming languages in Selenium, such as Java exceptional handling, C# exceptional handling, Python exceptional handling, and C++ exceptional handling, provide mechanisms to handle typical exceptions. Java uses try-catch blocks, while Python utilizes try-except, both allowing detailed exception details and meaningful error messages. Handling exceptions effectively helps manage issues related to target windows, ensuring tests interact only with active windows and the correct window. Common exception in Selenium includes no such element and element not interactable errors. A well-implemented hierarchy of exceptions enhances the debugging of issues and keeps the Selenium framework stable, even when exceptions in Selenium occur.
Why is Exception Handling Important in Selenium Automation?
Exception handling is a vital part of Selenium automation as it ensures your tests run smoothly, even when unexpected issues occur. Different exceptions in Selenium cover a wide range of error types. By effectively managing errors, you can make your test scripts more reliable and easier to maintain.
- Exception handling is a powerful part of Selenium automation, ensuring that tests run smoothly despite unexpected issues.
- It improves test stability by preventing abrupt failures, allowing tests to either continue or fail gracefully with clear messages.
- Enhances debugging by providing informative error messages and logs, helping identify issues like incorrect selectors or common Selenium exceptions quickly.
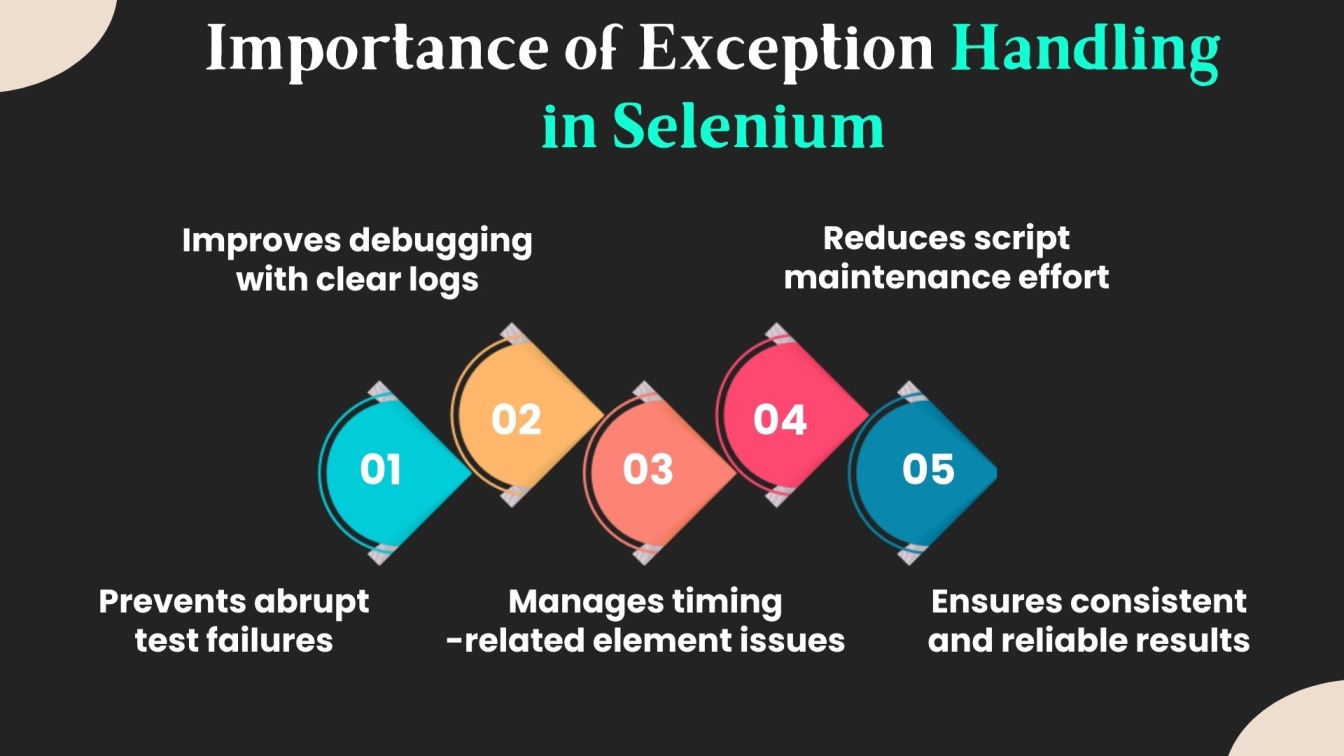
- Supports dynamic element management, allowing scripts to wait or retry actions for elements that aren’t ready or interactable, handling timing-related exceptions effectively.
