Testing APIs is essential in today's API-driven software development to guarantee functionality, performance, and dependability. A popular Java library called REST Assured makes testing RESTful web applications easier. We'll walk you through configuring REST Assured, creating your first API test, and grasping important ideas in this blog. 🚀
Beginning API testing with REST Assured gives up a world of options, from automating intricate procedures to writing straightforward test scripts. Now let's explore the topics we will cover in this guide:
💡 What is REST Assured?: Learn the fundamentals of REST Assured and why API testing requires it.
🛠️ Setting Up REST Assured: Comprehensive guidelines for setting up REST Assured for your project.
⚙️ Writing Your First Test: Create a "Hello World" API test using REST Assured.
🔍 Key Features of REST Assured: Explore REST Assured’s powerful capabilities for testing APIs.
🚀 Making HTTP Requests: Learn how to make GET, POST, PUT, and DELETE requests.
🛡️ Assertions in REST Assured: Add assertions to validate API responses and status codes.
🚧 Common Challenges: Recognize and get beyond typical roadblocks while using REST Assured.
🔄 Integration with Other Tools: Integrate REST Assured with JUnit, TestNG, Jenkins, and Allure.
🔥 REST Assured vs Other Tools: Compare REST Assured with Postman, Karate, and Insomnia for API testing.
⚡ Advanced Techniques: Explore advanced techniques and reusable test scripts for API automation.
What is REST Assured?
A well-known Java-based package called REST Assured was created to make testing RESTful APIs easier. Since APIs are essential to contemporary applications, testers and developers want a tool that makes it easy for them to efficiently check the functionality, performance, and behaviour of APIs.
This is made possible in part by REST Assured, which offers an easy-to-use interface for dealing with APIs, facilitating the creation and upkeep of test scripts.
REST Assured gives testers the ability to do a range of tasks, such as:
- Sending HTTP requests: A variety of HTTP methods, including GET, POST, PUT, DELETE, OPTIONS, and PATCH, are supported by REST Assured. With the help of this feature, testers can engage with various API endpoint kinds and confirm how they respond in various scenarios.
- Receiving and validating responses: The application not only makes queries but also records responses from APIs and verifies different elements of the answer, including headers, the body (in both JSON and XML forms), and status codes. This makes it simple to confirm that the expected results are returned by the API.
.jpeg)
Why Choose REST Assured for API Testing?
- Simple and Readable: REST Assured makes your test cases simple to read by using a given-when-then syntax.
- No Need for Complex Setup: Libraries and manual HTTP connections are not a concern.
- Rich Assertions: It provides built-in techniques for verifying headers, JSON contents, and response status codes, among other things.
- Integration with CI Tools: Jenkins, Maven, Gradle, and other CI/CD platforms are compatible with it well.
- Supports BDD (Behaviour-Driven Development): Adheres to BDD guidelines, encouraging improved communication between developers and testers
.jpeg)
Setting Up REST Assured for Your First API Test
- Embarking on your journey with REST Assured for REST API testing begins with setting up a Maven project, where you define dependencies in the pom file to automate the testing of REST services.
- REST Assured provides an intuitive syntax that simplifies working with REST APIs and REST web services, enabling testers to send various request methods like GET, POST, PUT, and DELETE.
- By utilizing query parameters, customizing the request body, and validating response headers and response structures, you can efficiently test application programming interfaces (APIs).
- Through its domain-specific language, REST Assured allows you to assert important aspects like response codes, making it one of the most popular tools for API testing in the development environment.
- If you’re working with dynamic languages or scripting automation tests, REST Assured is ideal for automation with REST Assured and verifying REST API tests.
- Its logging capabilities also allow you to log response details, giving you deeper insights into the behavior of the API under test.
Adding REST Assured to Your Maven/Gradle Project
The first step in using REST Assured is to add the required dependencies to your project. The procedures will vary slightly depending on whether your build tool is Gradle or Maven.
.jpeg)
This guarantees that REST Assured is accessible for testing, but since it's scoped for testing, it won't be incorporated into the final build.
.jpeg)
This will enable you to run your tests with REST Assured. After it's added, you can start creating test cases.
Setting Up Basic Configuration for REST Assured
After adding the dependencies, it's time to configure REST Assured. REST Assured allows you to set a base URI, port, and other configurations globally, which will be applied across all your test cases. This can save you from repeatedly specifying the base URI in each test.
