Are your testing efforts aligned with the growing demand for faster, smarter, and more efficient web application testing?
End-to-end (E2E) testing plays a crucial role in ensuring the functionality and user experience of web applications. By simulating real-world user interactions, E2E testing verifies that all components of an application, from the user interface to the backend, work seamlessly together.
Playwright, a free and open-source automation framework created by Microsoft, is quickly becoming a popular choice for automating tests in web applications.
In this guide, we will walk you through the essentials of end-to-end testing with Playwright, providing insights into its setup, key features, and best practices. Whether you're testing a simple web app or a complex multi-page application, Playwright simplifies the testing process, helping you achieve high-quality results quickly.
From handling browser contexts and popups to integrating with CI/CD pipelines, this guide will cover everything you need to know to leverage Playwright for efficient and effective web application testing.
What's next? Keep scrolling to find out:
🚀A detailed comparison of Playwright and Selenium, exploring which tool is better for web testing.
🚀Why Playwright stands out compared to other automation tools available in the market.
🚀How to set up Playwright for automated web application testing from scratch.
🚀Key techniques for handling popups, alerts, and managing browser contexts within Playwright tests.
🚀Best practices for optimizing Playwright test performance and integrating it into your CI/CD pipeline for continuous testing.
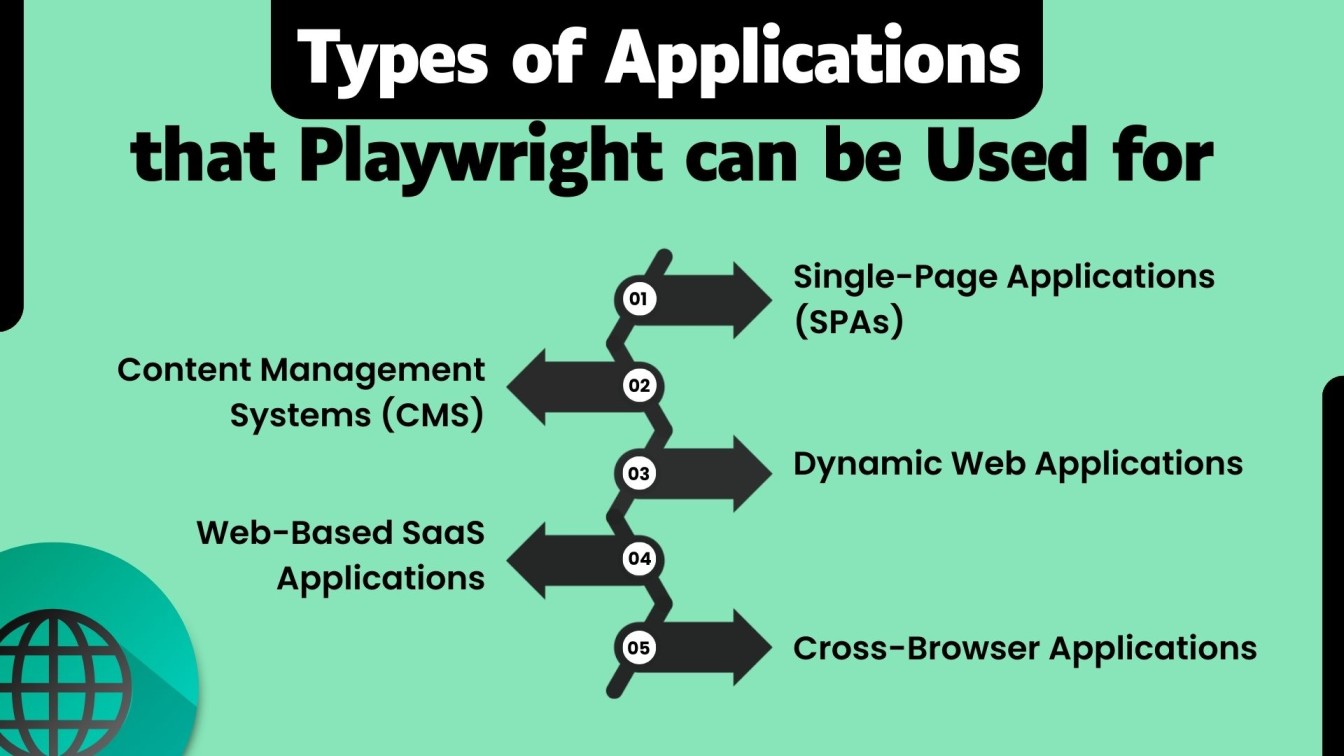
What is Automation Testing with Playwright?
Automation testing has become an integral part of modern software development, enabling teams to test applications more efficiently and consistently.
It provides a robust framework for automating tasks like interacting with web elements, testing across multiple browsers, and verifying functionality through simulated user interactions.
Playwright allows you to write reliable and fast test scripts that work with Chromium, Firefox, and WebKit, providing cross-browser testing in a single framework.
Playwright is a robust playwright automation tool that supports playwright Python, playwright Java, and playwright TypeScript for playwright end to end testing. With playwright locators, playwright selectors, and playwright test automation, it simplifies browser interactions. Playwright allows you to write reliable and fast test scripts that work with Chromium, Firefox, and WebKit, providing cross-browser testing in a single framework.
Unlike other testing automation tools, Playwright's built-in capabilities, like support for modern web apps and flexible API interactions, make it a popular choice for developers and testers alike.
