APIs (Application Programming Interfaces) are the backbone of modern applications, enabling seamless communication between different software applications. To ensure API functionality, security, and performance, comprehensive API testing is essential. This guide covers the API testing process, explains its importance, and provides a detailed API testing checklist using industry-leading API testing tools like Postman.
Why read this blog on API Testing Checklist and Best Practices?
📌 Comprehensive API testing checklist – Covers essential steps for validating API functionality, security, and performance.
📌 Automated API testing strategies – Learn how to use Postman, REST API testing tools, and automation frameworks for efficient API validation.
📌 API security testing best practices – Protect APIs from SQL injection, XSS, unauthorized access, and other security vulnerabilities.
📌 Regression testing and performance benchmarks – Ensure APIs maintain stability with continuous integration and load testing techniques.
📌 Authentication and authorization validation – Understand OAuth, API tokens, and multi-factor authentication to secure endpoints.
With an in-depth API testing checklist, security guidelines, and automation tools, this guide is essential for ensuring seamless integration and reliability in modern applications.
Introduction to API Testing
API testing is a critical process that evaluates the functionality, security, performance, and reliability of API endpoints. Unlike traditional UI testing, which focuses on the user interface, API tests directly validate the request methods, response payloads, and status codes at the backend.
Key Aspects of API Testing
- Functional Testing: Ensures that APIs return the expected API responses for valid requests.
- Security Testing: Identifies security vulnerabilities, unauthorized access, and injection attacks.
- Performance Testing: Assesses response times, performance benchmarks, and potential bottlenecks.
- Regression Testing: Detects issues after software updates using regression testing tools.
- Load Testing: Simulates concurrent users to measure the API’s ability to handle traffic spikes.

Popular API testing tools include Postman, SoapUI, and JMeter, which help automate REST API testing and ensure seamless integration within the development lifecycle

Postman is ideal for functional API testing with an easy-to-use interface, while SoapUI offers flexibility for automation and scripting. JMeter is best suited for performance and load testing, making it a strong choice for large-scale applications.
Why API Testing is Crucial?
The API testing process is an essential component of software quality assurance, ensuring that APIs function reliably, securely, and efficiently. Here’s why testing of APIs is indispensable:
1. Ensuring API Functionality and Reliability
APIs serve as the foundation for software applications, enabling communication between application endpoints. Functional testing ensures that APIs deliver valid credentials, accurate response bodies, and detailed error messages, preventing issues like invalid requests or performance degradation.
2. Strengthening API Security
APIs are a prime target for cyber threats such as injection attacks, unauthorized access, and token generation misuse. Conducting API security testing helps detect common vulnerabilities and ensures access control mechanisms like multi-factor authentication are implemented correctly.
3. Improving Performance and Load Handling
APIs must handle high volumes of traffic efficiently. Load testing and stress testing measure API response times, identifying potential bottlenecks that could impact concurrent users. Tools like Postman test API capabilities allow testers to conduct load test API Postman scenarios to assess performance under different traffic patterns.
4. Enhancing Continuous Integration and Regression Testing
Continuous testing ensures that every API update maintains its intended functionality. Automated API security testing tools allow teams to execute regression testing efficiently, preventing financial losses due to faulty deployments.
5. Optimizing API Error Handling and Response Codes
Proper error handling improves user experience by providing meaningful error messages and correct status codes. This ensures that developers and consumers of the API can quickly diagnose issues and apply fixes.
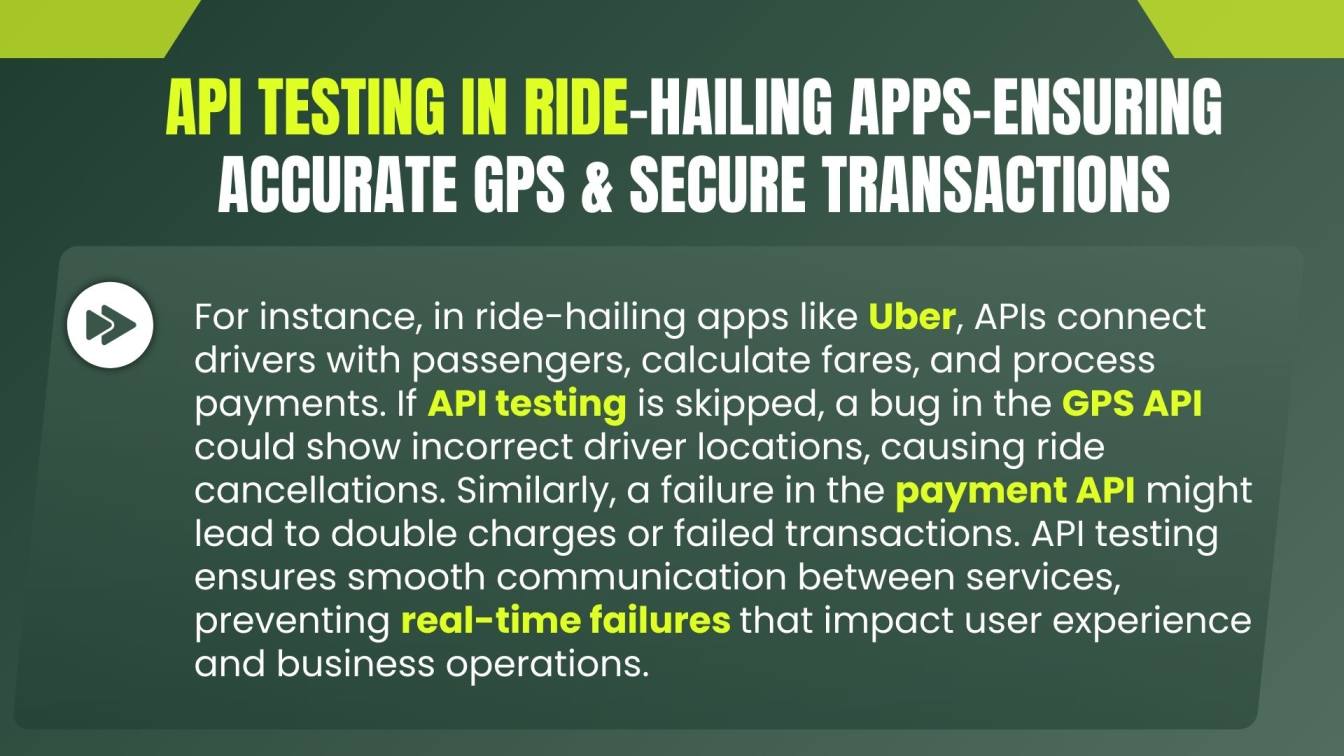
API Testing Checklist