Here’s an example of how to configure the base URL in a test class:
.jpeg)
The base URI in this case is set to https://jsonplaceholder.typicode.com. You can utilize the dummy endpoints provided by this API for testing. You won't need to define this base URI again in each test because going forward, REST Assured will utilize it for all requests.
Writing a Simple "Hello World" API Test
With the setup complete, let’s move on to writing your first API test. In this example, we will use REST Assured to perform a GET request to an endpoint and validate the response. The goal is to check if the response contains the correct status code (200) and expected data (userId of 1).

This test verifies that the GET /posts/1 endpoint returns a status code of 200 and the userId is 1.
Key Features and Capabilities of REST Assured
- JSON and XML Parsing: Easily handle JSON and XML responses with built-in parsers. REST Assured can automatically deserialize JSON or XML responses into objects, making it simple to work with structured data. This feature helps in asserting nested values and complex data structures without requiring additional parsing libraries.
- Authentication Support: Works with OAuth2, Basic, and Digest authentication. REST Assured makes it easy to test secure APIs by allowing you to add authentication tokens or credentials to requests effortlessly. Dealing with token-based authentication or HTTP Basic Auth, REST Assured offers simple methods.
- File Uploads/Downloads: Provides methods to handle multipart file uploads and downloads. This feature allows you to test APIs that involve file handling, such as uploading images or documents to a server. You can also easily verify if the files are being downloaded correctly by the API and validate file integrity.
- Session and Cookies Handling: Enables management of sessions and cookies during API tests. This is useful when testing APIs that maintain user sessions or track user state through cookies. REST Assured allows you to capture, store, and reuse cookies or session IDs across multiple requests, enabling more realistic test scenarios.
Writing Your First API Test with REST Assured
When creating API tests with REST Assured, the Given-When-Then format is key to writing clear, concise, and structured test cases. This pattern is inspired by Behaviour-Driven Development (BDD) and helps make test cases more readable and intuitive.
Understanding the Given-When-Then Syntax
REST Assured follows the Given-When-Then format for creating test cases:
- Given: This step defines the setup or preconditions of the request. This is where you specify things like request parameters, headers, authentication details, and other necessary information required before making the actual request.
- When: This step specifies the action you want to perform, such as the HTTP method you’re testing (GET, POST, PUT, DELETE). This is where the interaction with the API occurs.
- Then: This step defines the assertions you want to make in the response. It allows you to check things like status codes, headers, or the content of the response body to ensure the API behaves as expected.
Now, let's move on to examples of using these HTTP methods in REST Assured tests.
Making HTTP GET, POST, PUT, and DELETE Requests
REST Assured allows you to perform all major HTTP methods—GET, POST, PUT, DELETE—within the same testing framework. Here’s how each of these methods can be written and validated.
.jpeg)
In this example, we are making a GET request to the /posts/1 endpoint. This retrieves a resource, such as a post with ID 1, from the server. After performing the request, we validate that the API responds with a status code of 200 (OK), confirming that the request was successful and the resource exists.
.jpeg)
Here, we’re making a POST request to create a new resource on the server. The body() method is used to define the data being sent in the request, in this case, a JSON object containing title, body, and userId. The expected status code is 201, which indicates that the resource was successfully created.
.jpeg)
This example demonstrates a PUT request, which is used to update an existing resource on the server. We are sending the updated data for post 1 via the body() method. The status code 200 indicates that the update was successful.
.jpeg)
Finally, the DELETE request is used to remove a resource from the server. In this case, we are deleting the post with ID 1. After the request, we validate that the status code is 200, indicating that the resource was successfully deleted.