- Reduces maintenance effort, as well-implemented exception handling makes scripts less prone to breaking from minor changes in the application or browser session.
- Ensures consistent test results by managing unexpected events and reducing false positives and false negatives.
- It allows for custom exception implementations, making scripts more flexible and tailored to specific test needs.
Common Types of Exceptions in Selenium
Selenium automation encounters a range of exception types that can disrupt test execution. A strong understanding of exception handling helps in writing stable scripts while improving code readability and reliability. Below are some frequently occurring exceptions in Selenium scripts:
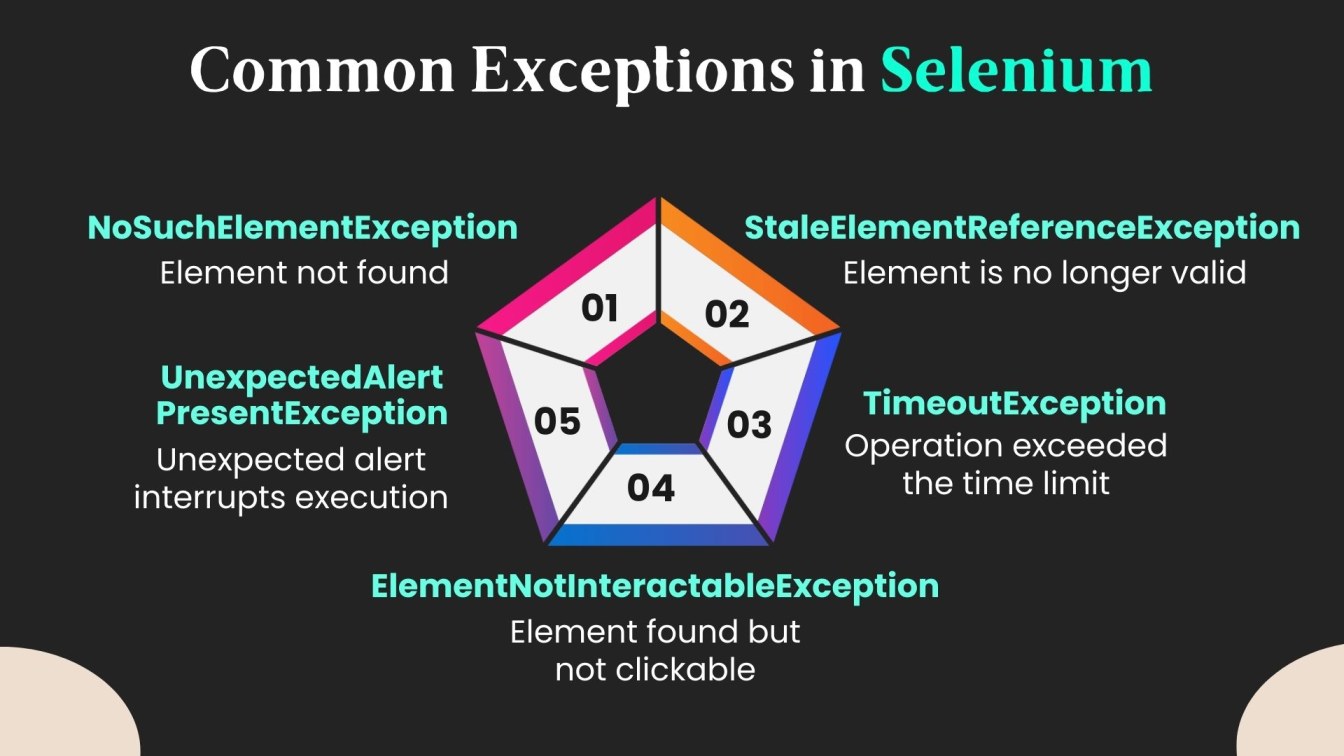
- NoSuchElementException: Raised when Selenium fails to locate the requested element, often due to incorrect locators or dynamic XPath issues.
- StaleElementReferenceException: This occurs when an element becomes invalid after a DOM modification, making it unreferencable in the script.
- TimeoutException: Triggered when an operation, such as waiting for an element, exceeds the specified time, affecting execution flow.
- ElementNotInteractableException: Thrown when an element is found but cannot be interacted with, often due to incorrect state or visibility issues.