A well-defined API testing checklist ensures that all critical areas of an API are tested systematically. Below are essential steps to perform comprehensive API testing:
1. Understand API Requirements and Specifications
- Review API documentation, endpoints, request parameters, and expected responses.
- Ensure API specifications align with business requirements and functional needs.
2. Validate API Requests and Responses
- Ensure the request method (GET, POST, PUT, DELETE) performs the intended operation.
- Verify API responses match expected response payloads with correct status codes (e.g., 200 OK, 400 Bad Request, 401 Unauthorized, 500 Internal Server Error).
3. Perform API Security Testing
- Check for unauthorized access and validate authentication tokens.
- Test for API security vulnerabilities such as injection attacks, broken authentication, and weak access control.
- Use the best API security testing tools to automate penetration testing.
4. Conduct Functional Testing
- Execute test scenarios covering valid inputs, invalid inputs, and boundary conditions.
- Ensure that APIs return detailed error messages when an invalid request is sent.
- Use Postman’s REST API testing capabilities to efficiently test API functions.
5. Implement Regression Testing
- Utilize regression testing tools to verify API stability after code changes.
- Automate test cases using automated rest API testing solutions.
- Maintain a structured testing suite to track test results.
6. Perform Load and Stress Testing
- Simulate multiple concurrent users to evaluate performance benchmarks.
- Identify potential bottlenecks using load testing tools.
- Use JMeter to test APIs under different traffic patterns.
7. Validate Security Mechanisms
- Ensure API authentication is properly enforced.
- Verify multi-factor authentication and token generation processes.
- Implement an API security testing checklist to cover all security aspects.
8. Check API Documentation and Compliance
- Ensure the API documentation is updated with response codes, request methods, and security requirements.
- Test compliance with industry standards such as OAuth 2.0 for authentication.
- Verify third-party API interactions for seamless software integration.
By following this API testing checklist, teams can improve API security, reliability, and overall user satisfaction.

Understand API Requirements and Specifications
Before conducting API tests, it's essential to have a thorough understanding of the API requirements and specifications. A well-defined Application Programming Interface (API) ensures seamless communication between systems, reducing the chances of invalid requests and security vulnerabilities.
Key Aspects of API Requirements:
- Business Requirements: Understanding the API business logic and expected functionality ensures alignment with user needs.
- API Endpoints: Define all available API requests, their request methods, and expected API responses.
- Authentication Mechanisms: Determine if API authentication requires multi-factor authentication or authentication tokens.
- Security Requirements: List all potential threats, such as unauthorized access, injection attacks, and security breaches.
- Performance Benchmarks: Define acceptable response times, limits for concurrent users, and potential bottlenecks.

Verify HTTP Methods (GET, POST, PUT, DELETE, etc.):
API testing test cases should validate that each request method is implemented correctly. The HTTP methods commonly used in REST API testing include:
- GET: Retrieves data from the API endpoint.
- POST: Submits new data to the server.
- PUT: Updates existing data.
- DELETE: Removes data from the system.
How to Test HTTP Methods?
- Use Postman to test API requests, verifying that the correct status code is returned.
- Validate API responses for different request methods using rest API testing tools.
- Ensure that unauthorized users cannot modify or delete data (access control validation).
- Perform regression testing after every API update to prevent unintended changes.
Testing each request method prevents security risks and ensures seamless integration within the development lifecycle.
Check Response Status Codes and Messages
Validating response codes is critical to ensuring that APIs communicate errors and successes properly. Standard status codes include:
- 200 OK: Successful request.
- 400 Bad Request: Invalid input from the user.
- 401 Unauthorized: Missing or incorrect authentication.
- 403 Forbidden: Access control prevents the request.
- 404 Not Found: Invalid API endpoint.