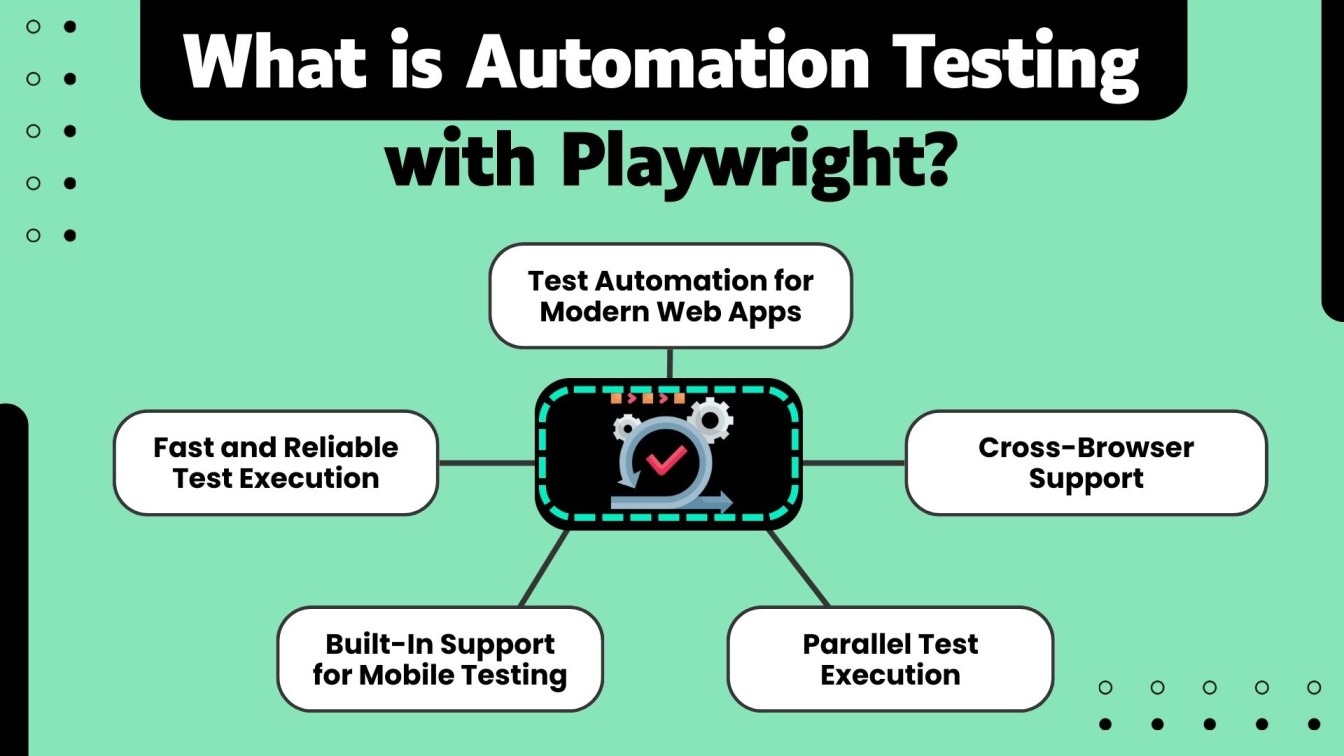
Playwright testing provides an advanced approach to test automation, offering rich features like automatic waits, advanced browser contexts, and parallel test execution.
It stands out when compared to other automation tools, such as Selenium, by offering a more consistent and feature-rich testing experience.
Playwright’s ability to handle scenarios like popups, authentication, and file downloads with ease makes it a go-to solution for web application testing.
With the Playwright GitHub repository and detailed Playwright documentation, getting started with Playwright is simple and accessible.
Playwright vs. Selenium: Which is Better for Web Testing?
When it comes to web automation testing, two tools that often come up are Playwright and Selenium. Both tools are widely used for automating web browsers, but they differ in key aspects such as performance, features, and ease of use.
Playwright, developed by Microsoft, has quickly gained popularity due to its modern approach and enhanced capabilities. In contrast, Selenium is one of the most widely recognized and long-established tools in the field of web automation.
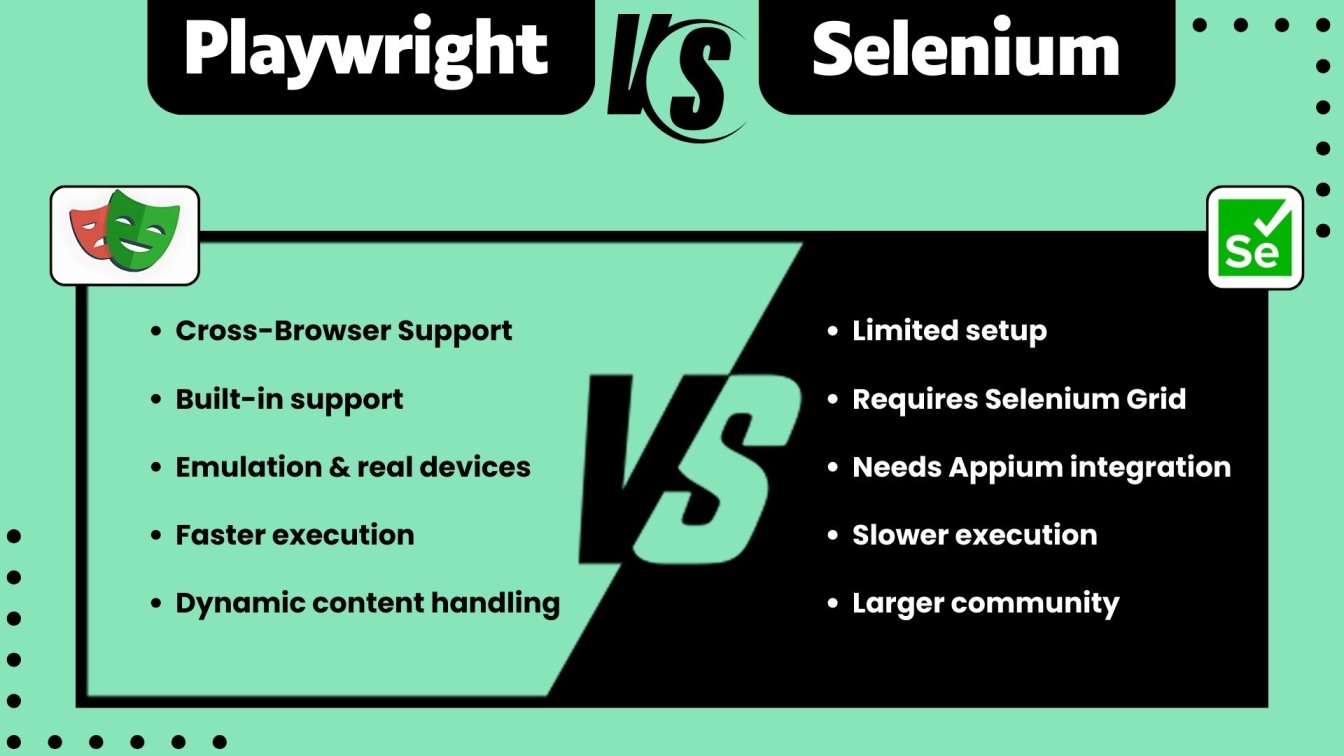
Playwright stands out for its speed, ease of use, and native support for modern web app features, making it an ideal choice for teams working on JavaScript-heavy applications or needing efficient parallel test execution. On the other hand, Selenium remains a strong option for teams working on legacy systems or projects that require broad compatibility with older tools and extensive community support. Ultimately, if you’re looking for a modern, fast, and robust testing solution, Playwright would likely be the better choice.