- UnexpectedAlertPresentException – Raised when an unexpected alert appears, stopping test execution and requiring explicit handling within the block of code.
Recognizing these standard exceptions, along with their hierarchy of exceptions, ensures efficient debugging. Understanding the object of the exception class allows better exception handling, making automation scripts more resilient.
How to Handle NoSuchElementException in Selenium
To make sure that your test scripts can handle situations when an element cannot be found on the page gracefully, you must handle the NoSuchElementException in Selenium. Usually, this exception happens when the element you are attempting to interact with is invisible or nonexistent.

To avoid this, you can first ensure the element is present by using explicit waits. For example, use WebDriverWait to wait for the element to become visible or clickable:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class SeleniumWaitExample {
public static void main(String[] args) {
WebDriver driver = // Initialize WebDriver instance
try {
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("submit_button")));
element.click();
} catch (Exception e) {
System.out.println("Element not found or not visible: " + e.getMessage());
} finally {
driver.quit();
}
}
}
You can also catch and handle this error gracefully by enclosing your operations in a try-except block, which keeps your script from crashing.
How to Handle ElementNotInteractableException in Selenium
In Selenium automation testing, encountering the ElementNotInteractableException indicates that an element is present in the DOM but cannot be interacted with, often due to it being hidden, disabled, or off-screen.
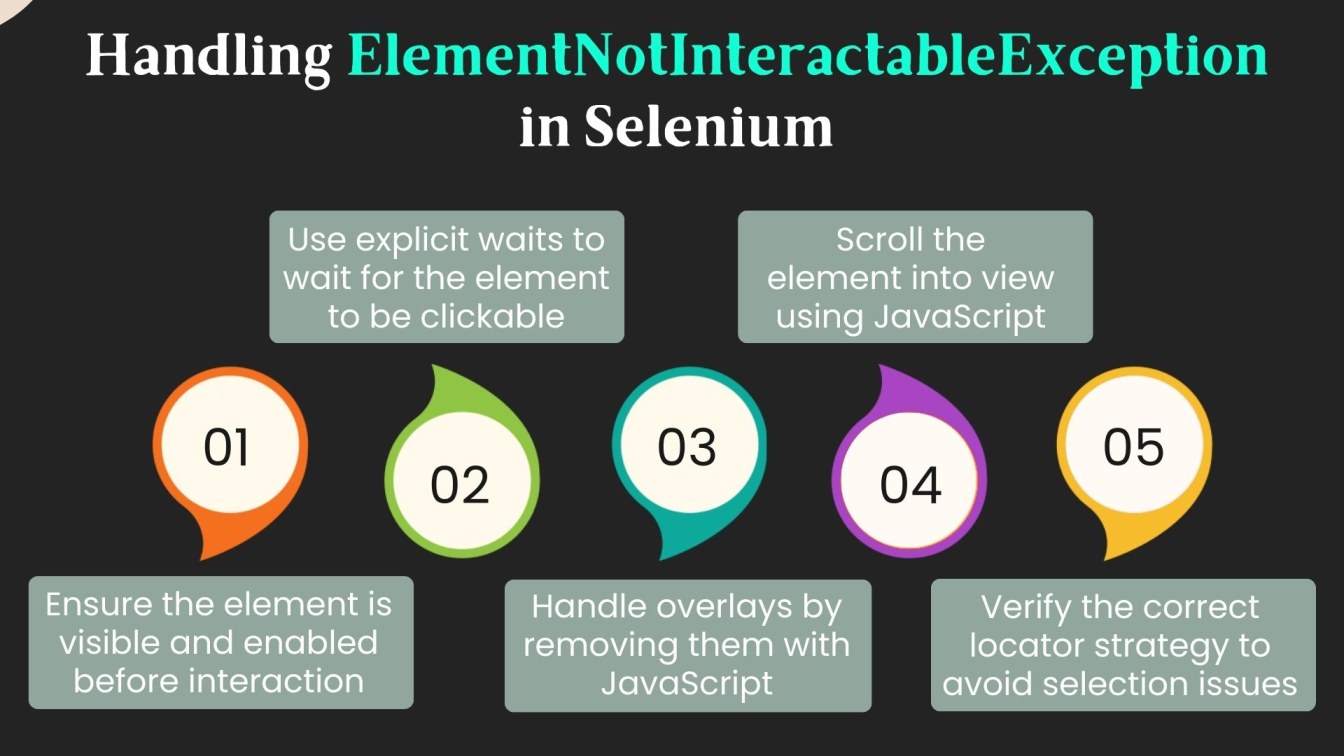
To address this issue, consider the following approaches:
- Ensure Element Visibility and Enablement: Before interacting with an element, confirm that it is both visible and enabled. You can use explicit waits to wait for the element to become clickable:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class SeleniumWaitExample {
public static void main(String[] args) {
WebDriver driver = // Initialize WebDriver instance
try {
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id("submit_button")));
element.click();
} catch (Exception e) {
System.out.println("Element not clickable: " + e.getMessage());