- 500 Internal Server Error: Unexpected server failure.
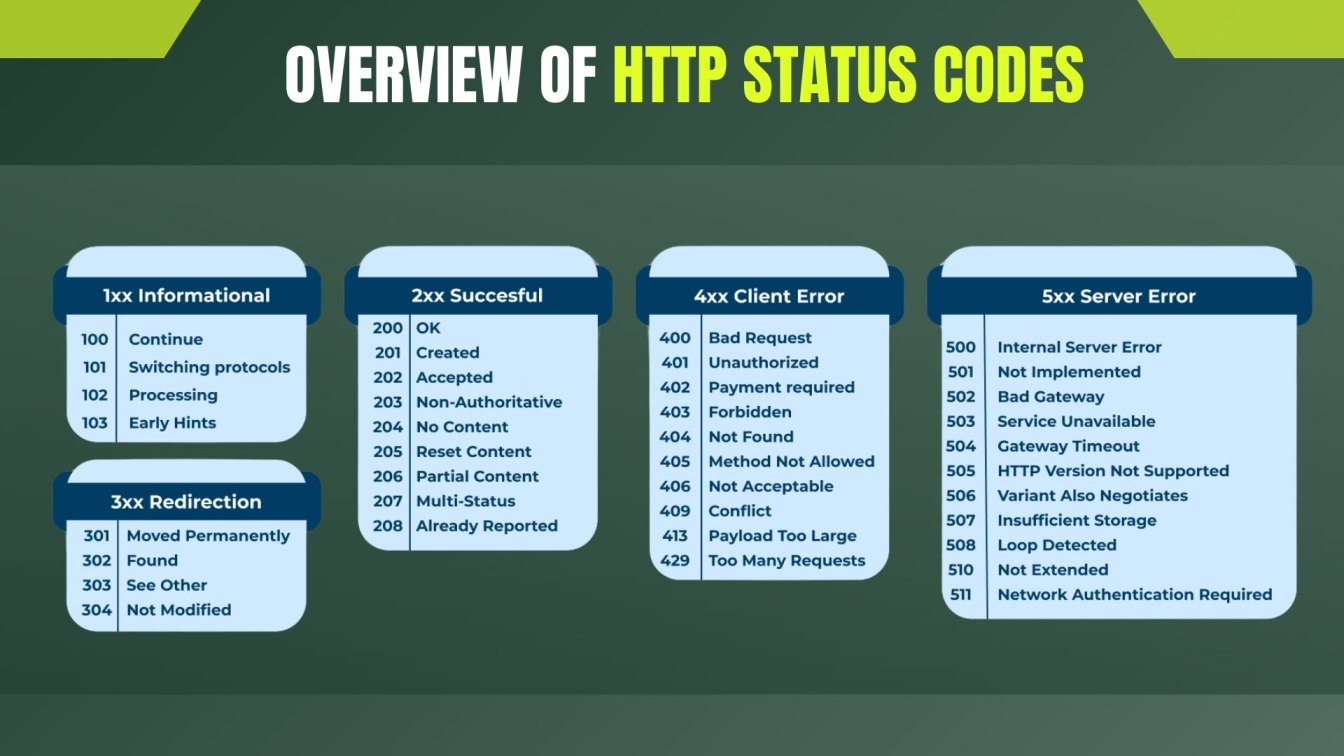
Best Practices for Validating Status Codes:
- Use Postman’s API testing capabilities to confirm correct responses.
- Implement automated REST API testing to check responses at scale.
- Ensure detailed error messages provide clear explanations of failures.
By validating response codes, testers can improve user experience while preventing security weaknesses in API interactions.
Validate Request and Response Payloads
A well-structured request payload ensures the correct data is sent to an API endpoint, while a proper response payload confirms that the API returns the expected results.
Testing Request and Response Payloads
- Use Postman’s REST API testing to validate response bodies.
- Implement validation testing to ensure data accuracy.
- Conduct security testing to detect invalid inputs that could cause cyber threats.
- Check API functionality by validating field formats, data types, and required parameters.
By thoroughly testing request and response payloads, testers can identify potential security risks and improve API accuracy.
Ensure Proper Authentication and Authorization
API authentication ensures that only legitimate users can access protected data, while authorization defines what actions they can perform. Weak authentication can lead to broken authentication attacks, compromising user data.
API Authentication Methods
- Token Generation: APIs should require authentication tokens to validate requests.
- OAuth 2.0: A secure authentication mechanism used by major applications.
- Multi-Factor Authentication (MFA): Adds an extra layer of security.

API Authorization Checks
- Ensure role-based access control is enforced.
- Prevent unauthorized access to sensitive data.
- Conduct security tests using best API security testing tools.

Implementing authentication and authorization properly prevents security breaches and protects APIs from potential threats.
Test for Performance and Load Handling
API performance benchmarks must be met to ensure smooth operation under high traffic. Load testing helps determine how an API handles concurrent users and traffic patterns.
Performance Testing Strategies:
- Load Test API Postman: Simulate multiple requests to check for performance degradation.
- Stress Testing: Push the API beyond its limits to measure resilience.
- Monitor Response Times: Ensure APIs respond within acceptable time frames.