Playwright vs. Other Automation Testing Tools
Playwright stands out from other automation testing tools due to its robust support for cross-browser testing, faster execution, and flexibility in handling modern web applications.
Unlike Selenium, which requires additional setup for each browser and parallel test execution, Playwright simplifies the process with built-in support for Chromium, Firefox, and WebKit, and native parallel execution capabilities.
It also supports mobile device emulation and real-device testing, something that tools like Cypress and Puppeteer lack.
Playwright’s ability to test dynamic content, handle multi-tab testing, and simulate real-world user interactions makes it an ideal choice for complex web applications and modern workflows.
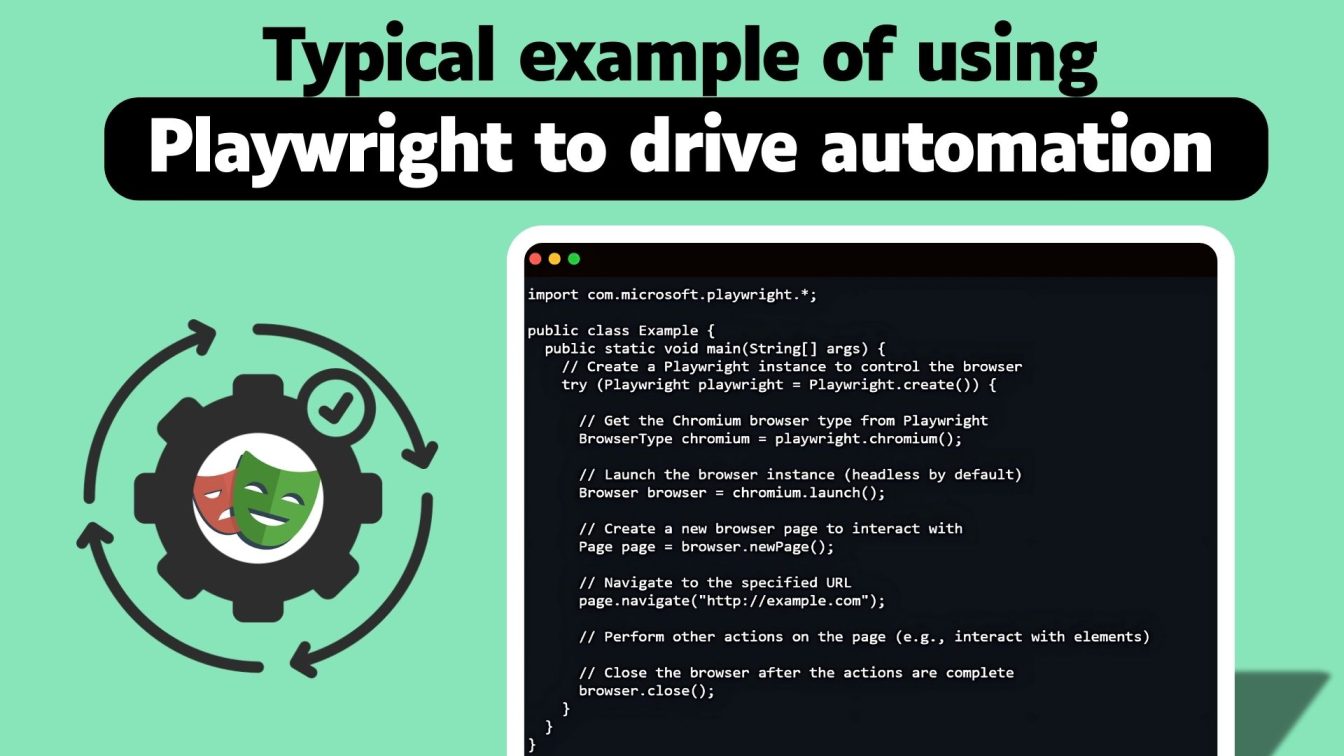
In comparison to other tools like TestCafe and Appium, Playwright offers a more streamlined setup and superior performance, especially when it comes to browser-based testing.
TestCafe, while great for simple tests, lacks the versatility and cross-browser testing capabilities of Playwright. Appium, although a powerful tool for mobile testing, is more complex to set up and is mainly focused on mobile applications.
Why Choose Playwright for Web Application Testing?
Playwright is gaining rapid popularity as a top choice for web application testing due to its robust feature set, speed, and flexibility in handling complex test scenarios. Unlike older tools such as Selenium, Playwright is designed to automate testing for modern web applications, including Single-Page Applications (SPAs), Progressive Web Apps (PWAs), and dynamic websites.