} finally {
driver.quit();
}
}
}
2. Handle Overlays:
If an overlay is covering the element, you can use Java to remove it:
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
public class JavaScriptExecutorExample {
public static void main(String[] args) {
WebDriver driver = // Initialize WebDriver instance
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("document.getElementById('overlay').style.display='none';");
}
}
Scroll Element into View:
If the element is off-screen, scroll it into view using Java:
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
public class ScrollIntoViewExample {
public static void main(String[] args) {
WebDriver driver = // Initialize WebDriver instance
WebElement element = driver.findElement(By.id("submit_button"));
JavascriptExecutor js = (JavascriptExecutor) driver;
js.executeScript("arguments[0].scrollIntoView(true);", element);
}
}
Dealing with StaleElementReferenceException in Selenium
When Selenium encounters a StaleElementReferenceException, it means that the script is trying to work with an element that has been modified in the DOM or is no longer there. This frequently occurs after a page refresh or when the content of the website changes dynamically.

Methods for Dealing with the StaleElementReferenceException:
- Re-find the Element: Before engaging with a WebElement, locate it right away rather than saving a reference to it. This guarantees that the element you're dealing with is the most recent version.
- Install Explicit Waits: Use WebDriver's explicit waits to pause action until certain circumstances are met, like the element becoming clickable or visible. This explains the loading of dynamic content.
- Employ Try-Catch Blocks with Retry Logic: Put interactions inside a try-catch block that retries the operation after moving the element after catching the StaleElementReferenceException.
- Avoid Superfluous DOM Interactions: Reduce the amount of time spent interacting with items that are likely to get stale, particularly in applications that contain dynamic content.
- Make Sure the Page Loads Completely: To avoid premature interactions, make sure the page or certain areas have loaded completely before interacting with items.
Handling TimeoutException in Selenium WebDriver