By integrating continuous testing with automated rest API testing, developers can identify potential bottlenecks before they impact users.
Implement Security Testing (SQL Injection, XSS, etc.)
APIs are vulnerable to security risks such as SQL Injection, Cross-Site Scripting (XSS), and unauthorized access. Implementing API security testing helps detect these common vulnerabilities.
Security Testing Checklist
✔ Use security testing tools to detect injection attacks.
✔ Validate user input to prevent XSS and invalid requests.
✔ Check detailed error messages for sensitive data leaks.
✔ Perform penetration testing to identify security weaknesses.

Automated API security testing ensures that vulnerabilities are addressed before attackers exploit them.
Monitor API Rate Limits and Throttling
APIs often enforce rate limits to prevent security breaches and ensure fair resource allocation. Rate limiting restricts how often a user or application can make API calls.
How to Test API Rate Limits?
- Simulate excessive requests using testing suite tools.
- Check if the API returns a 429 Too Many Requests error when limits are exceeded.
- Ensure rate limits are applied fairly across application endpoints.
Monitoring API rate limits helps protect against security threats like DDoS attacks and unauthorized access.
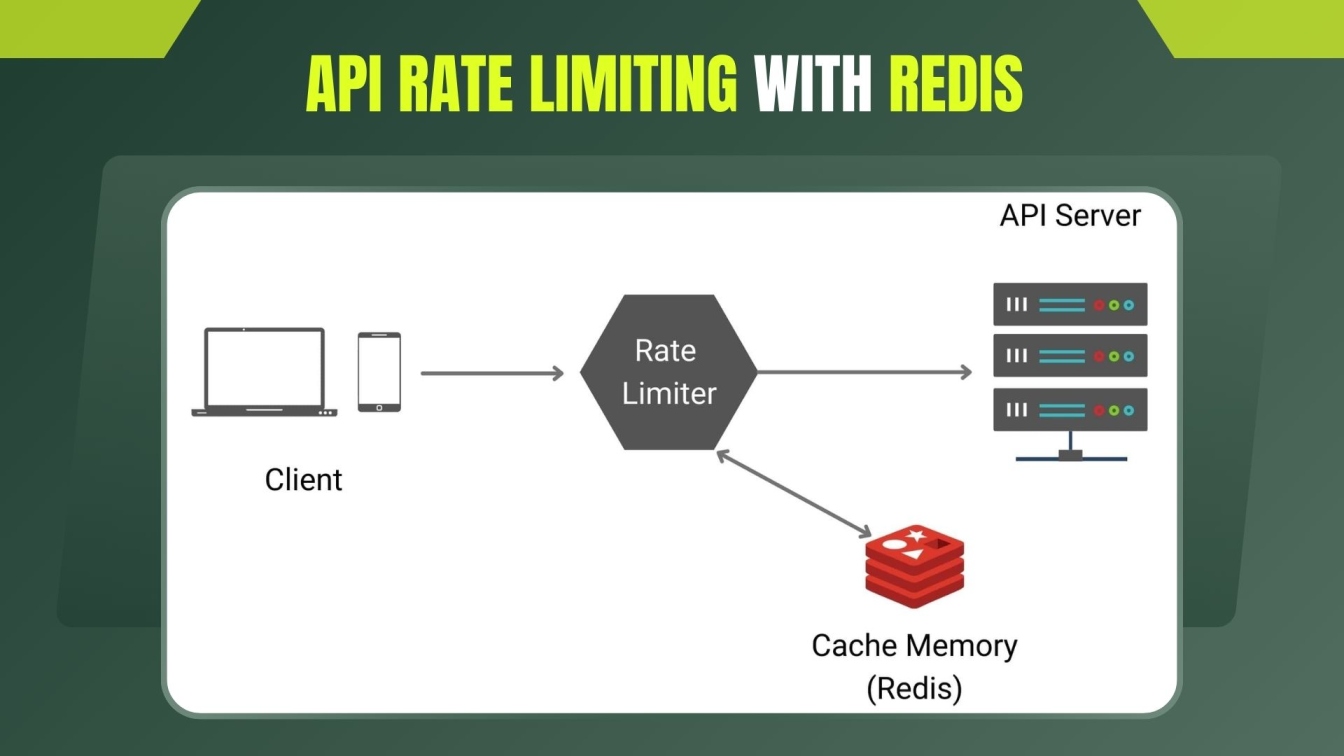
Validate Error Handling and Edge Cases
Robust error handling enhances user experience by providing meaningful error messages instead of vague responses. Testing edge cases ensures that APIs handle invalid inputs gracefully.
Best Practices for API Error Handling
- Verify APIs return detailed error messages instead of generic ones.
- Test invalid request scenarios to ensure the API responds correctly.
- Conduct regression testing to validate error handling after updates.
By testing for error responses and edge cases, developers can improve API reliability and security.