One of the key reasons to choose Playwright is its seamless cross-browser testing capabilities, allowing tests to be executed across Chromium, Firefox, and WebKit without needing multiple configurations or additional tools.

Playwright provides better test stability and execution speed compared to other testing frameworks. It eliminates common issues related to flakiness by automatically waiting for elements to load and ensuring tests are executed reliably.
Moreover, its advanced features, such as handling multiple browser contexts, real-time content updates, and mobile emulation, allow for comprehensive testing. Whether you are testing for performance, cross-browser compatibility, or user interactions, Playwright simplifies and accelerates the testing process, making it an excellent tool for developers and QA teams.
Setting Up Playwright for Automated Testing
Setting up Playwright for automated testing is a straightforward process. By following these steps, you can get Playwright running in your environment and start writing tests efficiently.
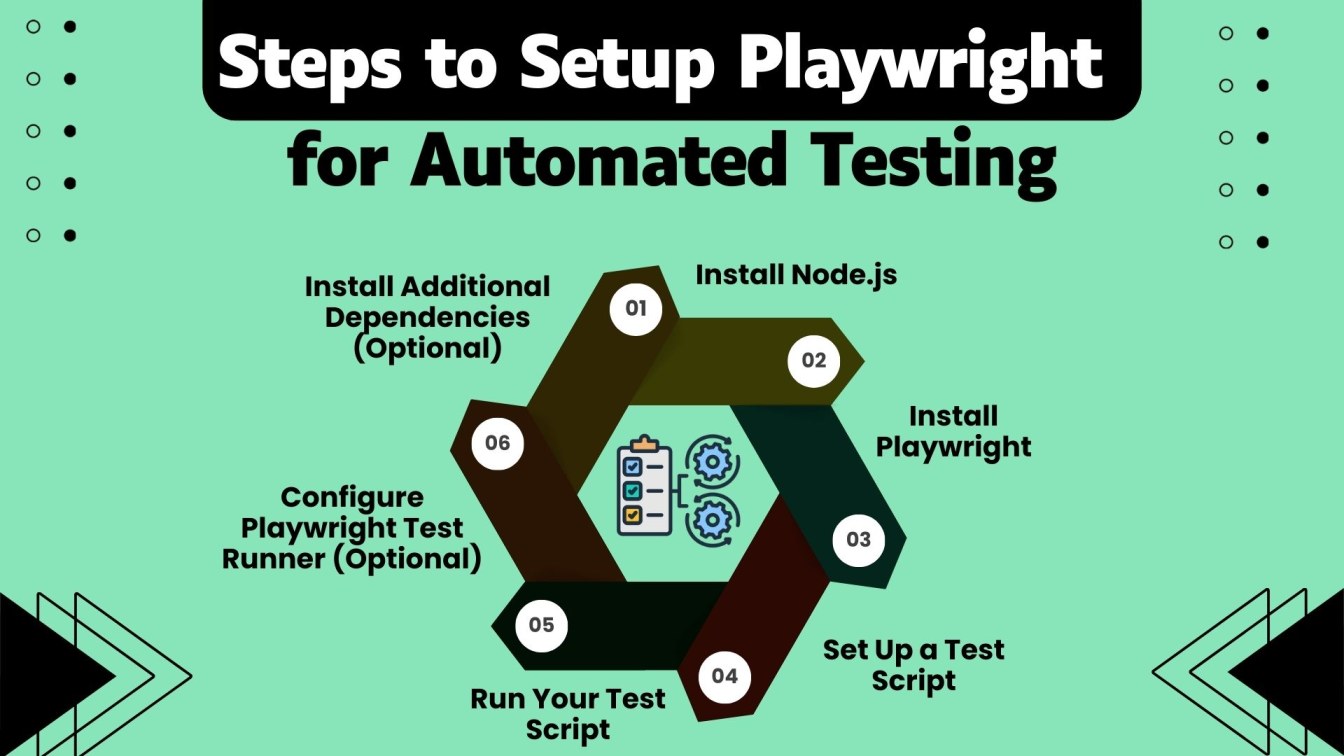
1. Install Node.js
- Playwright requires Node.js to run, so the first step is to install it on your machine.
- You can download Node.js from the official website: https://nodejs.org/
2. Install Playwright
- Open your terminal or command prompt and navigate to your project directory. If you don't have a project, create a new one using the following command:
mkdir playwright-tests
cd playwright-tests
npm init -y # Initialize a new npm project
- Install Playwright and the necessary browsers:
npm install playwright
- This command installs the Playwright package and its dependencies, including Chromium, Firefox, and WebKit browsers for testing.
3. Set Up a Test Script
- After installing Playwright, you can create a simple test script to start automating web application tests. Create a file, e.g., test.js, and add the following code:
const { chromium } = require('playwright'); // Import Playwright's chromium browser module
(async () => {
const browser = await chromium.launch(); // Launch Chromium browser
const page = await browser.newPage(); // Open a new page
await page.goto('https://example.com'); // Navigate to a URL
console.log(await page.title()); // Log the page title
await browser.close(); // Close the browser
})();
4. Run Your Test Script
- To run the test, execute the following command in your terminal:
node test.js
- If everything is set up correctly, Playwright will launch the Chromium browser, navigate to "example.com", print the page title in the terminal, and close the browser.
5. Configure Playwright Test Runner (Optional)
- For more structured testing, Playwright offers a built-in test runner. To use it, install the test runner as a dev dependency:
npm install --save-dev @playwright/test
- Create a test file (e.g., example.test.js) with the following code:
const { test, expect } = require('@playwright/test');
test('title should be correct', async ({ page }) => {
await page.goto('https://example.com');
await expect(page).toHaveTitle('Example Domain');
});
- Run the test using the Playwright Test:
npx playwright test
6. Install Additional Dependencies (Optional)
- Depending on your project needs, you may want to install other packages for assertions, reporting, or integrating with CI/CD tools. For example, for browser automation:
npm install playwright-cli
Users can install playwright via npm install playwright, pip install playwright, or npx playwright install for quick setup. Features like playwright recorder, playwright page.locator, and playwright take screenshot help in debugging. It integrates with playwright github actions and supports playwright Docker for scalable execution. Playwright mobile testing and playwright visual testing ensure broad testing capabilities across different environments.