When a command in Selenium WebDriver takes longer than expected to execute, such as locating an element or loading a page, a TimeoutException occurs. Selenium webdriver exception helps debug and fix automation script errors. This is common in dynamic web applications where elements load asynchronously, requiring an effective exception-handling mechanism.
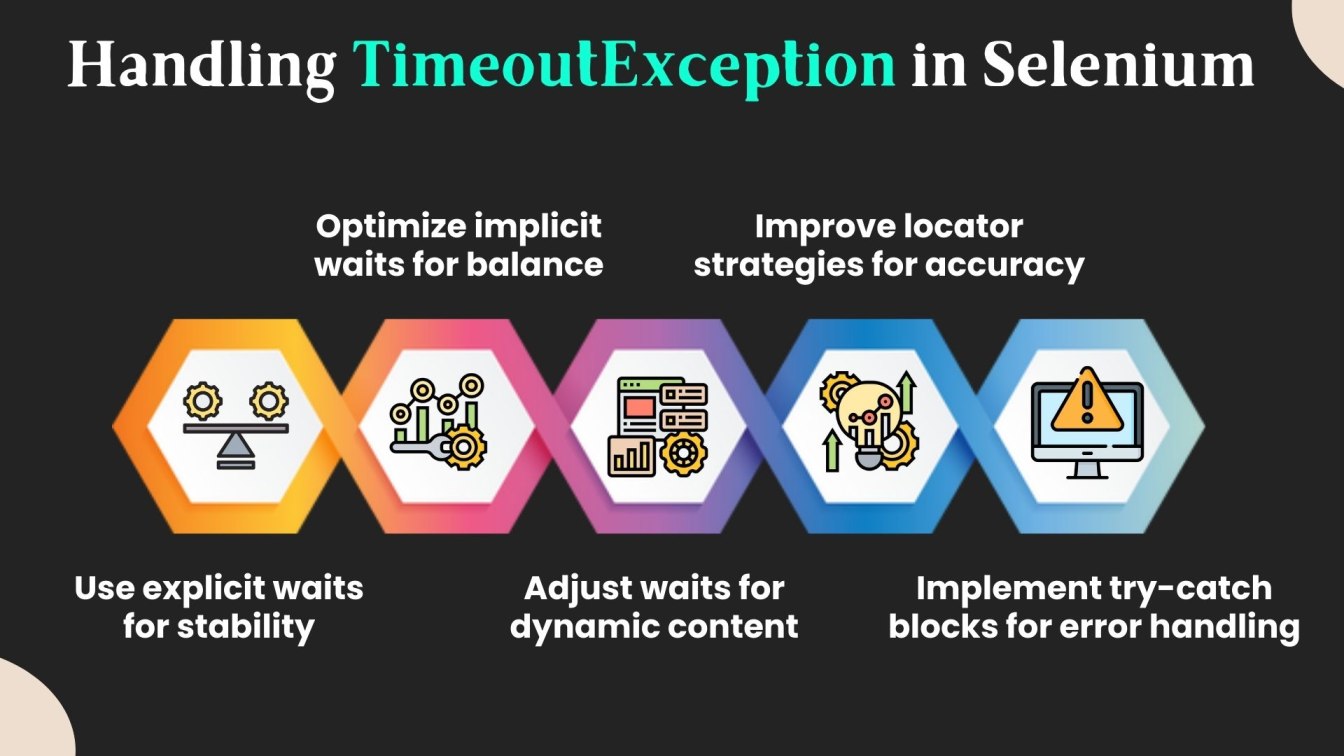
Methods for Handling TimeoutException
- Use Explicit Waits: Implement WebDriverWait with ExpectedConditions to pause execution until elements are available, reducing exception occurrence in Selenium scripts.
- Optimize Implicit Waits: Set implicitlyWait() to manage wait times for elements, balancing script efficiency and automation code execution.
- Handle Dynamic Content: Adjust waits for AJAX-based elements to avoid frequent exceptions, ensuring robust script execution.
- Enhance Locator Strategies: Use precise locators to prevent unnecessary delays and improve script reliability, minimizing uncaught exceptions.
- Try-Catch Blocks: Wrap Selenium commands in try-catch blocks to handle exceptions, log errors, and maintain smooth script execution.
Understanding exception hierarchy, using the throws keyword, and following best practices in exception handling can significantly enhance Selenium automation.
Best Practices for Exception Handling in Selenium
Selenium's efficient exception handling reduces unexpected errors and guarantees seamless test execution. Writing reliable automation scripts means foreseeing possible problems and resolving them politely.

- Use Explicit Waits - To avoid race situations, wait for elements to load using explicit waits rather than predefined sleep intervals.
- Install Try-Catch Blocks - To handle exceptions gracefully, wrap important Selenium commands in try-catch blocks.
- Handle Specific Exceptions: Identify and handle common exceptions such as TimeoutException and NoSuchElementException separately.
- Use Logging and Screenshots: To make debugging easier, record error details in logs and screenshots.
- Retry Failed Steps: To handle dynamic web content, retry unsuccessful tasks by relocating elements or utilizing loops.
Using Try-Catch Blocks for Exception Handling in Selenium
Selenium, effective exception handling is crucial for building robust and reliable test scripts. Utilizing try-catch blocks allows you to manage errors gracefully, ensuring that your tests can handle unexpected situations without abrupt failures.