Adding Assertions for Status Codes and Response Data
Assertions allow you to verify various aspects of the response, such as status codes, headers, or the content of the body. Here’s how you can add multiple assertions to your test:
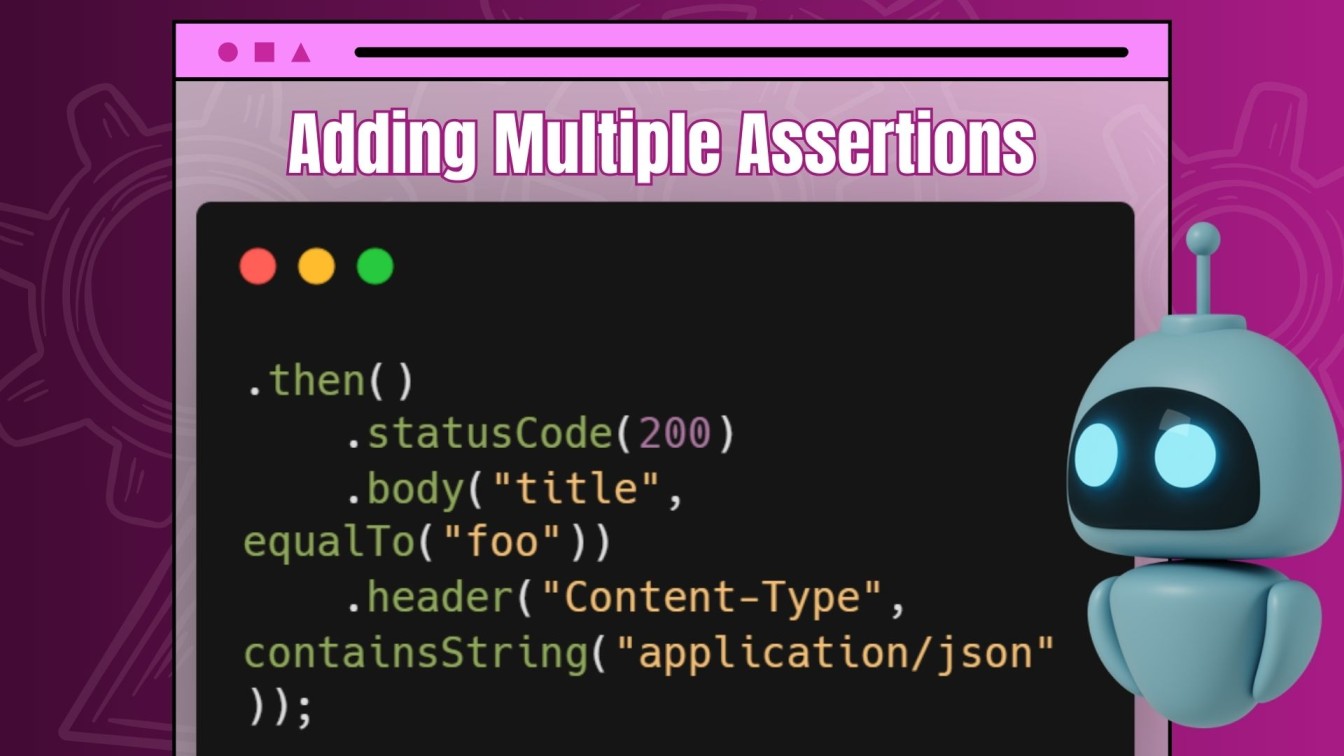
In this snippet:
- We are first asserting that the status code is 200, indicating a successful request.
- Then, we check that the response body contains a field called "title" with the value "foo". This ensures that the API returns the expected data.
- Finally, we verify that the Content-Type header contains "application/json", confirming that the response is in JSON format.
These assertions help you ensure API behaves as expected and returns correct data, headers, and status codes.
Common Challenges Faced When Using REST Assured and How to Overcome Them
- Dealing with Dynamic Data: Use variables and parameterization to handle data-driven tests. When APIs return dynamic data, such as session IDs or timestamps, you can store those values in variables and reuse them in subsequent requests or validations. Additionally, using a data-driven approach allows you to test the API with multiple sets of data efficiently, enhancing test coverage.
- Handling Authentication: Ensure correct tokens or session handling by configuring authentication methods. REST Assured supports various authentication types such as OAuth2, Basic, and Digest, making it easier to access secure APIs. For token-based authentication, you can capture the token from a login API and pass it to subsequent requests, ensuring your tests maintain the correct session state.
- Timeouts and Delays: Set timeouts for slow APIs to prevent tests from hanging. In real-world scenarios, some APIs may take longer to respond due to server load or complex operations. REST Assured allows you to configure request timeouts, which helps avoid long-running or stuck tests by setting an upper limit for the response time. This ensures that your test suite remains efficient and reliable.

Best Practices for Using REST Assured in API Testing
By following these best practices, your REST Assured test suite becomes more maintainable and easy to update as APIs evolve. Modular design enhances reusability, reducing duplication across tests. Reliable tests in CI/CD pipelines provide quick feedback, preventing critical bugs.
Parameterization and version control keep tests adaptable to data and API changes. Finally, including negative tests, logging, and performance checks ensures your suite is robust, catching performance and security issues.