Handling Popups and Alerts in Playwright Tests
Handling Popups in Playwright Tests
In web applications, popups are often used for various purposes, such as displaying additional information or asking users for confirmation. Playwright makes it easy to handle popups during automated testing. Popups typically open in new browser windows or tabs, and Playwright allows you to manage them by listening to events like popup. By detecting when a popup appears, you can switch to the new window or tab, interact with elements, and verify its content. For example, if you open a popup with a button, you can handle it by capturing the popup’s window and interacting with its elements.
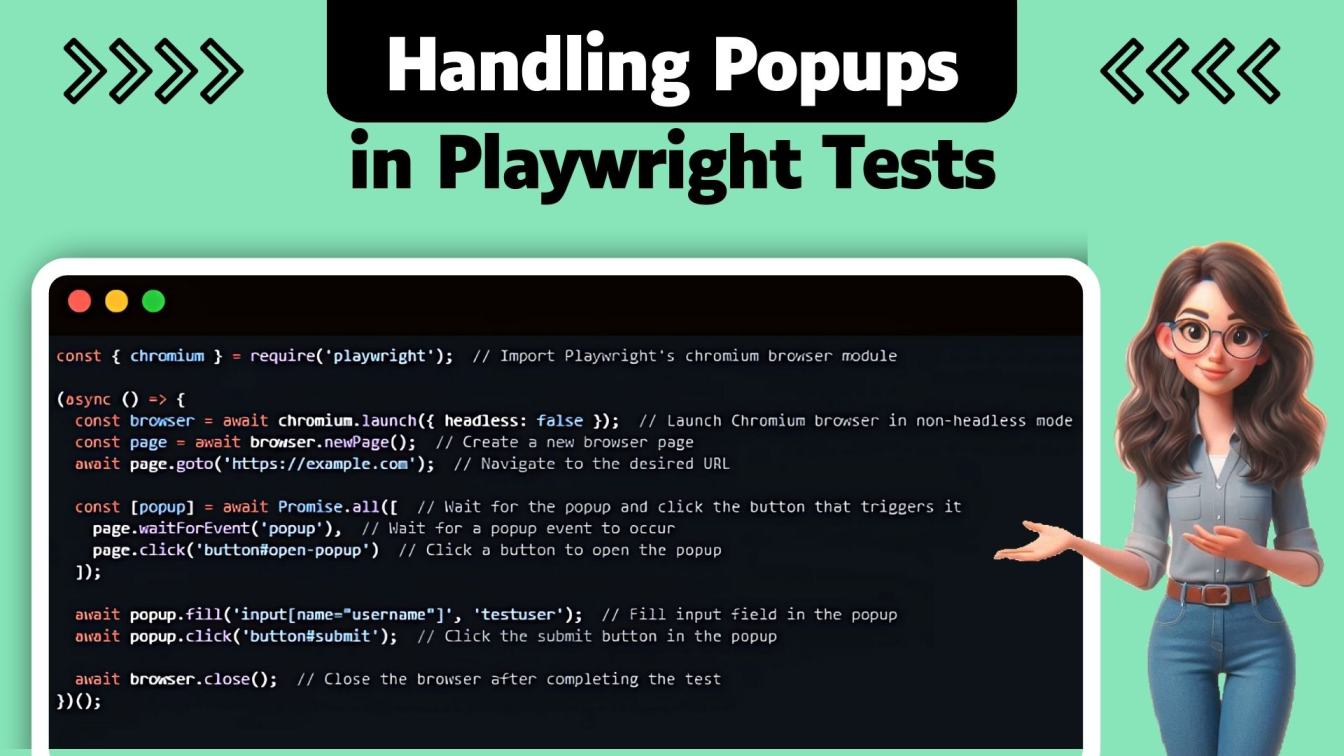
Handling Alerts in Playwright Tests
Alerts are commonly used in web applications to display notifications or messages to users. In Playwright, handling alerts is simple with the dialog event, which captures different types of dialogs, including alerts, prompts, and confirms. You can listen for the dialog event, verify the content of the alert, and take actions like accepting or dismissing the alert. Handling alerts in Playwright ensures that you can test how your application responds to these dialogues without manual intervention.

How to Write Effective Tests with Playwright
Writing effective tests with Playwright requires ensuring test reliability and simulating real user interactions accurately. One of the main challenges in testing is flaky tests, which can occur due to unreliable waits or improper handling of asynchronous actions. To minimize this, Playwright’s built-in mechanisms like waitForSelector or waitForEvent should be used to ensure elements are properly loaded before interacting with them.
Playwright enables simulating real user interactions like mouse movements, clicks, and keyboard inputs, which are crucial for testing dynamic web applications. Writing tests that mimic actual user behavior leads to more reliable results and ensures a seamless user experience.