Python Exception Handling: In Python try-except block is used to catch and handle exceptions. For example:
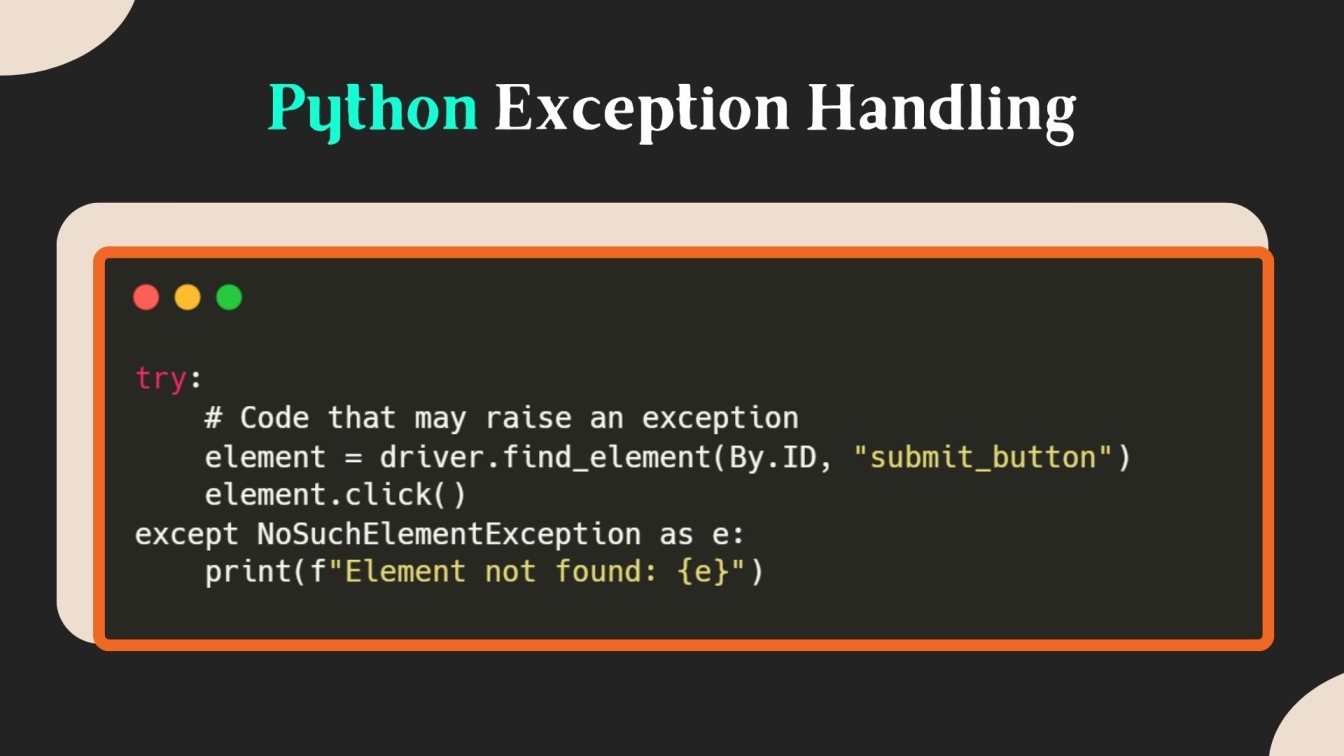
This app ensures that if the element is not found, the script continues without crashing.
Java Exception Handling: In Java, exception handling is achieved using try-catch blocks. For instance:
try {
// Code that may throw an exception
WebElement element = driver.findElement(By.id("submit_button"));
element.click();
} catch (NoSuchElementException e) {
System.out.println("Element not found: " + e.getMessage());
}
This ensures that if the element is not found, the exception is caught, and the script can handle it appropriately.
Using try-finally:
The finally block is used to execute a piece of code that must run regardless of whether an exception occurred or not. For example:
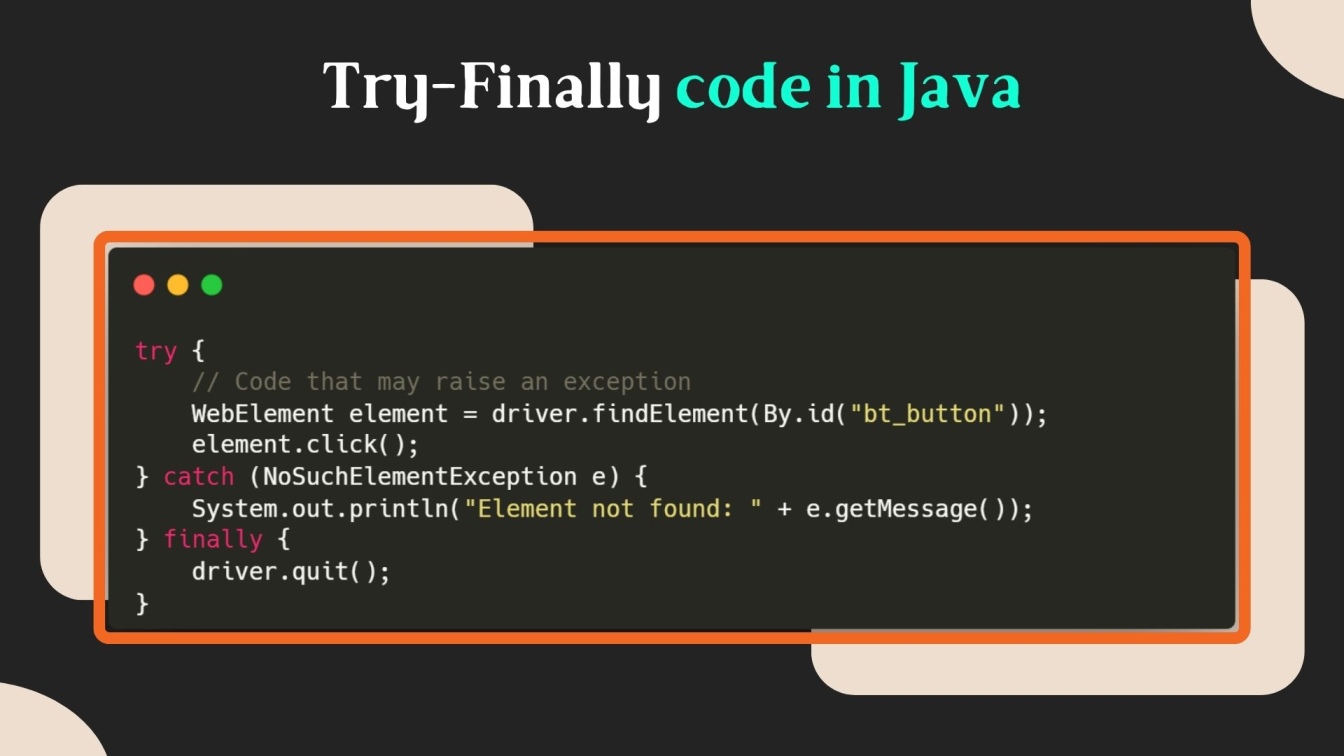
In this example.quit() will execute whether or not an exception was raised, ensuring that the browser closes properly.
By implementing these exception-handling techniques, you can create Selenium test scripts that are more resilient and capable of handling unexpected scenarios gracefully.
Understanding and Handling WebDriverException in Selenium
When the WebDriver cannot carry out a specified action, a general exception known as the WebDriverException is raised in Selenium WebDriver. This can occur for several reasons, including trying to use a browser after the WebDriver session has ended.
Common Causes:
- Session Closure: Attempting to perform actions after calling driver.quit() or driver.close().
- Browser Crashes: Unexpected browser crashes can lead to this exception.

Handling Strategies:
- Proper Session Management: Ensure that all interactions are completed before closing the WebDriver session.