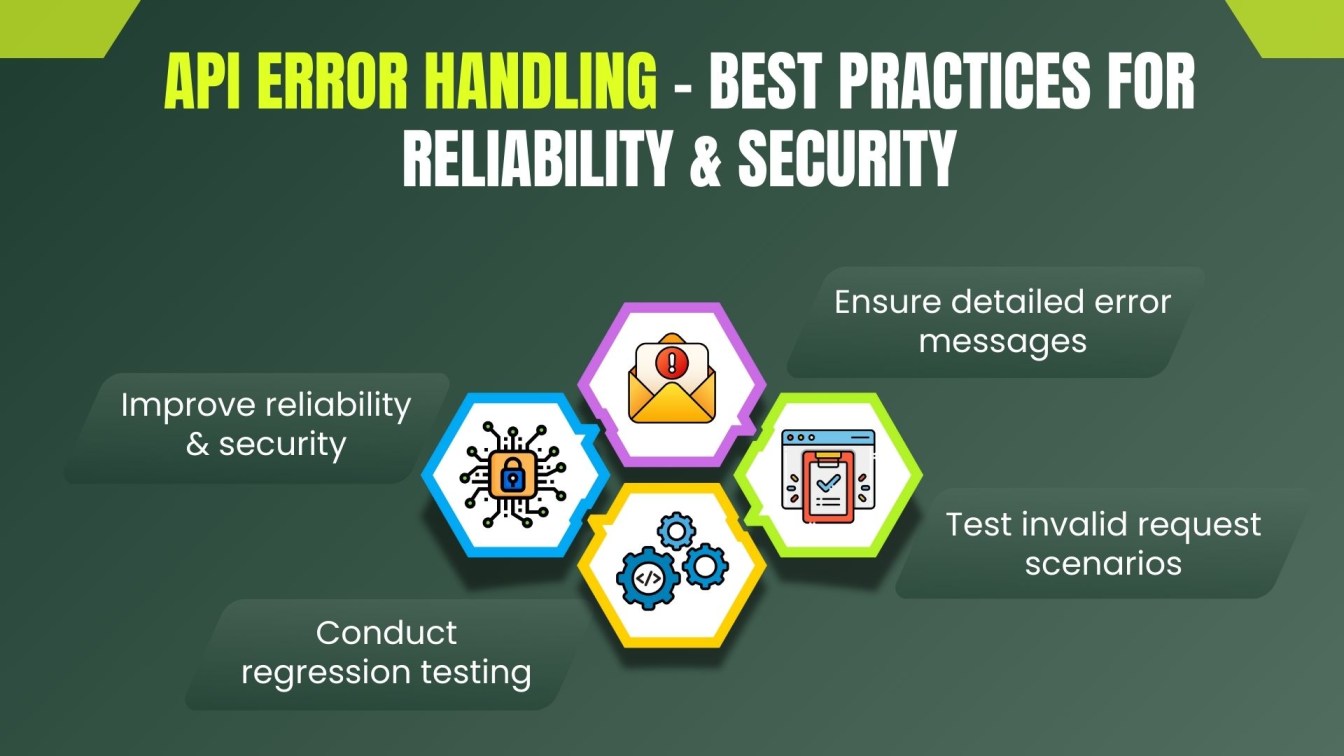
Best Practices for API Testing
API testing is a crucial step in ensuring Application Programming Interface (API) functionality, security, and performance. Implementing best practices can help teams conduct comprehensive API testing efficiently, minimizing potential threats and ensuring seamless integration in the development lifecycle.
Key Best Practices:
- Understand API Requirements: Review API specifications, request/response formats, and expected behaviors before testing.
- Validate All Request Methods: Ensure that GET, POST, PUT, DELETE, and PATCH methods function as intended.
- Test with Both Valid and Invalid Inputs: Check how the API handles edge cases, incorrect data, and unexpected inputs.
- Perform Security Testing: Validate authentication, authorization, and protection against common vulnerabilities like SQL injection, XSS, and CSRF.
- Automate API Tests: Use tools like Postman, SoapUI, and JMeter to automate functional, security, and performance testing.
- Check API Performance: Conduct load and stress testing to measure API response times under different conditions.
- Ensure Proper Error Handling: APIs should return meaningful error messages and appropriate status codes (e.g., 400 Bad Request, 401 Unauthorized).
- Maintain Up-to-Date API Documentation: Ensure that API documentation reflects the latest changes and includes examples for easy integration.