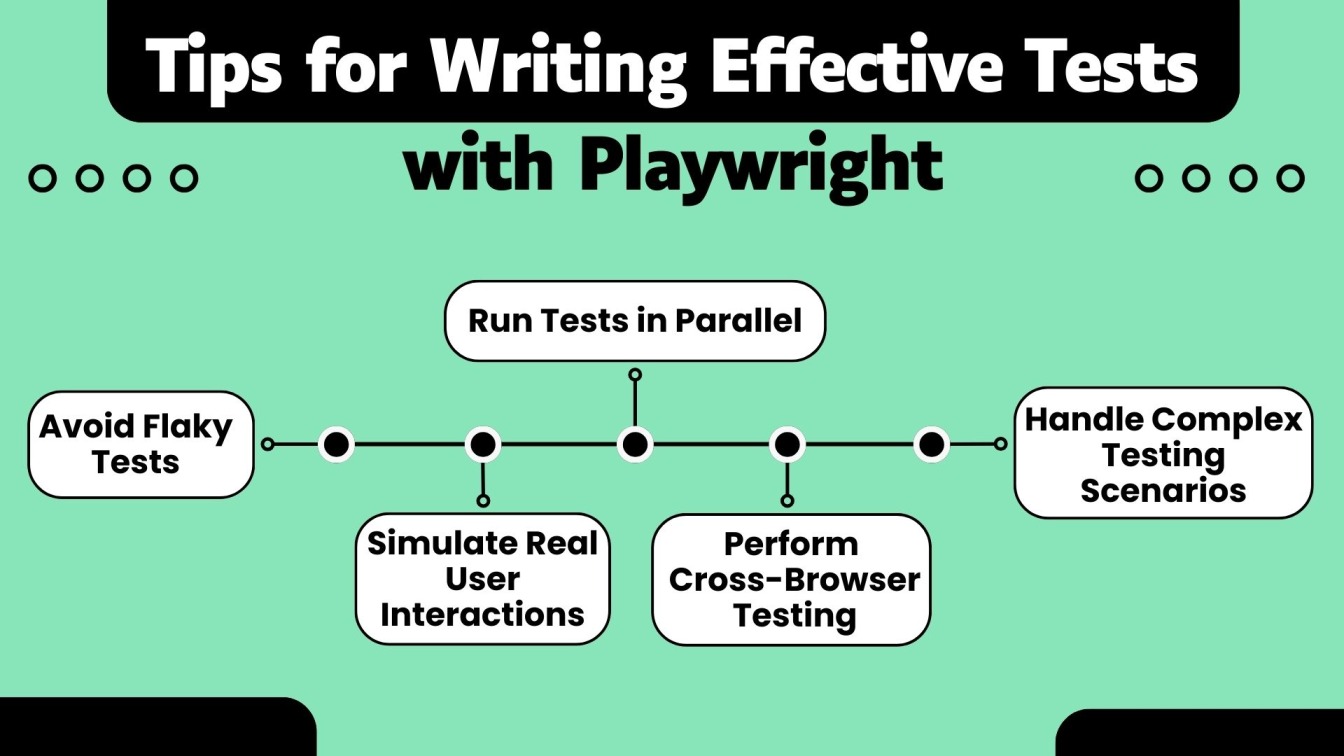
Playwright offers powerful support for running tests in parallel, allowing you to test across multiple browser instances, speeding up the execution process. It also supports cross-browser testing on a wide range of browsers, including Chromium, Firefox, and WebKit, ensuring that your web application performs consistently across major browsers.
Managing Browser Contexts and Pages with Playwright
Managing Browser Contexts with Playwright
In Playwright, a browser context represents an isolated environment within a browser, where each context can have its own cookies, cache, and session data. This allows for simulating multiple users or different sessions within the same browser instance, which is especially useful for testing scenarios like login/logout, multi-tab browsing, or handling multiple users interacting with the application simultaneously.
By using browser contexts, you can maintain test independence and avoid any data conflicts between different user sessions. Playwright allows you to create and manage these contexts easily, ensuring that each test remains isolated and reliable.
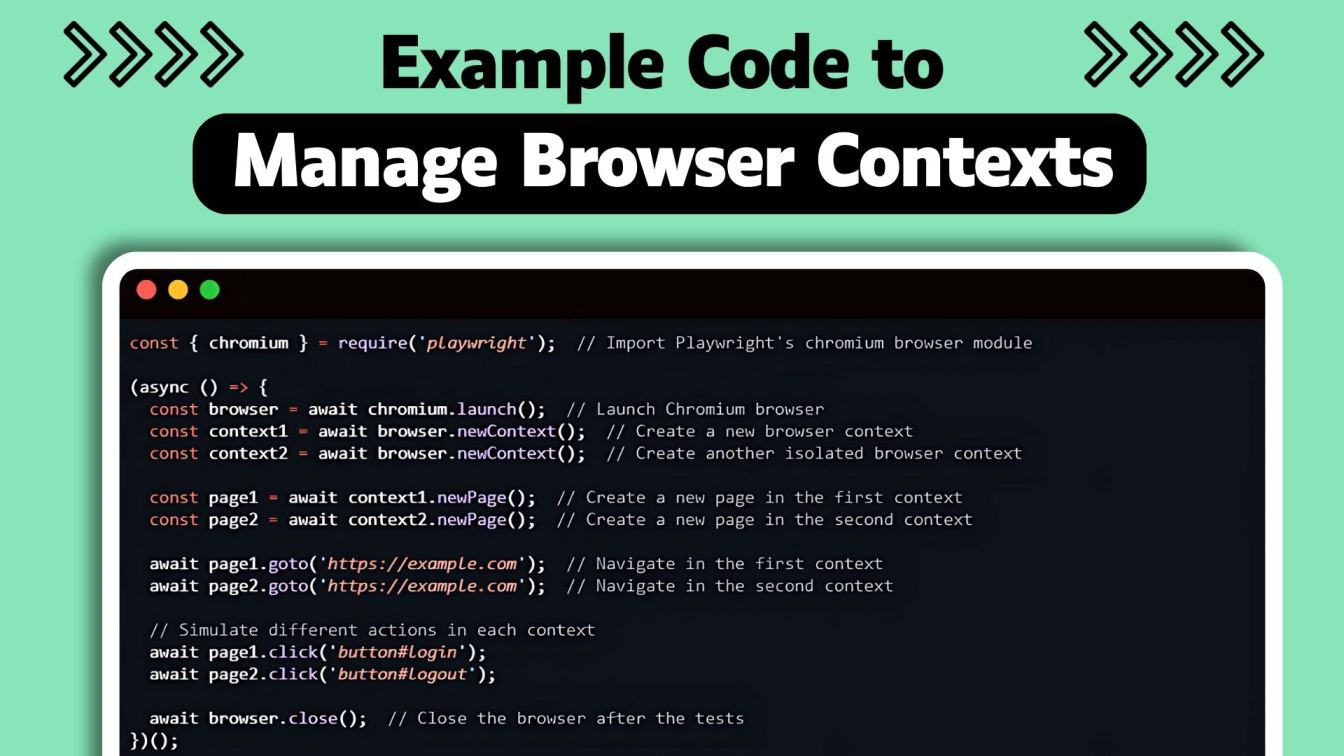
Managing Pages with Playwright
In Playwright, a page represents a single tab within a browser context. You can interact with pages individually, and perform actions like navigation, form submission, and element interactions.