- Exception Handling: Use try-catch blocks to gracefully handle exceptions and maintain test stability.
- Explicit Waits: Implement explicit waits to ensure elements are ready for interaction, reducing the likelihood of exceptions.
Using Explicit Waits to Avoid Exceptions in Selenium
Managing dynamic web elements is essential in Selenium automated testing to avoid problems such as ElementNotInteractableException. By using explicit waits, you can make sure that items are prepared for interaction by having your script halt until a particular condition is satisfied.

How to Handle UnexpectedAlertPresentException in Selenium
The UnexpectedAlertPresentException in Selenium happens when an unexpected alert interrupts the script's flow while the test is running. A try-catch block can be used to handle this exception and gracefully handle the alert.

This method guarantees that the script will transition to the unexpected alert, ignore it, and carry on without crashing. Managing these issues guarantees more dependable scripts and more seamless automated testing.
Advanced Techniques for Exception Handling in Selenium
Building robust and dependable Selenium code execution requires sophisticated exception handling in Selenium scripts. One effective approach is using explicit waits, which allow waiting for specific conditions (such as an element becoming clickable) before interacting with it. This reduces the likelihood of exceptions like ElementNotInteractableException and improves script reliability.
Handling multiple types of exceptions in a single try-catch block is another crucial strategy. Catching specific exceptions in Selenium scripts, such as NoSuchElementException and TimeoutException, independently provides greater control over how each is managed, ensuring smooth test execution.