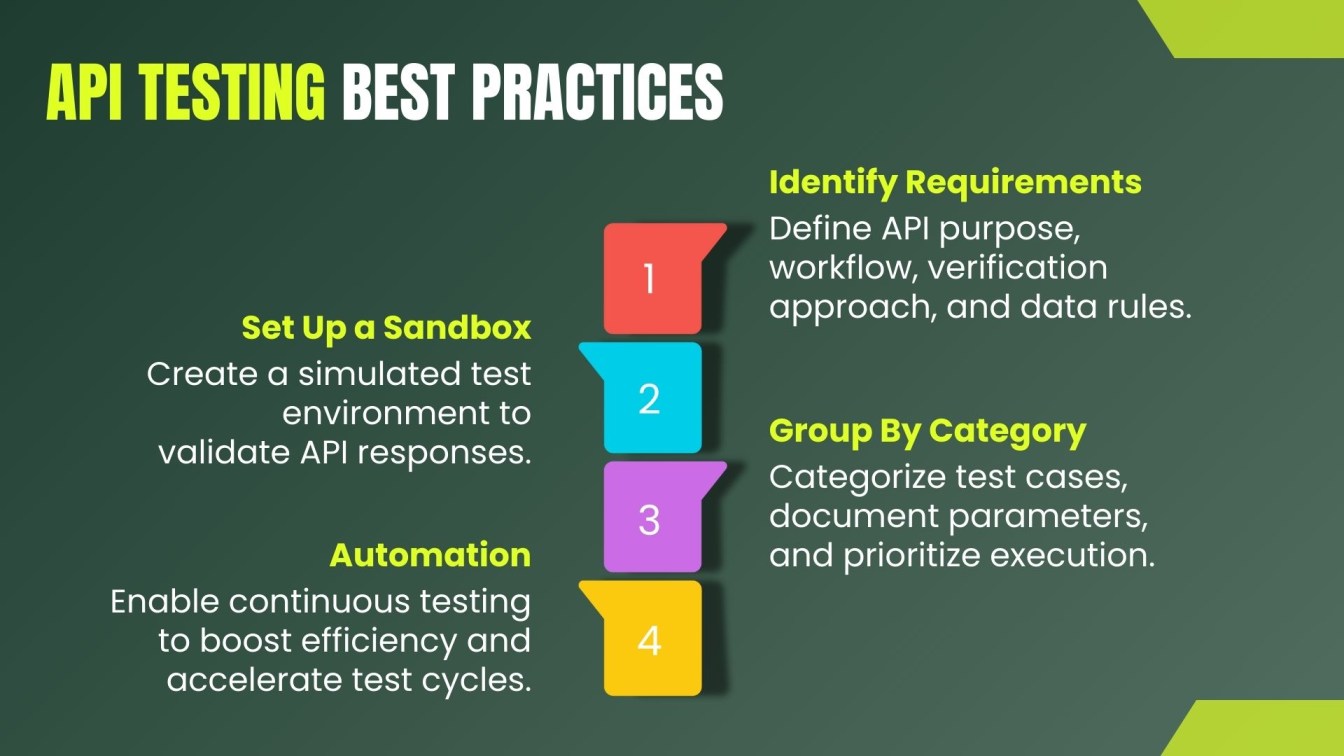
Use Automation for Repetitive Tests
Automated API testing plays a vital role in continuous integration and continuous testing, enabling teams to run multiple API tests efficiently. Manual testing can be time-consuming, especially when verifying multiple API endpoints for different request methods, such as GET, POST, PUT, DELETE.
Benefits of Automated API Testing
- Faster Execution: Automate REST API testing to reduce human effort.
- Improved Accuracy: Eliminate errors in repetitive API testing test cases.
- Scalability: Run tests for multiple API requests simultaneously.
- Enhanced Security: Conduct automated API security testing to detect security vulnerabilities in real time.
- Regression Testing: Use regression testing tools to prevent issues after code updates.
Tools for Automating API Tests
Several API testing tools can help in automating API security testing, functional tests, and performance benchmarks:
- Postman: Widely used for Postman test API automation and REST API testing automation.
- Selenium: Useful for integrating API testing test cases with UI-based test automation.
- SoapUI: Ideal for both functional testing and security tests.
- JMeter: Great for load testing and stress testing to assess API performance under heavy traffic.
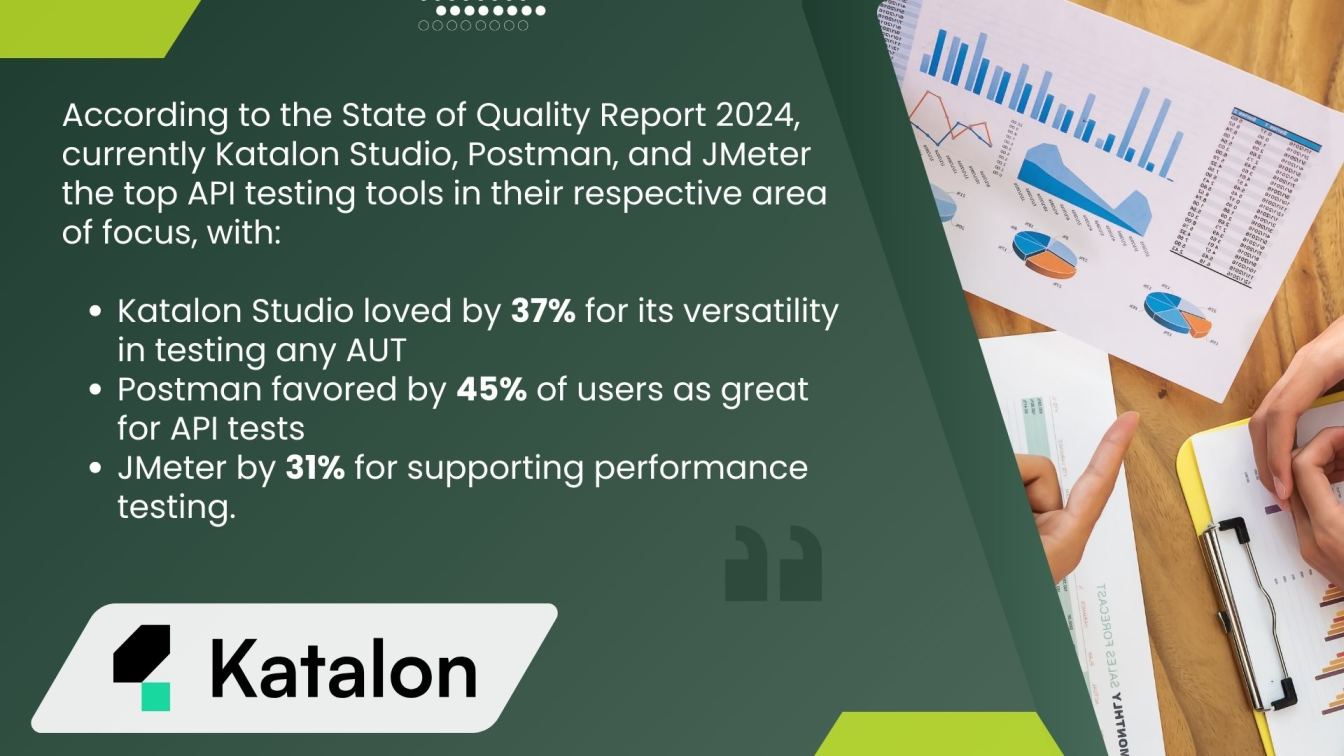
Using these testing suite tools, teams can conduct comprehensive API testing, ensuring robust validation of API functionality and security requirements.