Playwright allows you to create multiple pages within the same browser context, enabling you to simulate scenarios such as interacting with multiple tabs or windows.
Managing pages effectively helps in scenarios where you need to verify behavior across multiple tabs, such as verifying data between different parts of the application or simulating user workflows across different browser windows.
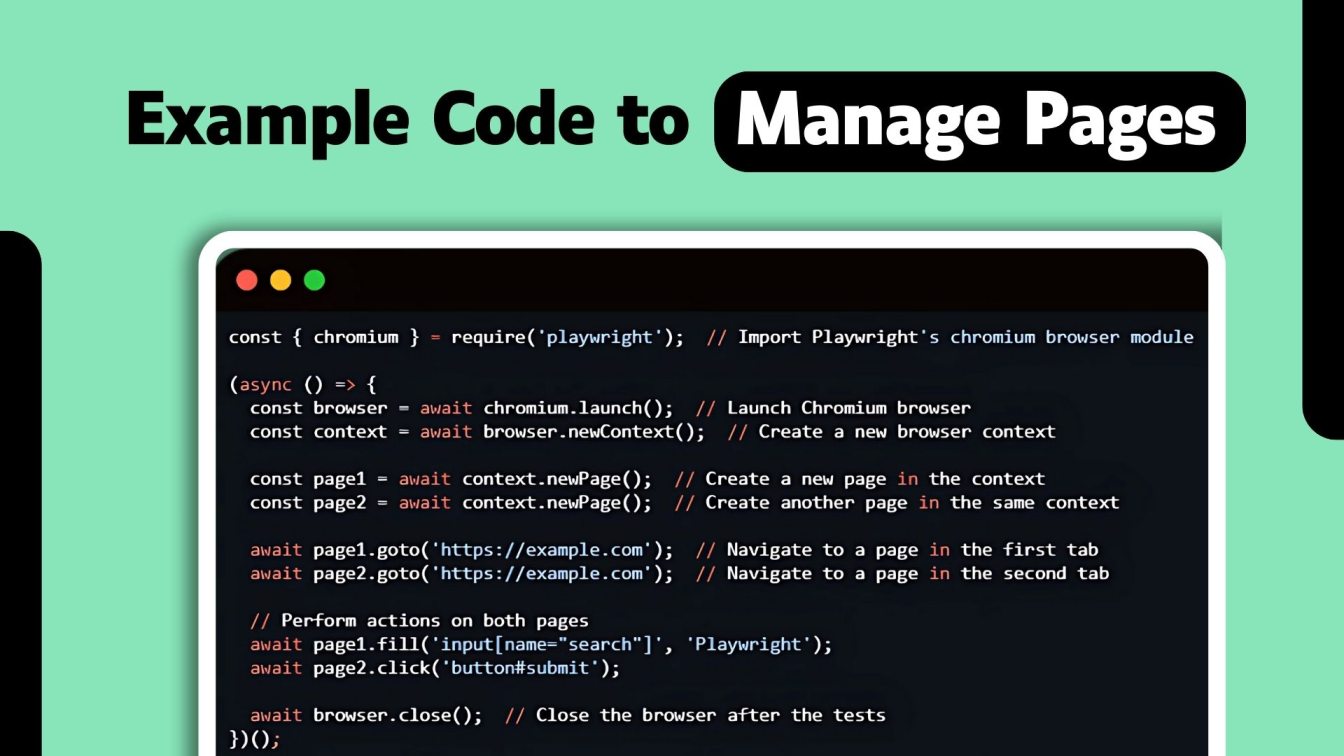
Tips for Optimizing Playwright Test Performance
Optimizing the performance of Playwright tests is crucial for reducing execution time and improving test efficiency, especially when testing large web applications or running tests across multiple browsers.
One key to improving performance is minimizing unnecessary waits and using Playwright’s built-in mechanisms for waiting for elements or events. By using the automatic waits for elements to load or actions to complete, tests can run faster and more reliably.
Additionally, running tests in parallel across multiple browsers or contexts can significantly speed up test execution, especially when multiple independent tests can be executed simultaneously.