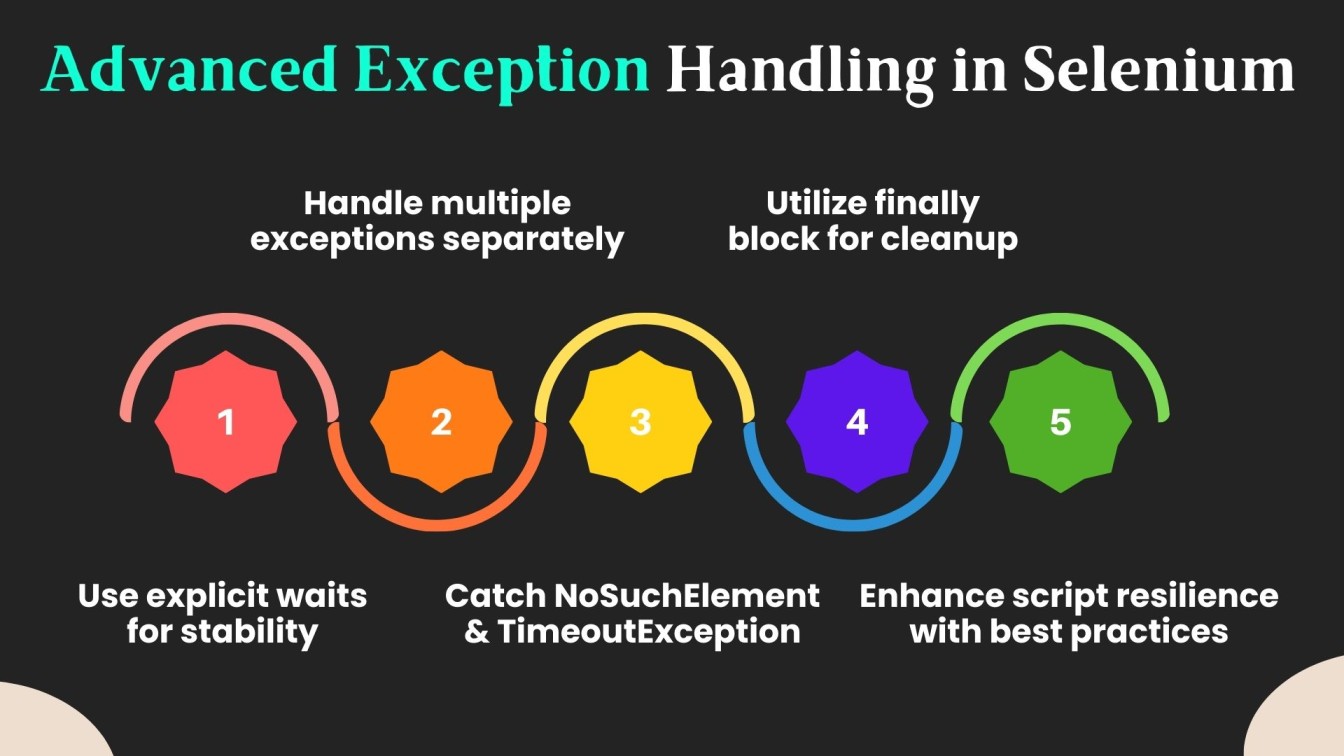
Additionally, leveraging the finally block ensures that essential operations, such as browser shutdown, are always executed regardless of whether an exception occurs. Understanding the hierarchy of exceptions and implementing these best practices enhances script resilience, making automation more effective in handling examples of exceptions across diverse testing scenarios.
Wind Up!!
Exception handling in Selenium WebDriver is essential for creating stable and reliable automation scripts. There are various types of exceptions, including checked and unchecked exceptions. Unchecked exceptions, such as NoSuchElementException, occur during program execution and can disrupt your test if not handled properly. On the other hand, checked exceptions must be explicitly managed.
Common Selenium IDE issues, like incorrect CSS selectors, can cause exceptions. Using catch statements in try-catch blocks helps manage these errors and ensures smooth test execution. You can also create custom exceptions using the throw keyword for more flexibility in your automation code. Proper exception handling prevents compilation errors, ensuring that your scripts continue to run without interruptions. With these techniques, handling window handles and exceptions becomes more efficient, making your testing process more robust and reliable. Happy testing!
People Also Ask
👉What is the difference between throw and throws?
The throw keyword is used to manually trigger an error condition, while throws declare exceptions a method might raise, ensuring structured handling within the custom exception hierarchy.
👉What is a null pointer exception in Selenium?
A NullPointerException occurs when an object is accessed before being initialized, affecting Selenium code execution and requiring proper handling using try-catch keywords.
👉What are the different types of waits in Selenium?
Selenium provides implicit, explicit, and fluent waits, ensuring correct window identification and synchronization for stable test execution in an automation framework.
👉How to print exception in Selenium?
Exceptions in Selenium scripts can be printed in Java using predefined methods like printStackTrace() or getMessage() for efficient debugging of issues in test scripts.
👉What is POM in Selenium?
The Page Object Model (POM) is a design pattern in the Selenium framework that enhances maintainability by separating test logic from UI elements.