Maintain Clear and Updated API Documentation
A well-documented API is essential for developers, testers, and stakeholders. Clear and updated API documentation ensures that everyone understands how the API works, what request methods are supported, and what response payloads to expect.
Key Components of API Documentation
- API Endpoints: List available API requests and their expected parameters.
- Request and Response Examples: Provide real-time examples to illustrate API functionality.
- Authentication Mechanism: Explain API authentication methods such as token generation, OAuth, and multi-factor authentication.
- Error Responses: Document possible error responses and their corresponding status codes.
- Rate Limits and Throttling: Clearly define API limits to prevent unauthorized access and potential bottlenecks.
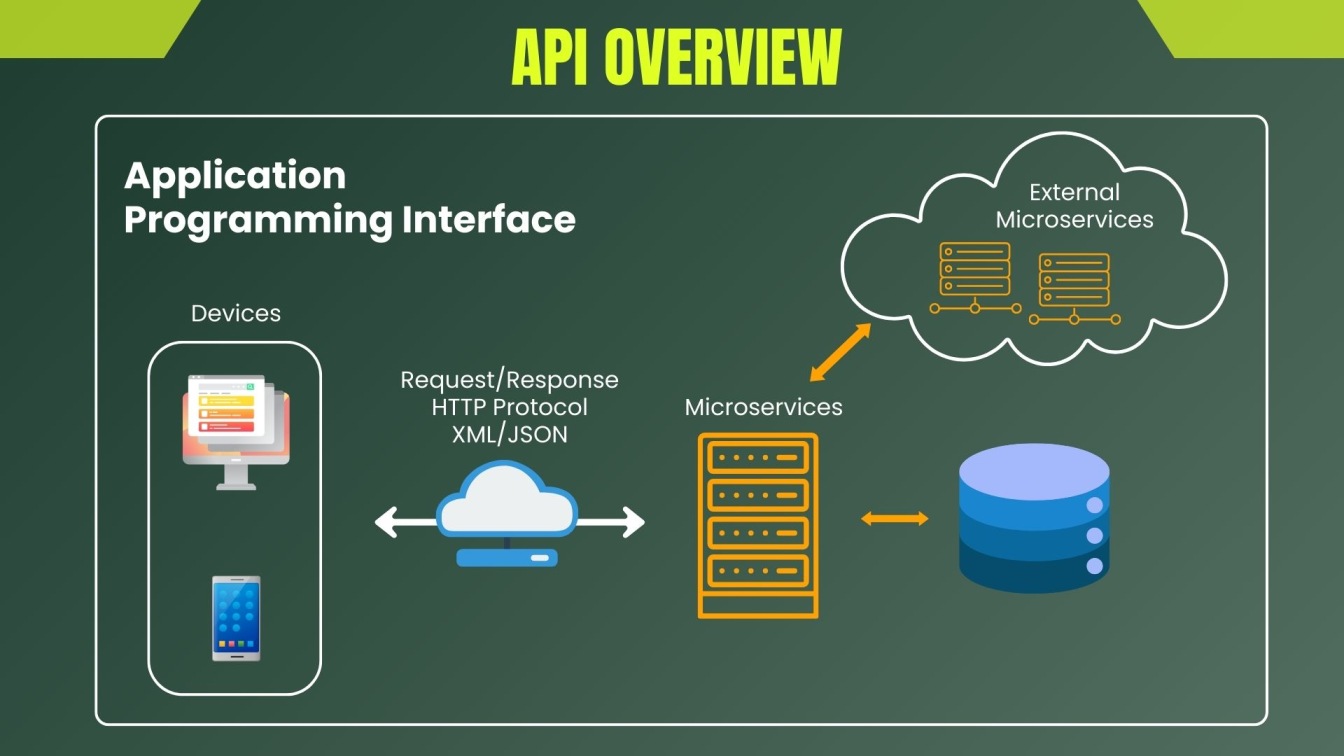
Best Practices for API Documentation
- Use tools for REST API testing like Swagger for automated documentation generation.