Integrating REST Assured with Other Testing Tools
REST Assured can be easily integrated with a variety of other tools to enhance its functionality, from test management to reporting and continuous integration. This makes it highly versatile for different stages of API testing and automation, allowing testers to streamline workflows and improve collaboration. Below are some common integrations used alongside REST Assured.
Using REST Assured with JUnit or TestNG
REST Assured integrates seamlessly with JUnit or TestNG, two popular testing frameworks used in Java projects. By combining REST Assured with JUnit or TestNG, you can take advantage of annotations like @Test, making your tests more organized and easier to execute in larger test suites. This integration also enables easy test case management, allowing you to group, run, and report test cases more efficiently.

Combining REST Assured with Allure for Test Reporting
REST Assured integration with Allure allows you to generate rich, interactive reports that visualize test results in an easy-to-read format. By incorporating Allure, you gain insights into test execution, such as pass/fail statuses, detailed error logs, and metrics like response times. This helps testers and developers track issues more effectively and communicate test results with stakeholders.
- To integrate Allure, you need to configure your project with Allure dependencies and ensure that test results are captured during execution.
- The combination of REST Assured with Allure enhances the visibility of your API testing efforts and ensures that your test outcomes are documented.
Integrating with Jenkins for CI/CD
Integrating REST Assured with Jenkins enables automated API testing as part of your continuous integration/continuous delivery (CI/CD) pipeline. With Jenkins, you can set up jobs that trigger your REST Assured test suite automatically whenever new changes are pushed to your codebase. This ensures that APIs are tested regularly, providing immediate feedback on their functionality after every build or deployment.
- By running REST Assured tests in Jenkins, you ensure that your APIs are continuously validated for correctness, performance, and reliability, reducing the risk of releasing faulty APIs into production.
- Jenkins also supports detailed reporting, making it easier to track test results and identify any issues that need to be addressed.
Comparing REST Assured with Other API Testing Tools
Before diving into REST Assured capabilities, let's compare it with some of the other popular API testing tools to understand its strengths and limitations.
REST Assured for Automation: Writing Reusable Test Scripts
One of the key advantages of using REST Assured in API testing is the ability to create reusable test scripts. Writing reusable scripts not only enhances test maintainability but also significantly reduces duplication and effort when dealing with multiple test cases. Here’s how you can achieve this by leveraging methods, classes, and data-driven approaches:

Advanced Techniques in REST Assured for API Testing
- Handling File Uploads/Downloads: REST Assured makes it easy to handle file uploads using multipart form data, which is essential for testing APIs that handle file operations. You can use the multiPart() method to upload files and validate their successful transmission. Similarly, for file downloads, REST Assured allows you to verify the file contents and ensure the correct file types and sizes are being returned by the API.
- Complex JSON/XML Validation: REST Assured provides powerful methods to validate complex, nested JSON and XML structures in API responses. Using jsonPath() and xmlPath(), you can easily extract and assert specific fields, even when the data is deeply nested. This makes it convenient to work with large, hierarchical responses, ensuring that all key elements are accurately tested for correctness.
- Parameterized Tests: By using @Parameterized annotations in frameworks like JUnit or TestNG, you can run the same REST-assured test with multiple input values. This approach is useful for validating how an API handles different data sets, ensuring comprehensive coverage without duplicating test logic. Parameterized tests streamline your suite, allowing you to verify multiple scenarios efficiently.
Rounding Out!
In conclusion, REST Assured is a powerful API testing tool that simplifies writing and maintaining API test scripts. For a small project or large-scale automation, REST Assured provides the flexibility and robustness you need to ensure your APIs are functioning as expected. 🎯
People also asked
👉 What are the prerequisites for using REST Assured?
The prerequisites for using REST Assured include a basic understanding of Java, Maven or Gradle for dependency management, and familiarity with HTTP methods and API testing concepts.
👉 How do I set up REST Assured in a Java project?
In your build.gradle (Gradle) or pom.xml (Maven) file, add the REST Assured dependency, and import the required classes.
👉How do I send a GET request using REST Assured?
Insert given().when() to use.To send a GET request with REST Assured, use the get("/endpoint") method.
👉 How can I validate the response of an API request in REST Assured?
The then() method can be used to assert status codes, headers, and body content in responses.
👉 Can REST Assured be used for testing both JSON and XML responses?
Yes, both JSON and XML replies can be parsed and validated using REST Assured.