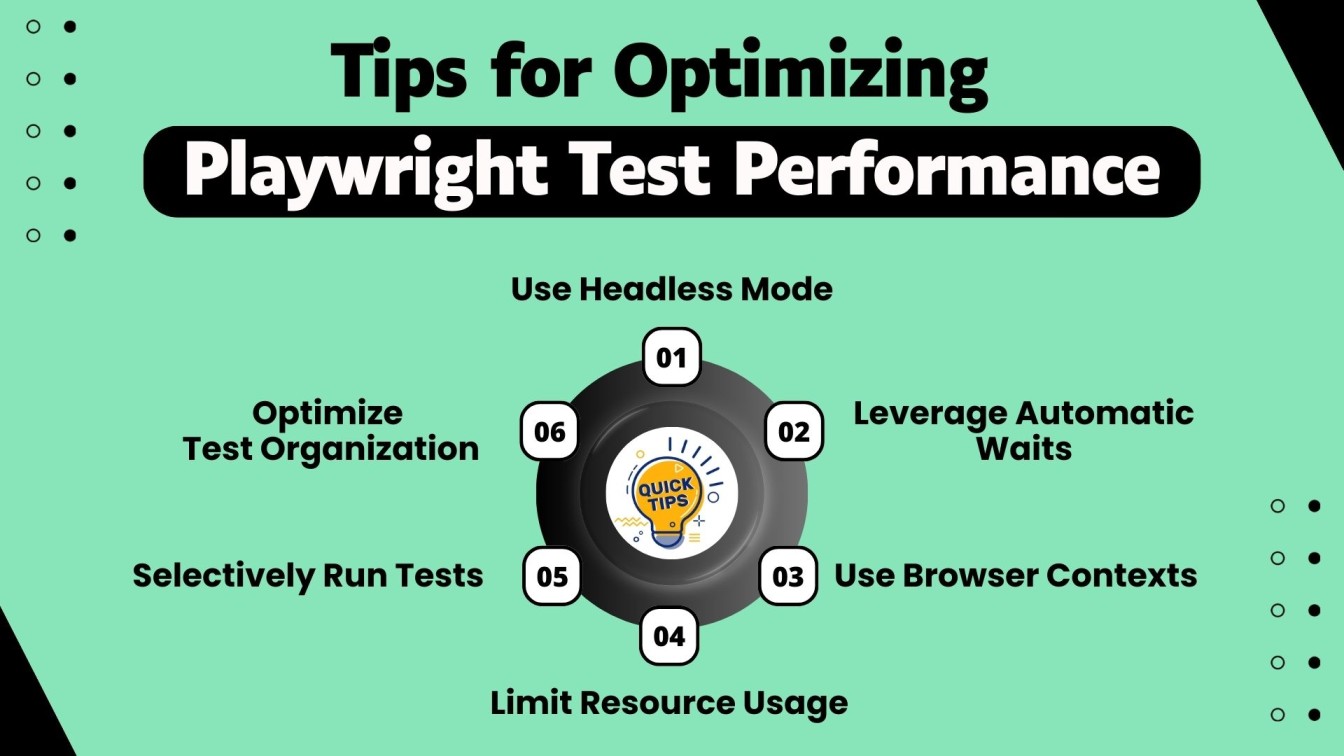
Another effective way to optimize performance is by using browser contexts to isolate tests, which can avoid the overhead of launching a new browser instance for each test. Playwright also provides the ability to configure headless browsers, which run faster than their head counterparts as they don’t render the UI.
Combining these strategies with efficient test organization and selective test execution can help streamline your testing process, ensuring both faster and more efficient results.
Key Features of Playwright for Web Application Testing
Playwright is a powerful open-source framework designed for modern web application testing. One of its standout capabilities is cross-browser testing, which allows you to test your web applications on major browsers like Chromium, Firefox, and WebKit.
This makes Playwright an excellent choice for ensuring consistent behavior across different browsers, reducing the chance of bugs related to browser-specific quirks.
Additionally, Playwright simplifies parallel testing, enabling you to run multiple tests concurrently, significantly reducing the overall testing time and making it suitable for larger applications with extensive test suites.

For example, GitHub Actions can be integrated with Playwright to automate tests across different browsers and environments, ensuring continuous testing in your CI/CD pipeline.
Furthermore, Playwright is ideal for writing integration tests that ensure various parts of your application work together seamlessly. It allows you to simulate real user interactions and test how components interact with each other.
Integrating Playwright with CI/CD Pipelines for Continuous Testing
Integrating Playwright with a continuous integration pipeline is crucial for automating web application testing throughout the development lifecycle.
By adding Playwright to your CI/CD pipeline, you ensure that tests are run automatically whenever new code is committed, providing immediate feedback on code quality. This integration helps catch bugs and issues early, improving code quality and ensuring that your application remains reliable as it evolves.
To integrate Playwright into your pipeline, you'll need to set up a configuration file that specifies the testing environment, such as the browsers to test on, the commands to run, and any necessary environment variables. This configuration file will automate the setup process and streamline the execution of Playwright tests in your CI/CD tools, such as Jenkins, GitHub Actions, or GitLab CI.
Bringing to Close!!!
In this comprehensive guide, we explored how Playwright has become a leading choice for end-to-end testing of modern web applications. With its powerful capabilities, Playwright enables cross-browser testing, faster parallel test execution, and seamless integration into CI/CD pipelines for continuous testing.
We discussed how Playwright stands out from other automation tools like Selenium, particularly for testing dynamic web applications, handling popups, and alerts, and managing complex browser contexts and pages.
We also covered the setup process for Playwright, its ability to handle authentication flows, and how its open-source framework can be easily integrated with tools like GitHub Actions.
Moreover, we looked at key best practices to optimize Playwright test performance and enhance test reliability. By leveraging Playwright’s unique features, you can streamline testing workflows, improve code quality, and ensure that your web applications work consistently across different browsers, providing a seamless user experience.
For working on mobile testing, integration tests, or large-scale testing projects, Playwright is an indispensable tool for modern web application testing.
Many QA testing companies rely on Playwright for automated browser testing, ensuring smooth user interactions. Additionally, businesses exploring Reqtest pricing can evaluate test management solutions for better test planning and execution.

People Also Ask
👉Is end-to-end testing part of UAT?
Yes, end-to-end testing is often a part of User Acceptance Testing (UAT), as it ensures the entire application works as intended from the user's perspective.
👉Can we automate a Windows application using Playwright?
No, Playwright is primarily designed for automating web applications in browsers and does not support Windows desktop application automation.
👉Can Playwright be used for performance testing?
While Playwright is excellent for functional and end-to-end testing, it is not specifically designed for performance testing; tools like JMeter or Gatling are better suited for this purpose.
👉Which script language is best for automation?
The best scripting language for automation depends on the tool you are using, but JavaScript (for tools like Playwright) and Python (for Selenium) are among the most commonly used.
👉Can Jest be used for end-to-end testing?
Yes, Jest can be used for end-to-end testing with integrations like Puppeteer or Playwright, although it is primarily designed for unit and integration testing.