- Keep documentation updated with each change in the development process.
- Ensure the documentation includes security details to mitigate security risks and cyber threats.
By maintaining intuitive interface documentation, developers and testers can efficiently debug issues and improve the user experience.
Conclusion and Key Takeaways
Effective API testing ensures that modern applications remain secure, scalable, and high-performing. By following best practices such as automating repetitive tests, maintaining clear API documentation, and implementing security testing, teams can safeguard APIs against security weaknesses and performance degradation.
Final API Testing Checklist
✔ Perform functional testing to validate API accuracy.
✔ Use security testing of API to detect security vulnerabilities and prevent unauthorized access.
✔ Implement regression testing best practices to avoid breaking existing functionalities.
✔ Validate response bodies, error responses, and status codes for different request methods.
✔ Conduct penetration testing to identify common vulnerabilities, such as injection attacks.
✔ Use load testing to ensure APIs handle concurrent users without performance degradation.
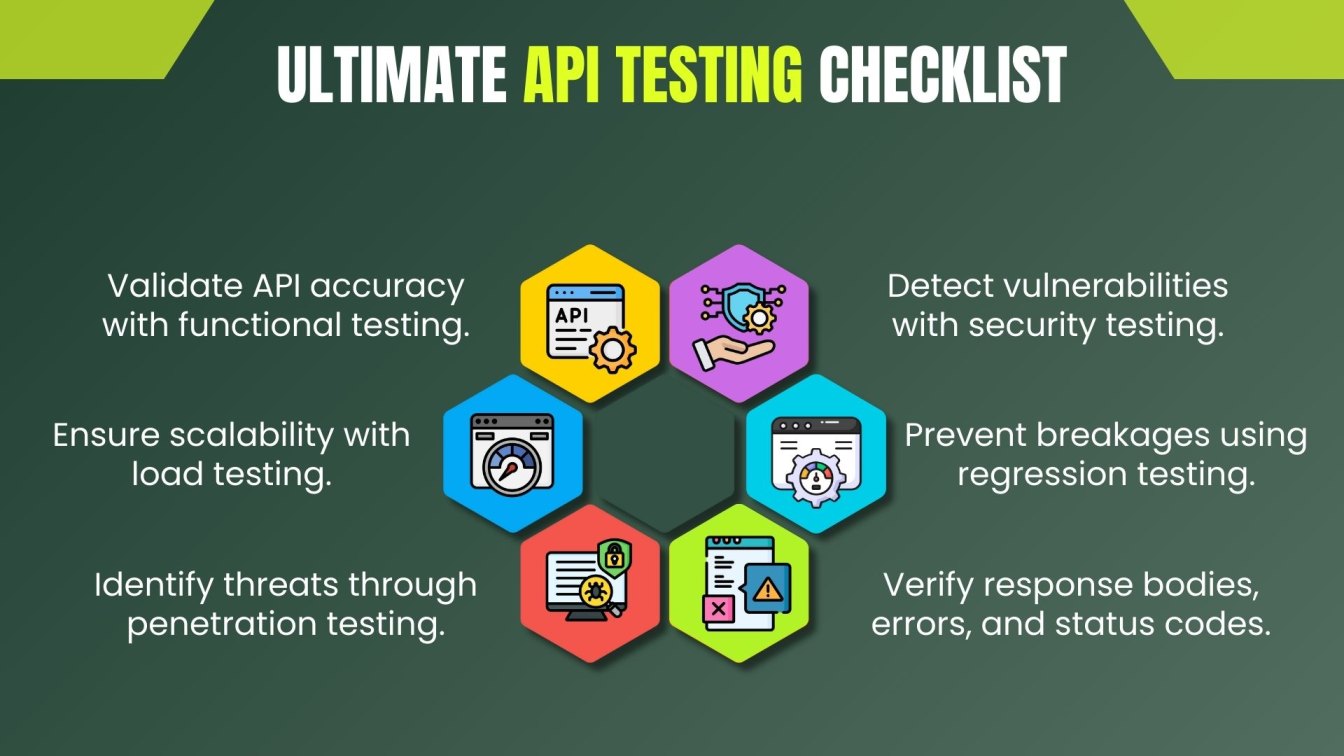
By integrating these best practices into the API testing process, businesses can reduce security risks, enhance API functionality, and provide a seamless user experience.
People Also Ask
How often should the API testing checklist be updated?
Regularly update the checklist based on new security threats, API changes, and industry best practices. Ideally, review it after major updates or quarterly.
What are the essential security measures for API testing?
Implement secure authentication (OAuth, API keys) and proper authorization controls. Encrypt sensitive data, validate user inputs to prevent injection attacks, and conduct regular security assessments to identify vulnerabilities like broken authentication and unauthorized access.
How do I handle different API versions during testing?
Maintain version-specific test cases, use automation to test backward compatibility, and ensure proper deprecation handling for older versions.
How can I ensure API reliability in distributed systems?
Perform load and stress testing, use retries and circuit breakers, and monitor API performance to handle network failures effectively.
What is the significance of contract testing in API testing?
Contract testing ensures API compatibility between services by validating request-response structures, reducing integration issues in microservices architectures.